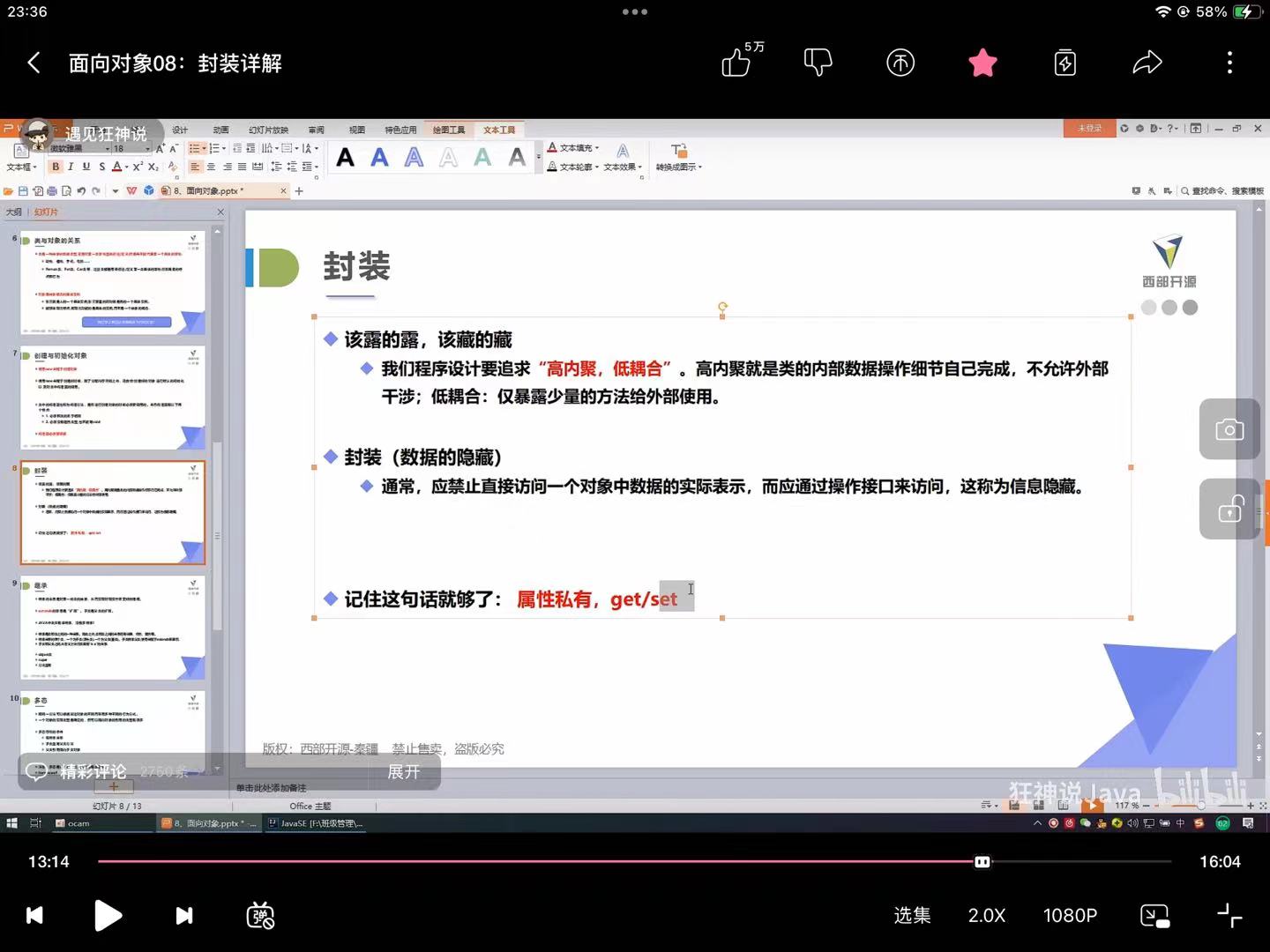
package com.oop.demo04; //類 private:私有 public class Student { //屬性私有,封裝大多數時候都是對於屬性來的 private String name;//名字,以前public所有人都可以操作這個名字,現在屬性私有就不讓所有人都可以操縱這個屬性了 private int id;//學號 private char sex;//性別 private int age; //提供一些可以操作這個屬性的方法! //提供一些public的get、set方法 //get獲得這個資料 public String getName(){ return this.name; } //set 給這個資料設定值 public void setName(String name){ this.name = name; } //alt+insert,選中屬性可以自動給屬性設定get和set方法 public char getSex() { return sex; } public void setSex(char sex) { this.sex = sex; } public int getAge() { return age; } public void setAge(int age) { if(age>120 || age<0){ this.age = age; }else{ age=3;//安全性檢查 } } }
package com.oop; import com.oop.demo04.Student; /* * 封裝的意義 * 1.提高程式的安全性 * 2.隱藏程式碼的實現細節 * 3.統一介面 * 4.系統的可維護性增加了 * */ public class Application { public static void main(String[] args) { Student s1 = new Student(); s1.setName("qingjiang"); System.out.println(s1.getName()); s1.setAge(999);//把年齡設定成這樣是不合法的,所以需要在封裝時做一些安全性檢查 System.out.println(s1.getAge()); } }