順序棧的實現方式
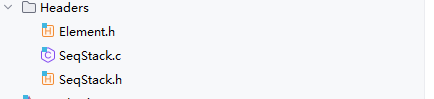
Element.h
typedef struct {
int id;
char * name;
} ElementType;
SeqStack.h
#include <stdio.h>
#include "Element.h"
#include <stdbool.h>
/** 定義棧的順序儲存方式*/
//const int maxsize = 6; //順序棧的容量
typedef struct Seqstack {
ElementType elements[MAXSIZE]; //順序棧中存放資料元素的陣列
int top; //棧頂下標
int length; //當前棧的元素個數
} SeqStk;
//初始化棧
void InitSeqStack(SeqStk *stk);
//判斷棧是否為空
bool EmptyStack(SeqStk *stk);
//進棧
bool PushSeqStack(SeqStk *stk, ElementType element);
//出棧
bool PopSeqStack(SeqStk *stk, ElementType *element);
//獲取棧頂元素
ElementType GetTop(SeqStk *stk);
//清空棧
void ClearSeqStack(SeqStk *stk);
SeqStack.c
#include <stdio.h>
#include "SeqStack.h"
#include <stdbool.h>
//初始化棧
void InitSeqStack(SeqStk *stk) {
stk->top = -1;
stk->length = 0;
}
//判斷棧是否為空
bool EmptyStack(SeqStk *stk) {
if (stk->top == -1)
return true;
return false;
}
//進棧
bool PushSeqStack(SeqStk *stk, ElementType element) {
//判斷棧是否滿
if (stk->top == MAXSIZE - 1) {
printf("棧已滿,進棧失敗!");
return false;
}
stk->top++; //棧未滿,棧頂指標+1
stk->elements[stk->top] = element; //新插入元素賦值給棧頂
stk->length++;
return true;
}
//出棧
bool PopSeqStack(SeqStk *stk, ElementType *element) {
//判斷棧是否空
if (EmptyStack(stk)) {
printf("空棧,出棧失敗!");
return false;
}
*element = stk->elements[stk->top]; //獲取棧頂元素
stk->top--; //棧頂top值-1
stk->length--;
return true;
}
//獲取棧頂元素
ElementType GetTop(SeqStk *stk) {
if (EmptyStack((stk)) == 1) {
printf("空棧,棧頂元素為空!");
}
return stk->elements[stk->top]; //返回棧頂資料元素
}
//清空棧
void ClearSeqStack(SeqStk *stk) {
stk->top = -1;
stk->length = 0;
}
main.c
#include <stdio.h>
#include <stdlib.h>
#include "Headers/Element.h"
#include "Headers/SeqStack.h"
ElementType datas[] = {
{1, "青花瓷"},
{2, "東風破"},
{3, "發如雪"},
{4, "娘子"},
{5, "菊花臺"},
{6, "千里之外"},
};
void TestSeqStack() {
SeqStk *stk = (SeqStk *)malloc(sizeof(SeqStk));
ElementType *element = (ElementType *)malloc(sizeof(ElementType));
InitSeqStack(stk);
for (int i=0; i<6; i++) {
printf("當前入棧元素:%d\t%s\n",datas[i].id,datas[i].name);
PushSeqStack(stk,datas[i]);
}
PopSeqStack(stk,element);
printf("當前出棧元素:%d\t%s\n",element->id,element->name);
for (int i=0; i<stk->length; i++) {
printf("當前棧內元素:%d\t%s\n",stk->elements[i].id,stk->elements[i].name);
}
printf("清除棧!");
ClearSeqStack(stk);
PopSeqStack(stk,element);
free(stk);
}
int main(void) {
TestSeqStack();
return 0;
}