模板PDF 的欄位
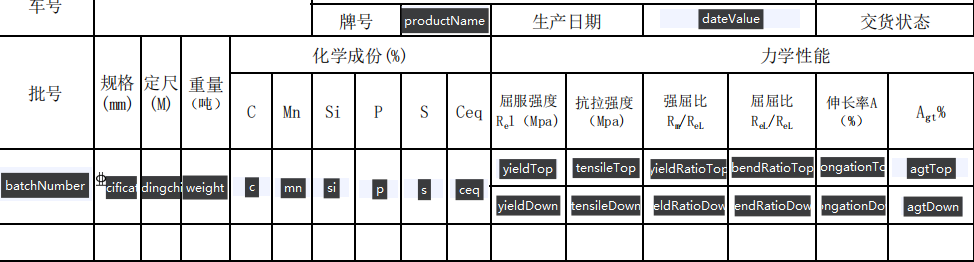
引入maven
<!-- iText for generating PDF files -->
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.5.13.2</version>
</dependency>
Java 程式碼
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.Image;
import com.itextpdf.text.pdf.*;
/**
* 根據pdf模板進行質檢報告生成 並上傳oss地址 生成二維碼在質檢報告右上角
* @param recordDTO
* @return 返回 oss url
*/
private String getPdfFilePath(TagPrintingRecordVO recordDTO) {
String fileName = "";
PdfReader reader;
ByteArrayOutputStream bos = new ByteArrayOutputStream();
try {
reader = new PdfReader("pdf/template.pdf");
bos = new ByteArrayOutputStream();
PdfStamper stamper = new PdfStamper(reader, bos);
AcroFields form = stamper.getAcroFields();
Map<String, String> map = new HashMap<>();
map.put("productName", recordDTO.getProductName());
map.put("weight", "");
map.put("specification", recordDTO.getSpecification().toString());
if(recordDTO.getType() == 1){ // 盤螺
map.put("dingchi", "");
map.put("deliveryType", "盤螺");
}else{ // 螺紋
map.put("dingchi", recordDTO.getDingchi().toString());
map.put("deliveryType", "熱軋");
}
map.put("batchNumber", recordDTO.getBatchNumber());
map.put("c", recordDTO.getC().toString());
map.put("mn", recordDTO.getMn().toString());
map.put("si", recordDTO.getSi().toString());
map.put("p", recordDTO.getP().toString());
map.put("s", recordDTO.getS().toString());
map.put("ceq", recordDTO.getCeq().toString());
map.put("yieldTop", recordDTO.getYieldTop());
map.put("yieldDown", recordDTO.getYieldDown().toString());
map.put("tensileTop", recordDTO.getTensileTop());
map.put("tensileDown", recordDTO.getTensileDown().toString());
map.put("yieldRatioTop", recordDTO.getYieldRatioTop());
map.put("yieldRatioDown", recordDTO.getYieldRatioDown().toString());
map.put("bendRatioTop", recordDTO.getBendRatioTop());
map.put("bendRatioDown", recordDTO.getBendRatioDown().toString());
map.put("elongationTop", recordDTO.getElongationTop());
map.put("elongationDown", recordDTO.getElongationDown().toString());
map.put("agtTop", recordDTO.getAgtTop());
map.put("agtDown", recordDTO.getAgtDown().toString());
map.put("gapTop", recordDTO.getGapTop());
map.put("gapDown", recordDTO.getGapDown().toString());
map.put("inspector", recordDTO.getInspector());
map.put("dateValue", recordDTO.getDateValue());
String filechirldName = DateUtils.getNowTimeStamp().toString();
fileName = "pdf/" + filechirldName+ ".pdf";
File pdfFile = new File(fileName);
pdfFile.createNewFile();
FileOutputStream fileOutputStream = new FileOutputStream(pdfFile, false);
this.fillPdfCellForm(map, form);
// 獲取頁面大小
PdfReader pdfReader = new PdfReader("pdf/template.pdf");
PdfDictionary pageDict = pdfReader.getPageN(1);
PdfArray mediaBox = pageDict.getAsArray(PdfName.MEDIABOX);
float pageWidth = mediaBox.getAsNumber(2).floatValue();
float pageHeight = mediaBox.getAsNumber(3).floatValue();
