【Python】基於pymysql的資料庫操作類
一 簡介
Python和MySQL互動的模組有 MySQLdb 和 PyMySQL(pymysql),MySQLdb是基於C 語言編寫的,而且Python3 不在支援MySQLdb 。PyMySQL是一個純Python寫的MySQL客戶端,它的目標是替代MySQLdb,可以在CPython、PyPy、IronPython和Jython環境下執行,PyMySQL在MIT許可下發布。
在開發基於Python語言的專案中,為了以後系統能相容Python3,我們使用了PyMySQL替換了MySQLdb。下面我們來熟悉一下pymysql的使用。
二 安裝方式
pymsql的原始碼 ,目前還在持續更新。
, 強烈建議大家仔細閱讀一遍。其用法和MySQLdb相差無幾,核心用法一致。這樣使用pymysql替換mysqldb的成本極小。
三 基於pymysql的資料庫互動
四 小結
前幾天剛剛將我們的系統中的MySQLdb 替換為PyMySQL, 還未遇到問題。歡迎大家使用測試上述指令碼,有問題歡迎和我討論。如果本文對您有幫助 ,可以贊助 一瓶飲料。
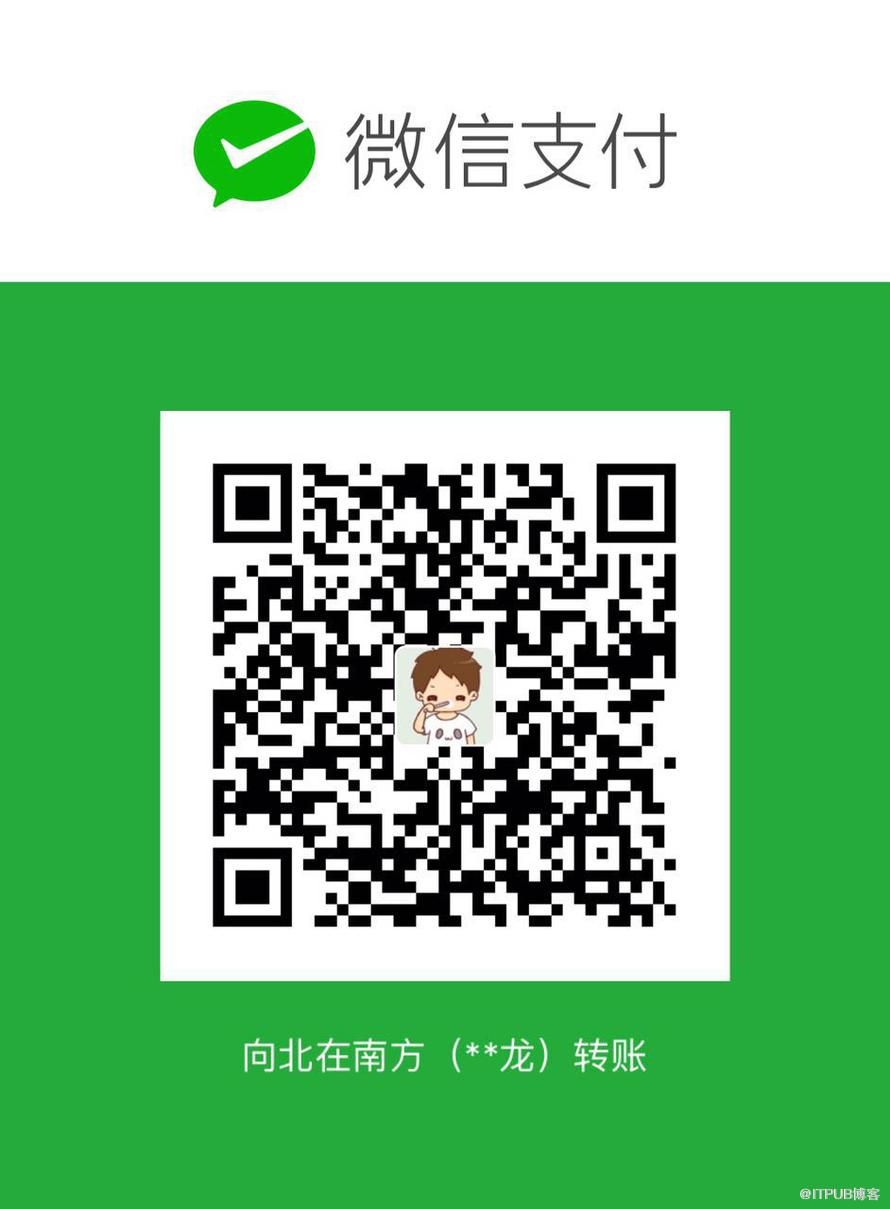
Python和MySQL互動的模組有 MySQLdb 和 PyMySQL(pymysql),MySQLdb是基於C 語言編寫的,而且Python3 不在支援MySQLdb 。PyMySQL是一個純Python寫的MySQL客戶端,它的目標是替代MySQLdb,可以在CPython、PyPy、IronPython和Jython環境下執行,PyMySQL在MIT許可下發布。
在開發基於Python語言的專案中,為了以後系統能相容Python3,我們使用了PyMySQL替換了MySQLdb。下面我們來熟悉一下pymysql的使用。
二 安裝方式
pymsql的原始碼 ,目前還在持續更新。
-
安裝要求:
-
Python -- one of the following:
-
CPython >= 2.6 or >= 3.3
-
PyPy >= 4.0
-
IronPython 2.7
-
MySQL Server -- one of the following:
-
MySQL >= 4.1 (tested with only 5.5~)
- MariaDB >= 5.1
- 安裝
- pip install PyMySQL
三 基於pymysql的資料庫互動
-
#!/usr/bin/env python
-
# encoding: utf-8
-
"""
-
author: yangyi@youzan
-
time: 2015/6/8 上午11:34
-
func: 基於pymysql的資料庫互動類,支援事務提交和回滾,返回結果記錄行數,和insert的最新id
-
"""
-
import pymysql
-
from warnings import filterwarnings
-
filterwarnings('ignore', category=pymysql.Warning)
-
CONNECT_TIMEOUT = 100
-
IP = 'localhost'
-
PORT = 3306
-
