使用前面學習的相關元件和api實現聯絡人的CRUD; 效果如下
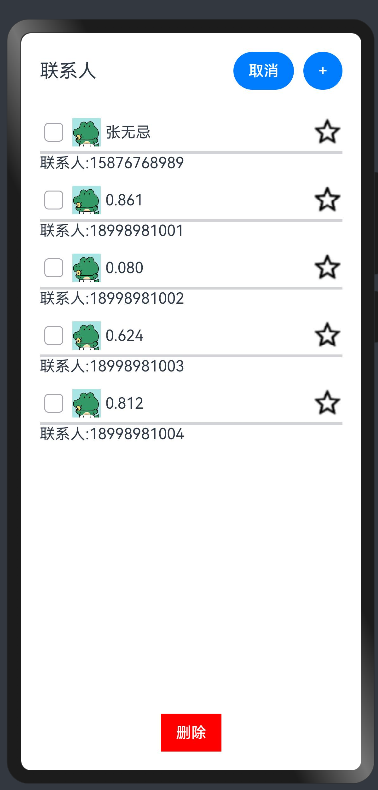
父元件
import { Contacts } from '../domain/Model'
import ContactsItem from '../components/ContactsItem'
@Entry
@Component
struct ContactsExample {
// 聯絡人陣列
@State contactsArr: Contacts[] = [
new Contacts(1, '張三丰', false, false, '18898789098', false),
new Contacts(2, '張無忌', false, false, '15876768989', false)
]
// 核取方塊|刪除按鈕 顯示與隱藏
@State isShow: boolean = false
@State next: number = 1000
build() {
Column() {
// 頂部
Row() {
Text('聯絡人').fontSize(20)
Blank()
Button(this.isShow ? '取消' : '選擇').onClick(() => {
this.isShow = !this.isShow
})
Button('+').margin({ left: 10 }).onClick(() => {
this.contactsArr.push(new Contacts(this.next++, Math.random().toFixed(3)
.toString(), false, false, `1899898${this.next}`, false))
})
}.width('100%')
// body 聯絡人資訊
List({ space: 5 }) {
ForEach(this.contactsArr, (contacts: Contacts, index: number) => {
ListItem() {
ContactsItem({ contacts: contacts, isShow: $isShow })
}
}, (contacts: Contacts) => contacts.id.toString())
}.margin({ top: 20 }).layoutWeight(1)
if (this.isShow) {
// 底部
Button('刪除')
.backgroundColor(Color.Red)
.type(ButtonType.Normal)
.onClick(() => {
this.contactsArr = this.contactsArr.filter(item => !item.isSelect)
})
}
}.width('100%')
.padding(20)
}
}
子元件
// 聯絡人元件
import { Contacts } from '../domain/Model'
@Component
export default struct ContactsItem {
@ObjectLink contacts: Contacts
@Link isShow: boolean
build() {
Column() {
// 聯絡人 | 收藏
Row({ space: 5 }) {
if (this.isShow) {
// 核取方塊
Checkbox().select(this.contacts.isSelect).onChange((val) => {
console.log(val + "")
this.contacts.isSelect = val
})
}
// 頭像
Image($rawfile('avatar.jpg')).width(30).height(30)
// 聯絡人名稱
Text(this.contacts.name)
Blank()
// 收藏按鈕
Image($rawfile(this.contacts.isSave ? 'collection.png' : 'cancel_collection.png'))
.width(30)
.height(30)
.onClick(() => {
console.log(this.contacts.isSave + "")
this.contacts.isSave = !this.contacts.isSave
})
}.width('100%')
.onClick(() => {
this.contacts.isExpand = !this.contacts.isExpand
})
// 分割線
Divider().strokeWidth(3).margin({ top: 5 })
if (this.contacts.isExpand) {
Row() {
Text('聯絡人:')
Text(this.contacts.phone)
}.width('100%')
}
}.width('100%')
.margin({ top: 10 })
}
}
模型(class類)
@Observed
export class Contacts {
id: number
name: string
isSelect: boolean
isSave: boolean
phone: string
isExpand: boolean
constructor(id: number, name: string, isSelect: boolean, isSave: boolean, phone: string, isExpand: boolean) {
this.id = id
this.name = name
this.isSelect = isSelect
this.isSave = isSave
this.phone = phone
this.isExpand = isExpand
}
}