Matplotlib 03-佈局格式定方圓
Matplotlib 03-佈局格式定方圓
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
plt.rcParams['font.sans-serif'] = ['SimHei']
plt.rcParams['axes.unicode_minus'] = False
一、子圖
1. 使用 plt.subplots
繪製均勻狀態下的子圖
返回元素分別是畫布和子圖構成的列表,第一個數字為行,第二個為列
figsize
引數可以指定整個畫布的大小
sharex
和 sharey
分別表示是否共享橫軸和縱軸刻度
tight_layout
函式可以調整子圖的相對大小使字元不會重疊
fig, axs = plt.subplots(2, 5, figsize=(10, 4), sharex=True, sharey=True)
fig.suptitle('樣例1', size=20)
for i in range(2):
for j in range(5):
axs[i][j].scatter(np.random.randn(10), np.random.randn(10))
axs[i][j].set_title('第%d行,第%d列'%(i+1,j+1))
axs[i][j].set_xlim(-5,5)
axs[i][j].set_ylim(-5,5)
if i==1: axs[i][j].set_xlabel('橫座標')
if j==0: axs[i][j].set_ylabel('縱座標')
fig.tight_layout()
除了常規的直角座標系,也可以通過projection
方法建立極座標系下的圖表
N = 150
r = 2 * np.random.rand(N)
theta = 2 * np.pi * np.random.rand(N)
area = 200 * r**2
colors = theta
plt.subplot(projection='polar')
plt.scatter(theta, r, c=colors, s=area, cmap='hsv', alpha=0.75)
plt.show()
2. 使用 GridSpec
繪製非均勻子圖
所謂非均勻包含兩層含義,第一是指圖的比例大小不同但沒有跨行或跨列,第二是指圖為跨列或跨行狀態
利用 add_gridspec
可以指定相對寬度比例 width_ratios
和相對高度比例引數 height_ratios
fig = plt.figure(figsize=(10, 4))
spec = fig.add_gridspec(nrows=2, ncols=5, width_ratios=[1,2,3,4,5], height_ratios=[1,3])
fig.suptitle('樣例2', size=20)
for i in range(2):
for j in range(5):
ax = fig.add_subplot(spec[i, j])
ax.scatter(np.random.randn(10), np.random.randn(10))
ax.set_title('第%d行,第%d列'%(i+1,j+1))
if i==1: ax.set_xlabel('橫座標')
if j==0: ax.set_ylabel('縱座標')
fig.tight_layout()
在上面的例子中出現了 spec[i, j]
的用法,事實上通過切片就可以實現子圖的合併而達到跨圖的共能
fig = plt.figure(figsize=(10, 4))
spec = fig.add_gridspec(nrows=2, ncols=6, width_ratios=[2,2.5,3,1,1.5,2], height_ratios=[1,2])
fig.suptitle('樣例3', size=20)