USER = 'root'
-
PASSSWORD = ''
-
-
class QueryException(Exception):
-
"""
-
"""
-
-
-
class ConnectionException(Exception):
-
"""
- """
-
-
class MySQL_Utils():
-
def __init__(
-
self, ip=IP, port=PORT, user=USER, password=PASSSWORD,
-
connect_timeout=CONNECT_TIMEOUT, remote=False, socket='', dbname='test'):
-
self.__conn = None
-
self.__cursor = None
-
self.lastrowid = None
-
self.connect_timeout = connect_timeout
-
self.ip = ip
-
self.port = port
-
self.user = user
-
self.password = password
-
self.mysocket = socket
-
self.remote = remote
-
self.db = dbname
-
self.rows_affected = 0
-
-
-
def __init_conn(self):
- try:
-
conn = pymysql.connect(
-
host=self.ip,
-
port=int(self.port),
-
user=self.user,
-
db=self.db,
-
connect_timeout=self.connect_timeout,
-
charset='utf8', unix_socket=self.mysocket)
-
except pymysql.Error as e:
-
raise ConnectionException(e)
-
self.__conn = conn
-
-
-
def __init_cursor(self):
-
if self.__conn:
-
self.__cursor = self.__conn.cursor(pymysql.cursors.DictCursor)
-
-
-
def close(self):
-
if self.__conn:
-
self.__conn.close()
-
self.__conn = None
-
- #專門處理select 語句
-
def exec_sql(self, sql, args=None):
-
try:
-
if self.__conn is None:
-
self.__init_conn()
-
self.__init_cursor()
-
self.__conn.autocommit = True
-
self.__cursor.execute(sql, args)
-
self.rows_affected = self.__cursor.rowcount
-
results = self.__cursor.fetchall()
-
return results
-
except pymysql.Error as e:
-
raise pymysql.Error(e)
-
finally:
-
if self.__conn:
-
self.close()
-
- # 專門處理dml語句 delete,updete,insert
-
def exec_txsql(self, sql, args=None):
-
try:
-
if self.__conn is None:
-
self.__init_conn()
-
self.__init_cursor()
-
if self.__cursor is None:
-
self.__init_cursor()
-
-
- self.rows_affected=self.__cursor.execute(sql, args)
- self.lastrowid = self.__cursor.lastrowid
- return self.rows_affected
-
except pymysql.Error as e:
-
raise pymysql.Error(e)
-
finally:
-
if self.__cursor:
-
self.__cursor.close()
-
self.__cursor = None
-
- # 提交
-
def commit(self):
-
try:
-
if self.__conn:
-
self.__conn.commit()
-
except pymysql.Error as e:
-
raise pymysql.Error(e)
-
finally:
-
if self.__conn:
-
self.close()
-
- #回滾操作
-
def rollback(self):
-
try:
-
if self.__conn:
- self.__conn.rollback()
-
except pymysql.Error as e:
-
raise pymysql.Error(e)
-
finally:
-
if self.__conn:
-
self.close()
-
# 適用於需要獲取插入記錄的主鍵自增id
-
def get_lastrowid(self):
- return self.lastrowid
#獲取dml操作影響的行數
def get_affectrows(self):
return self.rows_affected
#MySQL_Utils初始化的例項銷燬之後,自動提交
def __del__(self):
self.commit()
def get_affectrows(self):
return self.rows_affected
#MySQL_Utils初始化的例項銷燬之後,自動提交
def __del__(self):
self.commit()
前幾天剛剛將我們的系統中的MySQLdb 替換為PyMySQL, 還未遇到問題。歡迎大家使用測試上述指令碼,有問題歡迎和我討論。如果本文對您有幫助 ,可以贊助 一瓶飲料。
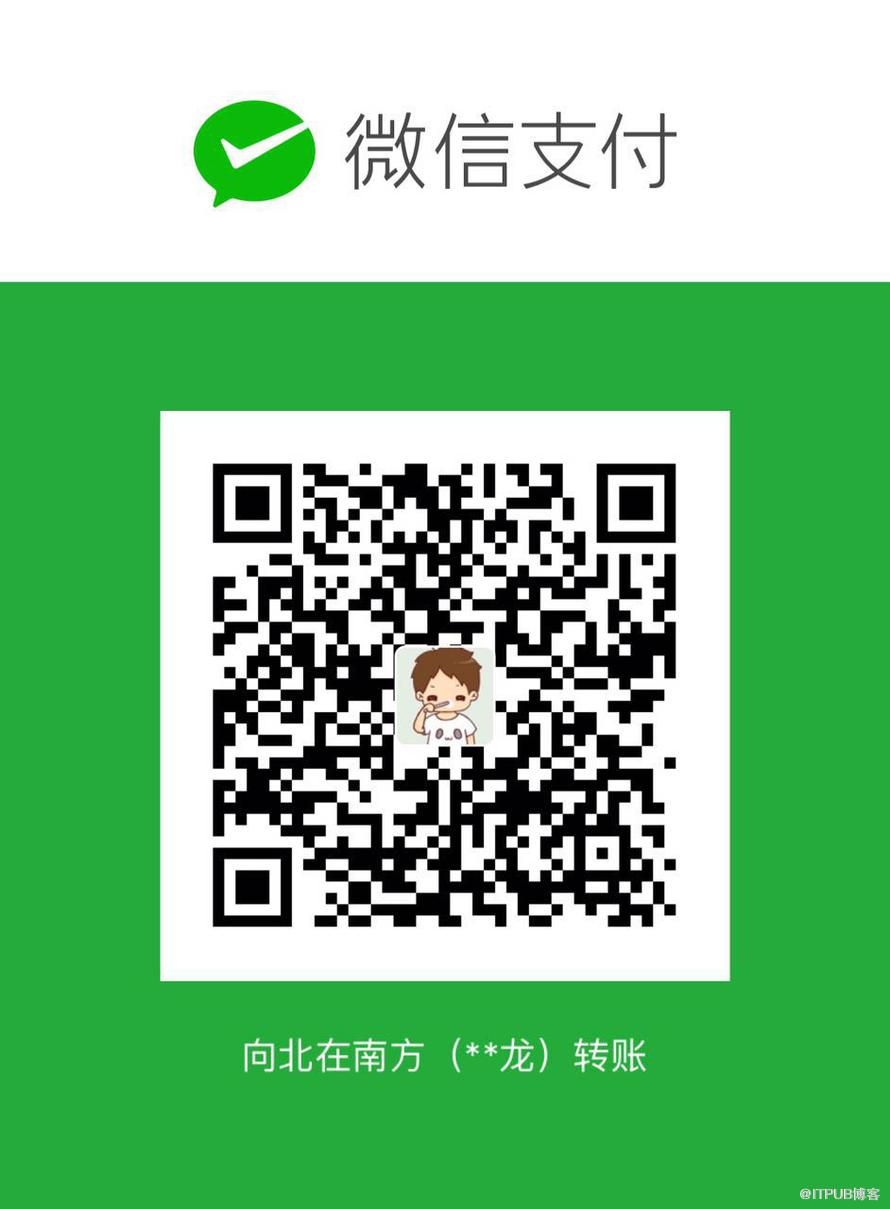
來自 “ ITPUB部落格 ” ,連結:http://blog.itpub.net/22664653/viewspace-2140569/,如需轉載,請註明出處,否則將追究法律責任。
