ListView是一個經常用到的控制元件,ListView裡面的每個子項Item可以使一個字串,也可以是一個組合控制元件。
在android中,由於資料來源多種多樣,如從資原始檔讀取、從資料庫中讀取、從網路上其他地方讀取,而最終這些資料都將被展示在ListView中,所以android就用adapter設計模式,對應每種資料來源使用對應的adapter來連線資料和檢視。Adapter就是資料和檢視之間的橋樑,資料在adapter中做處理,然後顯示到ListView上面。
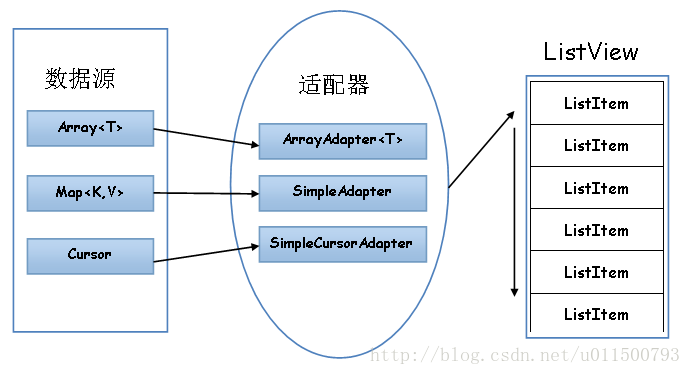
下面主要介紹三種adapter:ArrayAdapter<T>、SimpleAdapter和SimpleCursorAdapter。
1.ArrayAdapter<T>
效果圖如下:
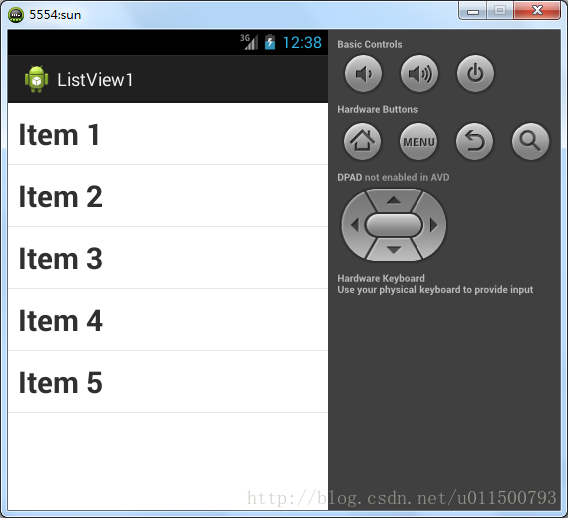
首先建立存放ListView的Activity所需要的佈局activity_main.xml檔案。
-
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
-
xmlns:tools="http://schemas.android.com/tools"
-
android:layout_width="match_parent"
-
android:layout_height="match_parent"
-
tools:context=".MainActivity" >
-
-
<ListView
-
android:id="@+id/list"
-
android:layout_width="match_parent"
-
android:layout_height="match_parent" />
-
</LinearLayout>
上面程式碼建立了一個佈局配置檔案,裡面只放了一個ListView控制元件,將其ID設定為:list。
接下來是list_item.xml,用來設定ListView中每個Item的佈局,是ListItem的XML實現。
Android提供了多種ListItem的Layout (R.layout),以下是較為常用的:
android.R.layout.simple_list_item_1 一行text
android.R.layout.simple_list_item_2 一行title,一行text
android.R.layout.simple_list_item_single_choice 單選按鈕
android.R.layout.simple_list_item_multiple_choice 多選按鈕
android.R.layout.simple_list_item_checked checkbox
我們可以自定義自己的Layout(list_item.xml):
-
<TextView xmlns:android="http://schemas.android.com/apk/res/android"
-
android:layout_width="match_parent"
-
android:layout_height="match_parent"
-
android:textStyle="bold"
-
android:textSize="30sp"
-
android:padding="10sp">
-
</TextView>
要注意的是自定義list_item.xml的根節點必須是TextView,否則就會有ArrayAdapter requires the
resource ID to be a TextView的錯誤。
最後是MainActivity.java程式碼,先找出ListView,然後往ListView裡填充陣列data。
-
@Override
-
protected void onCreate(Bundle savedInstanceState) {
-
super.onCreate(savedInstanceState);
-
setContentView(R.layout.activity_main);
-
-
String[] data = { "Item 1", "Item 2", "Item 3", "Item 4", "Item 5" };
-
-
-
ListView listView = (ListView) findViewById(R.id.list1);
-
-
ArrayAdapter<String> arrayAdapter = new ArrayAdapter<String>(this,
-
R.layout.list_item, data);
-
-
-
-
-
-
-
listView.setAdapter(arrayAdapter);
-
}
2.SimpleAdapter
效果圖如下:
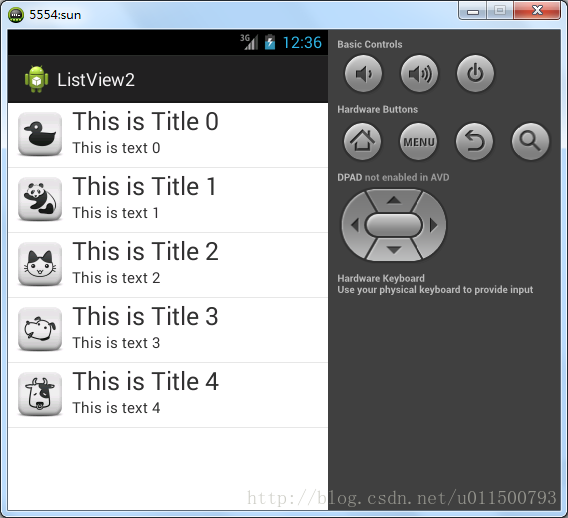
首先建立存放ListView的Activity所需要的佈局activity_main.xml檔案。
-
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
-
xmlns:tools="http://schemas.android.com/tools"
-
android:layout_width="match_parent"