# sub1
ax = fig.add_subplot(spec[0, :3])
ax.scatter(np.random.randn(10), np.random.randn(10))
# sub2
ax = fig.add_subplot(spec[0, 3:5])
ax.scatter(np.random.randn(10), np.random.randn(10))
# sub3
ax = fig.add_subplot(spec[:, 5])
ax.scatter(np.random.randn(10), np.random.randn(10))
# sub4
ax = fig.add_subplot(spec[1, 0])
ax.scatter(np.random.randn(10), np.random.randn(10))
# sub5
ax = fig.add_subplot(spec[1, 1:5])
ax.scatter(np.random.randn(10), np.random.randn(10))
fig.tight_layout()
二、子圖上的方法
在 ax
物件上定義了和 plt
類似的圖形繪製函式,常用的有: plot, hist, scatter, bar, barh, pie
fig, ax = plt.subplots(figsize=(4,3))
ax.plot([1,2],[2,1])
plt.show()
fig, ax = plt.subplots(figsize=(4,3))
ax.hist(np.random.randn(1000))
plt.show()
常用直線的畫法為: axhline, axvline, axline
(水平、垂直、任意方向)
fig, ax = plt.subplots(figsize=(4,3))
ax.axhline(0.5,0.2,0.8)
ax.axvline(0.5,0.2,0.8)
ax.axline([0.3,0.3],[0.7,0.7])
plt.show()
使用 grid
可以加灰色網格
fig, ax = plt.subplots(figsize=(4,3))
ax.grid(True)
使用 set_xscale, set_title, set_xlabel
分別可以設定座標軸的規度(指對數座標等)、標題、軸名
fig, axs = plt.subplots(1, 2, figsize=(10, 4))
fig.suptitle('大標題', size=20)
for j in range(2):
axs[j].plot(list('abcd'), [10**i for i in range(4)])
if j==0:
axs[j].set_yscale('log')
axs[j].set_title('子標題1')
axs[j].set_ylabel('對數座標')
else:
axs[j].set_title('子標題1')
axs[j].set_ylabel('普通座標')
fig.tight_layout()
與一般的 plt
方法類似, legend, annotate, arrow, text
物件也可以進行相應的繪製
fig, ax = plt.subplots()
ax.arrow(0, 0, 1, 1, head_width=0.03, head_length=0.05, facecolor='red', edgecolor='blue')
ax.text(x=0, y=0,s='這是一段文字', fontsize=16, rotation=70, rotation_mode='anchor', color='green')
ax.annotate('這是中點', xy=(0.5, 0.5), xytext=(0.8, 0.2), arrowprops=dict(facecolor='yellow', edgecolor='black'), fontsize=16)
Text(0.8, 0.2, '這是中點')
