點選檢視程式碼
import numpy as np
from scipy.optimize import minimize
def objective(x):
return -np.sum(np.sqrt(x) * np.arange(1, 101))
def constraint1(x):
return x[1] - 10
def constraint2(x):
return 20 - (x[1] + 2*x[2])
def constraint3(x):
return 30 - (x[1] + 2*x[2] + 3*x[3])
def constraint4(x):
return 40 - (x[1] + 2*x[2] + 3*x[3] + 4*x[4])
def constraint5(x):
return 1000 - np.dot(x, np.arange(1, 101))
constraints = [
{'type': 'ineq', 'fun': constraint1},
{'type': 'ineq', 'fun': constraint2},
{'type': 'ineq', 'fun': constraint3},
{'type': 'ineq', 'fun': constraint4},
{'type': 'ineq', 'fun': constraint5}
]
bounds = [(0, None)] * 100
x0 = np.ones(100) * 0.1
result = minimize(objective, x0, method='SLSQP', constraints=constraints, bounds=bounds)
print('Optimal solution:', result.x)
print('Objective function value at optimal solution:', -result.fun)
print("學號:3004")
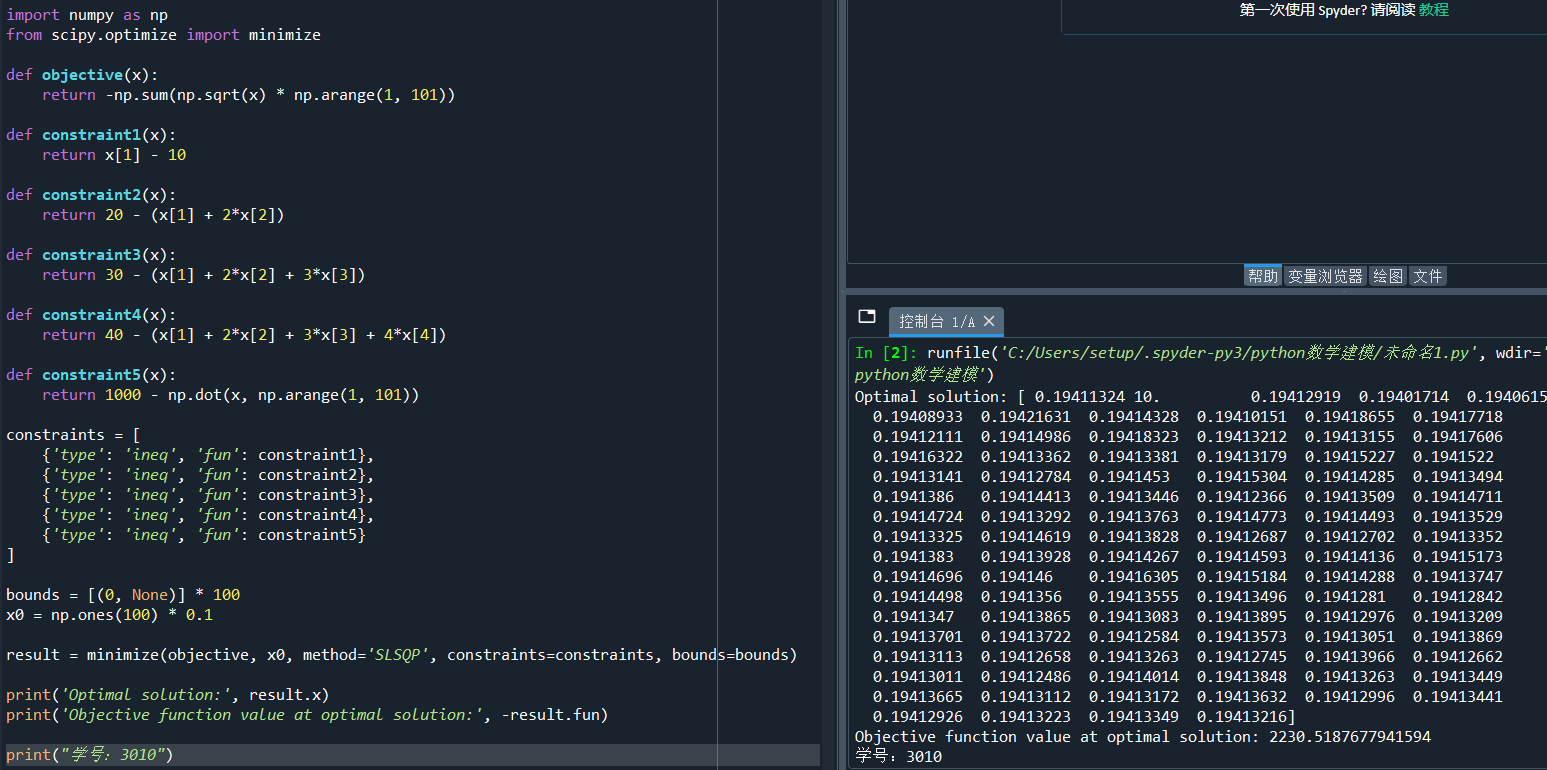
點選檢視程式碼
import numpy as np