-
android:layout_height="match_parent"
-
tools:context=".MainActivity" >
-
-
<ListView
-
android:id="@+id/list"
-
android:layout_width="fill_parent"
-
android:layout_height="fill_parent" />
-
</LinearLayout>
和之前一樣建立了一個佈局配置檔案,裡面只放了一個ListView控制元件,將其ID設定為:list。
接下來是list_item.xml,用來設定ListView中每個Item的佈局,是ListItem的XML實現。
-
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
-
xmlns:tools="http://schemas.android.com/tools"
-
android:layout_width="match_parent"
-
android:layout_height="wrap_content" >
-
-
<ImageView
-
android:id="@+id/ItemImage"
-
android:layout_width="wrap_content"
-
android:layout_height="wrap_content" >
-
</ImageView>
-
-
<TextView
-
android:id="@+id/ItemTitle"
-
android:layout_width="wrap_content"
-
android:layout_height="wrap_content"
-
android:layout_toRightOf="@id/ItemImage"
-
android:textSize="25sp" >
-
</TextView>
-
-
<TextView
-
android:id="@+id/ItemText"
-
android:layout_width="wrap_content"
-
android:layout_height="wrap_content"
-
android:layout_toRightOf="@id/ItemImage"
-
android:layout_below="@id/ItemTitle"
-
android:textSize="15sp" >
-
</TextView>
-
-
</RelativeLayout>
最後是MainActivity.java程式碼,先找出ListView,然後往ListView裡填充動態陣列data。
-
@Override
-
protected void onCreate(Bundle savedInstanceState) {
-
super.onCreate(savedInstanceState);
-
setContentView(R.layout.activity_main);
-
-
-
int[] images = new int[] { R.drawable.item_img_a,
-
R.drawable.item_img_b, R.drawable.item_img_c,
-
R.drawable.item_img_d, R.drawable.item_img_e };
-
-
-
List<HashMap<String, Object>> data = new ArrayList<HashMap<String, Object>>();
-
for (int i = 0; i < 5; i++) {
-
HashMap<String, Object> map = new HashMap<String, Object>();
-
map.put("ItemImage", images[i]);
-
map.put("ItemTitle", "This is Title " + i);
-
map.put("ItemText", "This is text " + i);
-
data.add(map);
-
}
-
-
-
ListView listView = (ListView) findViewById(R.id.list);
-
-
-
String[] from = new String[] { "ItemImage", "ItemTitle", "ItemText" };
-
-
int[] to = new int[] { R.id.ItemImage, R.id.ItemTitle, R.id.ItemText };
-
-
-
-
SimpleAdapter adapter = new SimpleAdapter(this, data,
-
R.layout.list_item, from, to);
-
listView.setAdapter(adapter);
-
}
3.SimpleCursorAdapter
下面用SimpleCursorAdapter來實現上一節中用SimpleAdapter實現的同樣的效果,activity_main.xml檔案和list_item.xml檔案都不需要更改,只需要更改MainActivity.java程式碼。
-
@Override
-
protected void onCreate(Bundle savedInstanceState) {
