【LeetCode】290. Word Pattern 單詞規律(Easy)(JAVA)每日一題
【LeetCode】290. Word Pattern 單詞規律(Easy)(JAVA)
題目地址: https://leetcode.com/problems/word-pattern/
題目描述:
Given a pattern and a string s, find if s follows the same pattern.
Here follow means a full match, such that there is a bijection between a letter in pattern and a non-empty word in s.
Example 1:
Input: pattern = "abba", s = "dog cat cat dog"
Output: true
Example 2:
Input: pattern = "abba", s = "dog cat cat fish"
Output: false
Example 3:
Input: pattern = "aaaa", s = "dog cat cat dog"
Output: false
Example 4:
Input: pattern = "abba", s = "dog dog dog dog"
Output: false
Constraints:
- 1 <= pattern.length <= 300
- pattern contains only lower-case English letters.
- 1 <= s.length <= 3000
- s contains only lower-case English letters and spaces ’ '.
- s does not contain any leading or trailing spaces.
- All the words in s are separated by a single space.
題目大意
給定一種規律 pattern 和一個字串 str ,判斷 str 是否遵循相同的規律。
這裡的 遵循 指完全匹配,例如, pattern 裡的每個字母和字串 str 中的每個非空單詞之間存在著雙向連線的對應規律
說明:
你可以假設 pattern 只包含小寫字母, str 包含了由單個空格分隔的小寫字母。
解題方法
- 要字元和字串一一對應,不能是多對一也不能是一對多
- 把對應關係記錄下來,然後每次檢視是否相同即可
- note: 要考慮長度不一的問題
class Solution {
public boolean wordPattern(String pattern, String s) {
Map<Character, String> map = new HashMap<>();
Set<String> set = new HashSet<>();
int index = 0;
for (int i = 0; i < pattern.length(); i++) {
char ch = pattern.charAt(i);
int start = index;
if (index >= s.length()) return false;
while (index < s.length() && s.charAt(index) != ' ') {
index++;
}
String cur = s.substring(start, index);
index++;
String pre = map.get(ch);
if (pre == null) {
if (set.contains(cur)) return false;