fig, ax = plt.subplots()
ax.plot([1,2],[2,1],label="line1")
ax.plot([1,1],[1,2],label="line1")
ax.legend(loc=1)
plt.show()
其中,圖例的 loc
引數如下:
string | code |
---|---|
best | 0 |
upper right | 1 |
upper left | 2 |
lower left | 3 |
lower right | 4 |
right | 5 |
center left | 6 |
center right | 7 |
lower center | 8 |
upper center | 9 |
center | 10 |
作業
1. 墨爾本1981年至1990年的每月溫度情況
ex1 = pd.read_csv('../data/layout_ex1.csv')
ex1.head()
Time | Temperature | |
---|---|---|
0 | 1981-01 | 17.712903 |
1 | 1981-02 | 17.678571 |
2 | 1981-03 | 13.500000 |
3 | 1981-04 | 12.356667 |
4 | 1981-05 | 9.490323 |
- 請利用資料,畫出如下的圖:
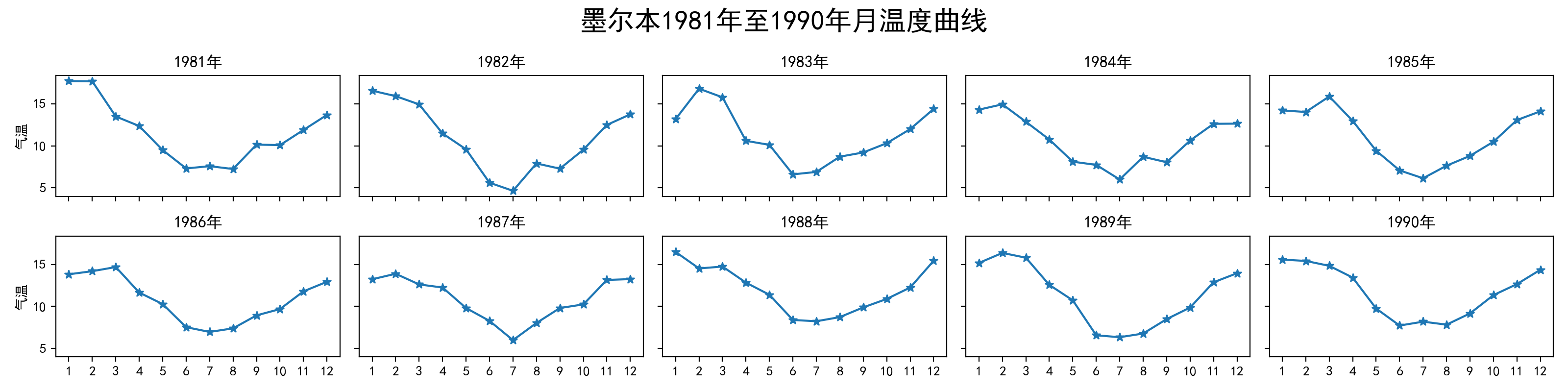
ex1[['Year','Month']] = [*ex1['Time'].apply(lambda x:x.split('-'))]
ex1['Month'] = ex1['Month'].apply(lambda x:int(x))
ex1.head()
Time | Temperature | Year | Month | |
---|---|---|---|---|
0 | 1981-01 | 17.712903 | 1981 | 1 |
1 | 1981-02 | 17.678571 | 1981 | 2 |
2 | 1981-03 | 13.500000 | 1981 | 3 |
3 | 1981-04 | 12.356667 | 1981 | 4 |
4 | 1981-05 | 9.490323 | 1981 | 5 |
fig, axs = plt.subplots(2, 5, figsize=(12, 4), sharex=True, sharey=True)
fig.suptitle('墨爾本1981年至1990年月溫度曲線', size=15)
axs, gb = axs.flatten() , ex1.groupby('Year')
for i,year in enumerate(gb.groups.keys()):
group = gb.get_group(year)
axs[i].plot(group.Month, group.Temperature, marker='*')
axs[i].set_title(f'{year}年')
if i//5: axs[i].set_xlabel('Month'), axs[i].set_xticks(group.Month)
if not i%5: axs[i].set_ylabel('Temperature')
fig.tight_layout()
2. 畫出資料的散點圖和邊際分佈
- 用
np.random.randn(2, 150)
生成一組二維資料,使用兩種非均勻子圖的分割方法,做出該資料對應的散點圖和邊際分佈圖
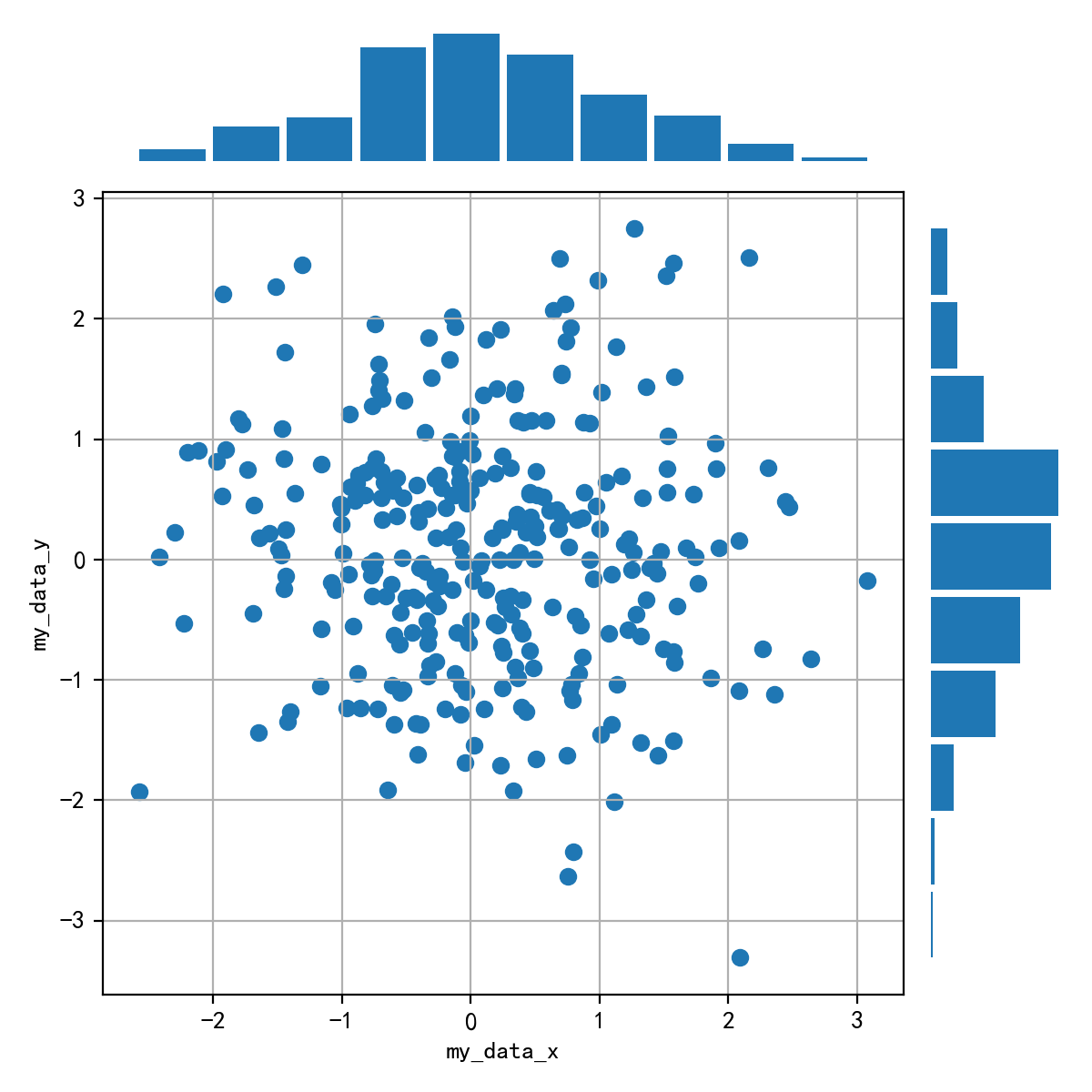
#非均勻子圖分割方法一
np.random.seed(666)
x,y = np.random.randn(2,300)
fig = plt.figure(figsize=(7,6))