// 生成二維碼
BarcodeQRCode qrCode = new BarcodeQRCode("https://XXXX.com/qrcode/"+filechirldName+".pdf", 200, 200, null);
Image qrCodeImage = qrCode.getImage();
qrCodeImage.scaleAbsolute(80, 80); // 設定二維碼圖片的大小
// 計算二維碼的位置(右上角)
float qrCodeX = pageWidth-80; // 80是右邊距
float qrCodeY = pageHeight-80; // 80是上邊距
qrCodeImage.setAbsolutePosition(qrCodeX, qrCodeY);
// 將二維碼圖片新增到 PDF 中
PdfContentByte contentByte = stamper.getOverContent(1);
contentByte.addImage(qrCodeImage);
stamper.setFormFlattening(true);
stamper.close();
reader.close();
fileOutputStream.write(bos.toByteArray());
fileOutputStream.close();
// 上傳 oss
Map<String, String> ossMap = AliYunOSSUtils.uploadFile(pdfFile, filechirldName+ ".pdf");
if(StringUtils.isEmpty(ossMap.get("url"))){
throw new RuntimeException("上傳oss 失敗");
}
pdfFile.delete();
return ossMap.get("url");
} catch (Exception e) {
e.printStackTrace();
throw new RuntimeException("建立PDF失敗");
}
}
/**
* 設定pdf form cell 設定字型
* @param map
* @param form
* @throws IOException
* @throws DocumentException
*/
private void fillPdfCellForm(Map<String, String> map, AcroFields form) throws IOException, DocumentException {
if (baseFont == null) {
baseFont = BaseFont.createFont("pdf/simhei.ttf", BaseFont.IDENTITY_H, BaseFont.EMBEDDED);
}
for (Map.Entry entry : map.entrySet()) {
String key = (String) entry.getKey();
String value = (String) entry.getValue();
form.setFieldProperty(key, "textfont", baseFont, null);
form.setField(key, value);
}
}
Excel 模板 欄位如何申明
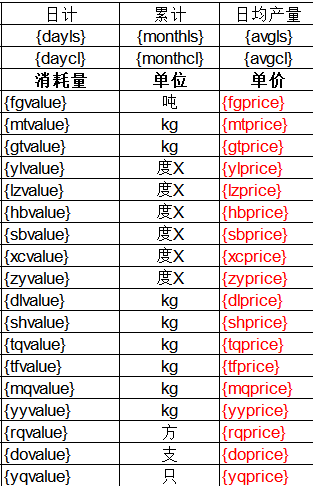
引入maven
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>easyexcel-core</artifactId>
<version>3.2.0</version>
<scope>compile</scope>
</dependency>
Java 程式碼
import com.alibaba.excel.EasyExcel;
import com.alibaba.excel.ExcelWriter;
import com.alibaba.excel.write.metadata.WriteSheet;
/**
* 匯出月均成本
*
* @param costBO
* @return
*/
@Override
public String simpleFillExcel(MothlyCostBO costBO) {
// 獲取當天的資料
ProductionRecordMonthlyCostVO dayCostVO = LedgerReportMapper.ProductionPlanCostLedgerReportDetailByDay(costBO);
// 當月 月初 到當前日期的 資料
ProductionRecordMonthlyCostVO monthlyCostVO = LedgerReportMapper.ProductionPlanCostLedgerReportDetailByMonth(costBO);
BigDecimal dayHost = LedgerReportMapper.ProductionPlanRecordHotShutTimeByDay(costBO);
BigDecimal monthHost = LedgerReportMapper.ProductionPlanRecordHotShutTimeByMonth(costBO);
ProductionExcelDownloadVO downloadVO = new ProductionExcelDownloadVO();
// 每日可以為0
if (JSONUtil.isNull(dayCostVO)) {
dayCostVO = new MindaProductionRecordMonthlyCostVO();
// 設定所有屬性為 BigDecimal.ZERO
dayCostVO.setSumFurnace(BigDecimal.ZERO);
dayCostVO.setSumSteel(BigDecimal.ZERO);
dayCostVO.setSumFerromaganese(BigDecimal.ZERO);
dayCostVO.setSumFurnacePower(BigDecimal.ZERO);
dayCostVO.setSumFurnaceElectrode(BigDecimal.ZERO);
dayCostVO.setSumFurnaceLime(BigDecimal.ZERO);