-
super.onCreate(savedInstanceState);
-
setContentView(R.layout.activity_main);
-
-
DBHelper dbHelper = new DBHelper(this);
-
-
insertDataIntoDB(dbHelper);
-
Cursor cursor = dbHelper.query();
-
-
-
ListView listView = (ListView) findViewById(R.id.list);
-
-
-
String[] from = new String[] { "ItemImage", "ItemTitle", "ItemText" };
-
-
int[] to = new int[] { R.id.ItemImage, R.id.ItemTitle, R.id.ItemText };
-
-
-
-
SimpleCursorAdapter adapter = new SimpleCursorAdapter(this,
-
R.layout.list_item, cursor, from, to);
-
listView.setAdapter(adapter);
-
}
-
-
private void insertDataIntoDB(DBHelper dbHelper) {
-
dbHelper.clear();
-
-
int[] images = new int[] { R.drawable.item_img_a,
-
R.drawable.item_img_b, R.drawable.item_img_c,
-
R.drawable.item_img_d, R.drawable.item_img_e };
-
-
for (int i = 0; i < 5; i++) {
-
ContentValues values = new ContentValues();
-
values.put("ItemImage", images[i]);
-
values.put("ItemTitle", "This is Title " + i);
-
values.put("ItemText", "This is text " + i);
-
dbHelper.insert(values);
-
}
-
}
這裡通過DBHelper這個類來實現資料庫的插入和查詢功能。
-
public class DBHelper extends SQLiteOpenHelper {
-
-
public DBHelper(Context context) {
-
super(context, "testDB", null, 1);
-
}
-
-
@Override
-
public void onCreate(SQLiteDatabase db) {
-
String createTableSQL = "create table IF NOT EXISTS tbl_test "
-
+ "(_id integer primary key autoincrement, ItemImage int, "
-
+ "ItemTitle text, ItemText text)";
-
db.execSQL(createTableSQL);
-
}
-
-
public void insert(ContentValues values) {
-
SQLiteDatabase db = getWritableDatabase();
-
db.insert("tbl_test", null, values);
-
}
-
-
public Cursor query() {
-
SQLiteDatabase db = getWritableDatabase();
-
Cursor cursor = db.query("tbl_test", null, null, null, null, null, null);
-
return cursor;
-
}
-
-
public void clear() {
-
SQLiteDatabase db = getWritableDatabase();
-
db.delete("tbl_test", null, null);
-
}
-
-
public void close() {
-
SQLiteDatabase db = getWritableDatabase();
-
db.close();
-
}
-
-
@Override
-
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
-
}
-
-
}
參考文章:
http://stephen830.iteye.com/blog/1139917
http://blog.csdn.net/hellogv/article/details/4542668
http://blog.csdn.net/hellogv/article/details/4548659
http://blog.csdn.net/xxg3053/article/details/6999872
http://gundumw100.iteye.com/blog/875967
http://android.blog.51cto.com/268543/336162/