spec = fig.add_gridspec(nrows=2, ncols=2, width_ratios=[5,1], height_ratios=[1,5])
ax = fig.add_subplot(spec[0,0])
ax.hist(x, rwidth=0.9, density=True)
ax.axis('off')
ax = fig.add_subplot(spec[1,0])
ax.scatter(x,y)
ax.set_xlabel('data_x')
ax.set_ylabel('data_y')
ax.grid()
ax = fig.add_subplot(spec[1,1])
ax.hist(y, orientation='horizontal', rwidth=0.9, density=True)
ax.axis('off')
fig.tight_layout()
#非均勻子圖分割方法二
np.random.seed(888)
x,y = np.random.randn(2,300)
fig = plt.figure(figsize=(7, 6))
spec = fig.add_gridspec(nrows=5, ncols=4, width_ratios=[1.1,1.6,2.3,1], height_ratios=[0.2,0.8,2.4,1.3,1.9])
ax = fig.add_subplot(spec[:2, :-1])
ax.hist(x, rwidth=0.9, density=True)
ax.axis('off')
ax = fig.add_subplot(spec[2:, :-1])
ax.scatter(x,y)
ax.set_xlabel('data_x')
ax.set_ylabel('data_y')
ax.grid()
ax = fig.add_subplot(spec[2:, -1:])
ax.hist(y, orientation='horizontal', rwidth=0.9, density=True)
ax.axis('off')
fig.tight_layout()
相關文章
- GridView實現方塊佈局View
- DialogPane對話方塊佈局
- scss中迴圈之@for迴圈佈局畫圓CSS
- flutter佈局-7-About對話方塊Flutter
- Android 實現一個通用的圓角佈局Android
- Vue專案rem佈局設定VueREM
- CSS佈局 --- 居中佈局CSS
- css佈局-float佈局CSS
- 阿里投資圓通快遞 馬雲物流佈局再獲拼圖阿里
- 居中佈局、三欄佈局
- qt 佈局---表單佈局QT
- java:佈局方法(流佈局)Java
- flex佈局(彈性佈局)Flex
- 佈局技巧:合併佈局
- felx佈局,需要定寬的,需注意:
- 【筆記】圓方樹筆記
- Matplotlib直方圖繪製技巧直方圖
- CSS 佈局之水平居中佈局CSS
- CSS佈局之三欄佈局CSS
- 浮動佈局 和 flex佈局Flex
- CSS佈局 --- 自適應佈局CSS
- CSS佈局 --- 等寬&等高佈局CSS
- 彈性佈局(伸縮佈局)
- 佈局
- [翻譯] 理解 CSS 佈局和塊級格式上下文CSS
- CSS經典佈局——聖盃佈局與雙飛翼佈局CSS
- 定製化根佈局(Kotlin實現)Kotlin
- CSS佈局–聖盃佈局和雙飛翼佈局以及使用Flex實現聖盃佈局CSSFlex
- css佈局系列1——盒模型佈局CSS模型
- 佈局管理器——相對佈局
- 聖盃佈局?雙飛翼佈局?
- 網頁佈局------幾種佈局方式網頁
- CSS 三欄佈局之聖盃佈局和雙飛翼佈局CSS
- 03-前後端資料傳輸格式-下後端
- 運籌帷幄,全面佈局,圓心科技上市程式進一步加快
- 3個自定義view佈局:矩形TextView,圓形進度條,圓環viewTextView
- iOS自動佈局(Autolayout)之VFL(視覺化格式語言)iOS視覺化
- flex佈局不相容ie怎麼設定Flex