from PyQt5.QtWidgets import *
import pyqtgraph as pg
import sys
class MainWindow(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle('pyqtgraph作圖示例')
# 建立 GraphicsLayoutWidget 物件
self.pw = pg.GraphicsLayoutWidget()
self.pw.setBackground('w')
# 繪圖物件1
plot1 = self.pw.addPlot()
plot1.setTitle("訂單數量1", color='#008080', size='12pt')
x1 = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
y1 = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
bg1 = pg.BarGraphItem(x=x1, height=y1, width=0.3, brush='r')
# 新增到介面上
plot1.addItem(bg1)
# 繪圖物件2
plot2 = self.pw.addPlot()
plot2.setTitle("訂單數量2", color='#008080', size='12pt')
x2 = [0.33, 1.33, 2.33, 3.33, 4.33, 5.33, 6.33, 7.33, 8.33, 9.33]
y2 = [0.33, 1.33, 2.33, 3.33, 4.33, 5.33, 6.33, 7.33, 8.33, 9.33]
bg2 = pg.BarGraphItem(x=x2, height=y2, width=0.3, brush='g')
# 新增到介面上
plot2.addItem(bg2)
# 建立其他Qt控制元件
okButton = QPushButton("OK")
lineEdit = QLineEdit('點選資訊')
# 水平layout裡面放 edit 和 button
hbox = QHBoxLayout()
hbox.addWidget(lineEdit)
hbox.addWidget(okButton)
# 垂直layout裡面放 pyqtgraph圖表控制元件 和 前面的水平layout
vbox = QVBoxLayout()
vbox.addWidget(self.pw)
vbox.addLayout(hbox)
# 設定全域性layout
self.setLayout(vbox)
if __name__ == '__main__':
app = QApplication(sys.argv)
main = MainWindow()
main.show()
app.exec_()
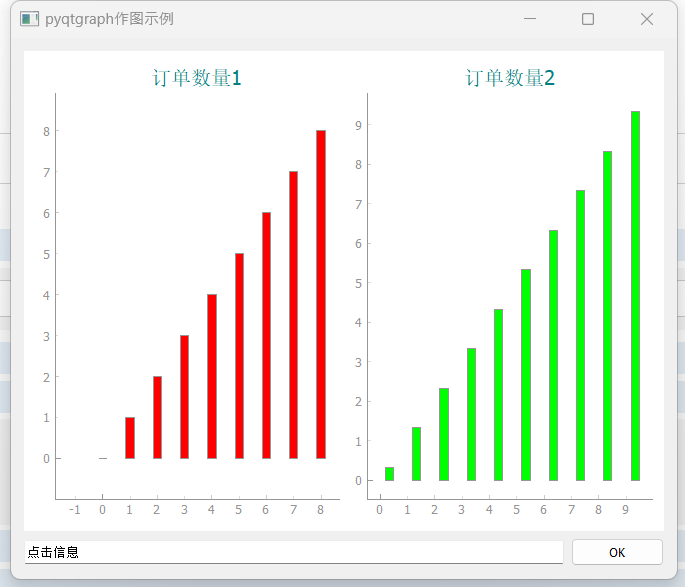