相關文章
- python資料庫操作 - PyMySQL入門Python資料庫MySql
- python操作MySQL資料庫連線(pymysql)PythonMySql資料庫
- Python3結構化資料庫操作包pymysqlPython資料庫MySql
- Python 利用pymysql和openpyxl操作MySQL資料庫並插入Excel資料PythonMySql資料庫Excel
- SQLite Helper類,基於.net c#的SQLite資料庫操作類SQLiteC#資料庫
- (資料庫之pymysql)資料庫MySql
- .NET關於資料庫操作的類-囊括所有的操作資料庫
- Python3資料庫操作基本類Python資料庫
- python3使用PyMysql連線mysql資料庫PythonMySql資料庫
- PHP常用操作類實現——資料庫操作類PHP資料庫
- 基於DataTables的資料操作demo
- 基於Python的介面自動化實戰-基礎篇之pymysql模組運算元據庫PythonMySql
- Django使用pymysql連線MySQL資料庫DjangoMySql資料庫
- Python使用pymysql和xlrd2將Excel資料匯入MySQL資料庫PythonMySqlExcel資料庫
- 【侯壘 】SQL資料庫操作類SQL資料庫
- php簡單操作mysql資料庫的類PHPMySql資料庫
- C#的Access資料庫操作 AccessHelper類C#資料庫
- Python操作SQLite資料庫PythonSQLite資料庫
- Python 操作 SQLite 資料庫PythonSQLite資料庫
- python資料庫(mysql)操作Python資料庫MySql
- python 操作mysql資料庫PythonMySql資料庫
- Python Mysql 資料庫操作PythonMySql資料庫
- python操作mysql資料庫PythonMySql資料庫
- python操作mongodb資料庫PythonMongoDB資料庫
- MongoDB資料庫基礎操作MongoDB資料庫
- 資料庫——基礎(資料庫操作,表格操作)——增加高階查詢資料庫
- 資料庫開發(19)基於物件的資料庫資料庫物件
- [python] 基於Tablib庫處理表格資料Python
- ASP.NET MongoDB資料庫操作類ASP.NETMongoDB資料庫
- C#:資料庫SQL操作通用類C#資料庫SQL
- python+資料庫(三)用python對資料庫基本操作Python資料庫
- Python操作MongoDB文件資料庫PythonMongoDB資料庫
- Python 資料庫騷操作 — RedisPython資料庫Redis
- Python資料庫MongoDB騷操作Python資料庫MongoDB
- Python 資料庫騷操作 -- RedisPython資料庫Redis
- Python之 操作 MySQL 資料庫PythonMySql資料庫
- Python 資料庫騷操作 -- MongoDBPython資料庫MongoDB
- Mysql資料庫基礎操作命令MySql資料庫