set.add(cur);
map.put(ch, cur);
} else {
if (!pre.equals(cur)) return false;
}
}
return index >= s.length();
}
}
執行耗時:1 ms,擊敗了98.94% 的Java使用者
記憶體消耗:36.4 MB,擊敗了80.89% 的Java使用者
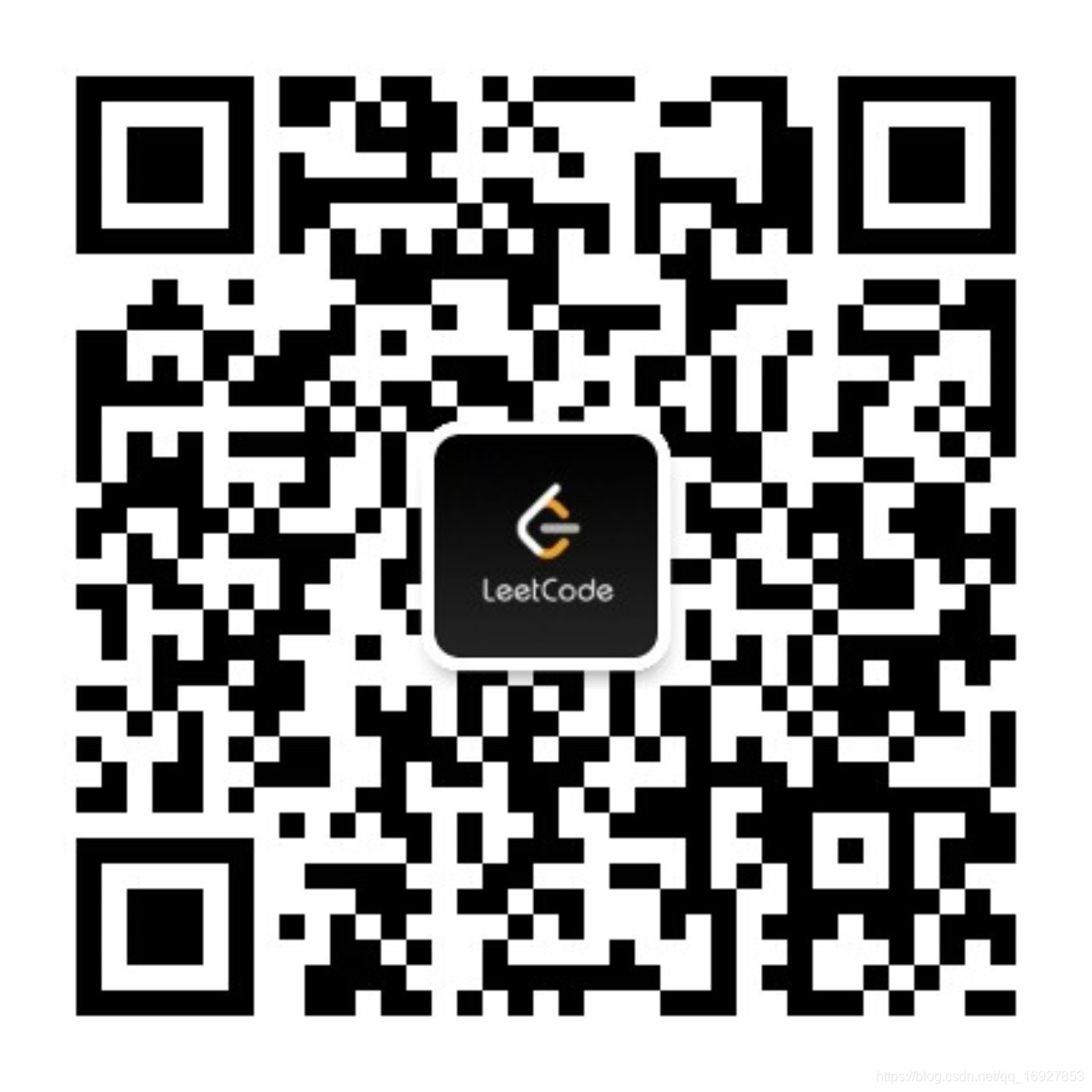
相關文章
- LeetCode-單詞規律LeetCode
- LeetCode-290-單詞規律LeetCode
- leedcode-單詞規律
- leetcode【每日一題】242. 有效的字母異位詞 javaLeetCode每日一題Java
- LeetCode每日一題: 最後一個單詞的長度(No.58)LeetCode每日一題
- LeetCode每日一題:反轉字串中的單詞 III(No.557)LeetCode每日一題字串
- 290.單詞模式。給定一種 pattern(模式) 和一個字串 str ,判斷 str 是否遵循相同的模式。(c++方法)模式字串C++
- leetcode每日一題LeetCode每日一題
- leetcode演算法題解(Java版)-16-動態規劃(單詞包含問題)LeetCode演算法Java動態規劃
- LeetCode每日一題:唯一摩爾斯密碼詞(No.804)LeetCode每日一題密碼
- LeetCode:每日一題:27. 移除元素 ——————簡單LeetCode每日一題
- Leetcode每日一題(1)LeetCode每日一題
- j-easy/easy-rules: Java簡單的規則引擎Java
- leetcode刷題之1160拼寫單詞 java題解(超詳細)LeetCodeJava
- java 英文單詞拼寫糾正框架(Word Checker)Java框架
- LeetCode 2024/6 每日一題 合集LeetCode每日一題
- [LeetCode題解]79. 單詞搜尋LeetCode
- LeetCode 單詞拆分LeetCode
- 【leetcode 簡單】第十四題 最後一個單詞的長度LeetCode
- LeetCode 每日一題「判定字元是否唯一」LeetCode每日一題字元
- Java規則引擎 Easy RulesJava
- LeetCode每日一題: 找不同(No.389)LeetCode每日一題
- LeetCode每日一題: 移除元素(No.27)LeetCode每日一題
- LeetCode 252. Meeting Rooms (Java版; Easy)LeetCodeOOMJava
- JAVA每日一題20201109Java每日一題
- 【LeetCode】455. Assign Cookies 分發餅乾(Medium)(JAVA)每日一題LeetCodeCookieJava每日一題
- LeetCode1160.拼寫單詞(Java+暴力+HashMap)LeetCodeJavaHashMap
- 每日一練(31):翻轉單詞順序
- 每日一詞-normORM
- 每日一詞-factor in
- 每日一詞 sidetrackIDE
- 每日一詞-remainREMAI
- Leetcode(easy heap)LeetCode
- LeetCode-EasyLeetCode
- 75. Sort Colors(Leetcode每日一題-2020.10.07)LeetCode每日一題
- LeetCode每日一題: 排列硬幣(No.441)LeetCode每日一題
- LeetCode每日一題: 各位相加(No.258)LeetCode每日一題
- 【LeetCode】每日一題164. 最大間距LeetCode每日一題