父元件檔案:parentcomponent.vue
子元件檔案:childcomponent.vue
- 傳普通值
- 傳動態值
- 傳物件
- 傳陣列
<!-- 父元件 -->
<template>
<h1>I am ParentComponent</h1>
<ChildComponent msg="nice"/>
</template>
<script setup>
import ChildComponent from './ChildComponent.vue'
</script>
一、傳普通值
<!-- 父元件 -->
<template>
<h1>I am ParentComponent</h1>
<ChildComponent msg="nice"/>
</template>
<script setup>
import ChildComponent from './ChildComponent.vue'
</script>
<!-- 子元件 -->
<template>
<h2>I am ChildComponent</h2>
<p>I want {{ props.msg }} from my Parent.</p>
</template>
<script setup>
import { defineProps } from 'vue';
const props = defineProps({ msg:String, })
</script>
二、傳動態值
<!-- 父元件 -->
<template>
<h1>I am ParentComponent</h1>
<ChildComponent :msg="message"/>
</template>
<script setup>
import ChildComponent from './ChildComponent.vue'
const message = "動態傳值"
</script>
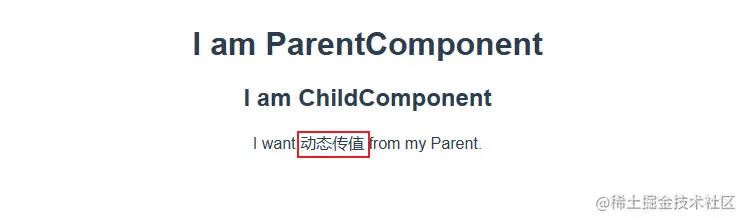
可能會有疑惑,defineProps是什麼呢?
它其實就是一個API函式。defineProps
是 Vue 3 中的一個函式,用於定義元件的 props。與 Vue 2 中的 props
配置選項不同,使用 defineProps
函式定義的 props 是隻讀的響應式物件,它們的值由父元件傳遞而來,不能被子元件修改。這有助於提高元件的可維護性和可重用性,並減少不必要的副作用。 簡單理解:就是用於拿父元件傳過來的值,且子元件不能修改!
三、傳物件
<!-- 父元件 -->
<template>
<h1>I am ParentComponent</h1>
<ChildComponent :arrMsg="A"/>
</template>
<script setup>
import ChildComponent from './ChildComponent.vue'
const A = [
{id:1,name:'Kiangkiang'},
{id:2,name:'Xiaohong'},
{id:3,name:'Xiaoma'}
]
</script>
<!-- 子元件 -->
<template>
<h2>I am ChildComponent</h2>
<h3>陣列</h3>
<ul v-for="item in props.arrMsg" :key="item.id">
<li>{{ item.id }}-{{ item.name }}</li>
</ul>
</template>
<script setup>
import { defineProps } from 'vue';
const props = defineProps({
arrMsg:Array//接收父元件ParentComponent傳過來的陣列
})
</script>
四、傳陣列
<!-- 父元件 -->
<template>
<h1>I am ParentComponent</h1>
<ChildComponent :list="ListMsg"/>
</template>
<script setup>
import ChildComponent from './ChildComponent.vue'
const ListMsg = {
name:'Xiaoma', age:'18', gender:'Boy',
}
</script>
<!-- 子元件 -->
<template>
<h2>I am ChildComponent</h2>
<h3>個人資訊</h3>
<ul>
<li>{{ props.list.name }}</li>
<li>{{ props.list.age }}</li>
<li>{{ props.list.gender }}</li>
</ul>
</template>
<script setup>
import { defineProps } from 'vue';
const props = defineProps({
list:Object,
})
</script>