from scipy.optimize import minimize
def objective(x):
return 2*x[0] + 3*x[0]**2 + 3*x[1] + x[1]**2 + x[2]
def constraint1(x):
return 10 - (x[0] + 2*x[0]**2 + x[1] + 2*x[1]**2 + x[2])
def constraint2(x):
return 50 - (x[0] + x[0]**2 + x[1] + x[1]**2 - x[2])
def constraint3(x):
return 40 - (2*x[0] + x[0]**2 + 2*x[1] + x[2])
def constraint4(x):
return x[0]**2 + x[2] - 2
def constraint5(x):
return 1 - (x[0] + 2*x[1])
constraints = [
{'type': 'ineq', 'fun': constraint1},
{'type': 'ineq', 'fun': constraint2},
{'type': 'ineq', 'fun': constraint3},
# {'type': 'eq', 'fun': constraint4},
{'type': 'ineq', 'fun': constraint5}
]
bounds = [(0, None)] * 3
x0 = np.array([0.1, 0.1, 0.1])
result = minimize(objective, x0, method='SLSQP', constraints=constraints, bounds=bounds)
print('Optimal solution:', result.x)
print('Objective function value at optimal solution:', result.fun)
print("學號:3010")
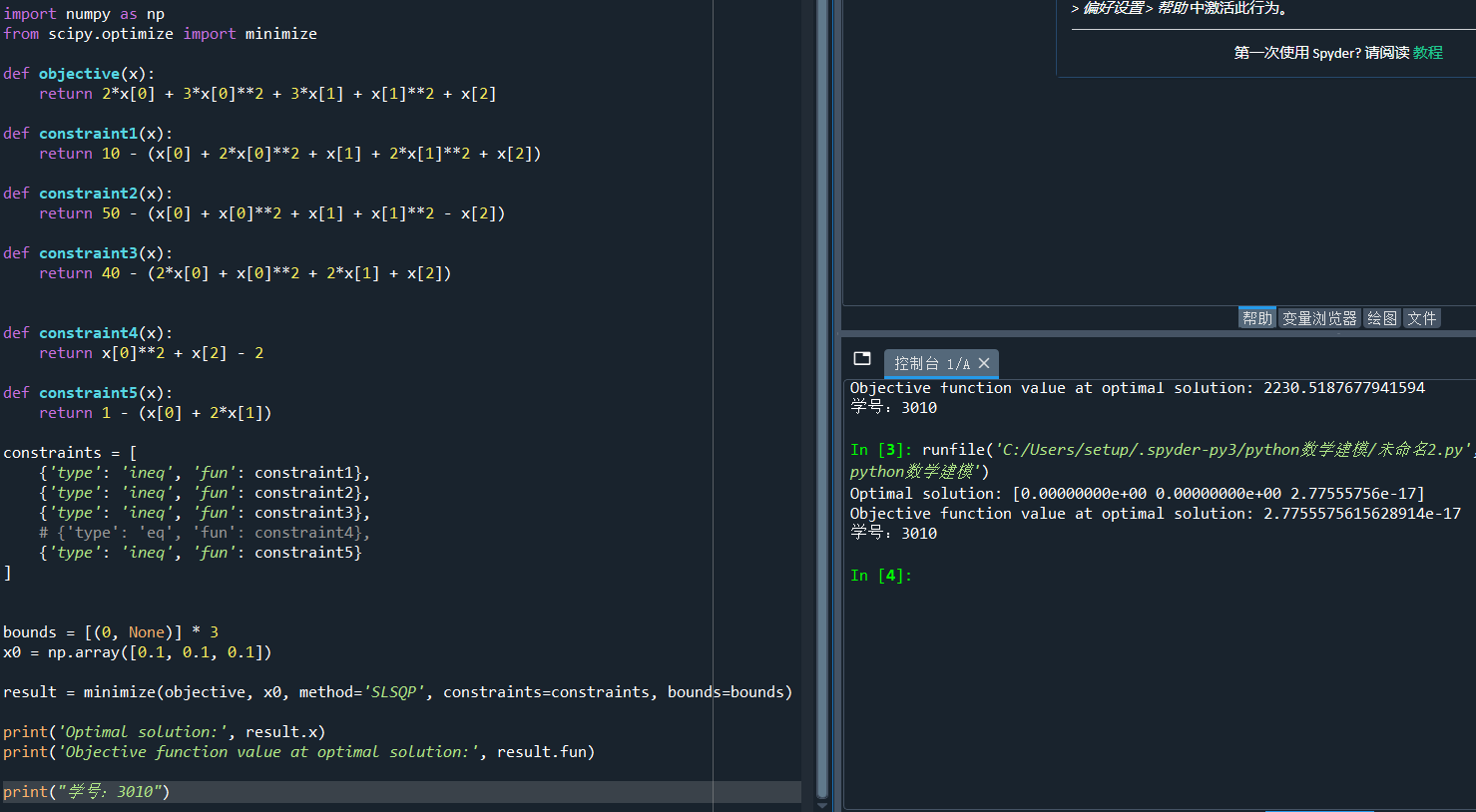