dayCostVO.setSumCarBon(BigDecimal.ZERO);
dayCostVO.setSumToner(BigDecimal.ZERO);
dayCostVO.setSumFurnaceMagnesium(BigDecimal.ZERO);
dayCostVO.setSumLox(BigDecimal.ZERO);
dayCostVO.setSumFurnaceNatural(BigDecimal.ZERO);
dayCostVO.setSumFurnaceThermocouple(BigDecimal.ZERO);
dayCostVO.setSumFurnaceSampler(BigDecimal.ZERO);
dayCostVO.setSumRefineLime(BigDecimal.ZERO);
dayCostVO.setSumSilicoferrite(BigDecimal.ZERO);
dayCostVO.setSumFluorite(BigDecimal.ZERO);
dayCostVO.setSumCarburizer(BigDecimal.ZERO);
dayCostVO.setSumInsulation(BigDecimal.ZERO);
dayCostVO.setSumSilicon(BigDecimal.ZERO);
dayCostVO.setSumSiliconAlloy(BigDecimal.ZERO);
dayCostVO.setSumDeaerator(BigDecimal.ZERO);
dayCostVO.setSumRefinePower(BigDecimal.ZERO);
dayCostVO.setSumLiquid(BigDecimal.ZERO);
dayCostVO.setSumRefineElectrode(BigDecimal.ZERO);
dayCostVO.setSumRefineNatural(BigDecimal.ZERO);
dayCostVO.setSumRefineThermocouple(BigDecimal.ZERO);
dayCostVO.setSumRefineSampler(BigDecimal.ZERO);
dayCostVO.setSumHighVanadium(BigDecimal.ZERO);
dayCostVO.setSumCastingPowder(BigDecimal.ZERO);
dayCostVO.setSumCastingInsulation(BigDecimal.ZERO);
dayCostVO.setSumSaladOil(BigDecimal.ZERO);
dayCostVO.setSumCopperPipe(BigDecimal.ZERO);
dayCostVO.setSumCastingNatural(BigDecimal.ZERO);
dayCostVO.setSumCastingThermocouple(BigDecimal.ZERO);
dayCostVO.setSumPowerCasting(BigDecimal.ZERO);
dayCostVO.setSumProtection(BigDecimal.ZERO);
dayCostVO.setSumPump(BigDecimal.ZERO);
dayCostVO.setSumDrive(BigDecimal.ZERO);
dayCostVO.setSumOxygen(BigDecimal.ZERO);
}
// 每月不能為null
if (monthlyCostVO.getSumDay().compareTo(BigDecimal.ZERO) > 0) {
if (dayHost == null) {
downloadVO = setterDownloadVO(dayCostVO, monthlyCostVO, BigDecimal.ZERO, monthHost, costBO);
} else {
downloadVO = setterDownloadVO(dayCostVO, monthlyCostVO, dayHost, monthHost, costBO);
}
}
// 生成Excel檔案
String templateFileName = "pdf/cost1.xlsx";
String filechirldName = DateUtils.getNowTimeStamp().toString();
String outputFileName = "pdf/" + filechirldName + ".xlsx";
try {
// 填充資料
ExcelWriter excelWriter = EasyExcel.write(outputFileName)
.inMemory(true)
.withTemplate(templateFileName).build();
WriteSheet writeSheet = EasyExcel.writerSheet().build();
excelWriter.fill(downloadVO, writeSheet);
excelWriter.writeContext().writeWorkbookHolder().getWorkbook();
Workbook workbook = excelWriter.writeContext().writeWorkbookHolder().getWorkbook();
workbook.getCreationHelper().createFormulaEvaluator().evaluateAll(); // 強制計算公式
excelWriter.finish();
File pdfFile = new File(outputFileName);
// 上傳 oss
Map<String, String> ossMap = AliYunOSSUtils.uploadFile(pdfFile, filechirldName + ".xlsx");
if (StringUtils.isEmpty(ossMap.get("url"))) {
throw new RuntimeException("上傳oss 失敗");
}
pdfFile.delete();
return ossMap.get("url");
} catch (Exception e) {
e.printStackTrace();
throw new RuntimeException("填充Excel失敗");
}
}