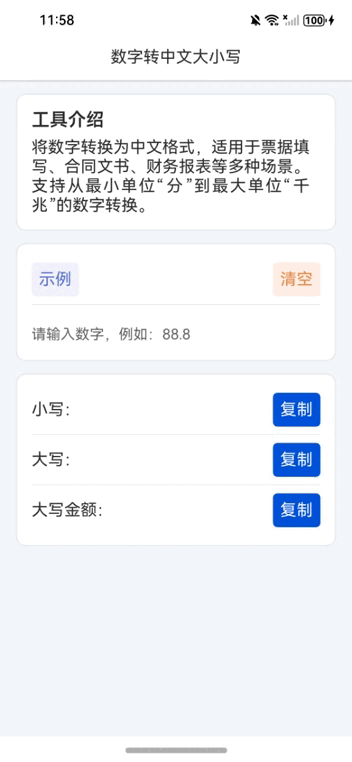
【引言】
本應用的主要功能是將使用者輸入的數字轉換為中文的小寫、大寫及大寫金額形式。使用者可以在輸入框中輸入任意數字,點選“示例”按鈕可以快速填充預設的數字,點選“清空”按鈕則會清除當前輸入。轉換結果顯示在下方的結果區域,每個結果旁邊都有一個“複製”按鈕,方便使用者將結果複製到剪貼簿。
【環境準備】
• 作業系統:Windows 10
• 開發工具:DevEco Studio NEXT Beta1 Build Version: 5.0.3.806
• 目標裝置:華為Mate60 Pro
• 開發語言:ArkTS
• 框架:ArkUI
• API版本:API 12
• 三方庫:chinese-number-format(數字轉中文)、chinese-finance-number(將數字轉換成財務用的中文大寫數字)
ohpm install @nutpi/chinese-finance-number ohpm install @nutpi/chinese-number-format
【功能實現】
• 輸入監聽:透過 @Watch 裝飾器監聽輸入框的變化,一旦輸入發生變化,即呼叫 inputChanged 方法更新轉換結果。
• 轉換邏輯:利用 @nutpi/chinese-number-format 和 @nutpi/chinese-finance-number 庫提供的方法完成數字到中文的各種轉換。
• 複製功能:使用 pasteboard 模組將結果顯示的中文文字複製到剪貼簿,透過 promptAction.showToast 提示使用者複製成功。
【完整程式碼】
// 匯入必要的模組 import { promptAction } from '@kit.ArkUI'; // 用於顯示提示資訊 import { pasteboard } from '@kit.BasicServicesKit'; // 用於處理剪貼簿操作 import { toChineseNumber } from '@nutpi/chinese-finance-number'; // 將數字轉換為中文大寫金額 import { toChineseWithUnits, // 將數字轉換為帶單位的中文 toUpperCase, // 將中文小寫轉換為大寫 } from '@nutpi/chinese-number-format'; @Entry // 標記此元件為入口點 @Component // 定義一個元件 struct NumberToChineseConverter { @State private exampleNumber: number = 88.8; // 示例數字 @State private textColor: string = "#2e2e2e"; // 文字顏色 @State private lineColor: string = "#d5d5d5"; // 分割線顏色 @State private basePadding: number = 30; // 基礎內邊距 @State private chineseLowercase: string = ""; // 轉換後的小寫中文 @State private chineseUppercase: string = ""; // 轉換後的中文大寫 @State private chineseUppercaseAmount: string = ""; // 轉換後的中文大寫金額 @State @Watch('inputChanged') private inputText: string = ""; // 監聽輸入文字變化 // 當輸入文字改變時觸發的方法 inputChanged() { this.chineseLowercase = toChineseWithUnits(Number(this.inputText), 'zh-CN'); // 轉換為小寫中文並帶上單位 this.chineseUppercase = toUpperCase(this.chineseLowercase, 'zh-CN'); // 將小寫中文轉換為大寫 this.chineseUppercaseAmount = toChineseNumber(Number(this.inputText)); // 轉換為大寫金額 } // 複製文字到剪貼簿的方法 private copyToClipboard(text: string): void { const pasteboardData = pasteboard.createData(pasteboard.MIMETYPE_TEXT_PLAIN, text); // 建立剪貼簿資料 const systemPasteboard = pasteboard.getSystemPasteboard(); // 獲取系統剪貼簿 systemPasteboard.setData(pasteboardData); // 設定剪貼簿資料 promptAction.showToast({ message: '已複製' }); // 顯示覆製成功的提示 } // 構建使用者介面的方法 build() { Column() { // 主列容器 // 頁面標題 Text('數字轉中文大小寫') .fontColor(this.textColor) // 設定字型顏色 .fontSize(18) // 設定字型大小 .width('100%') // 設定寬度 .height(50) // 設定高度 .textAlign(TextAlign.Center) // 文字居中對齊 .backgroundColor(Color.White) // 設定背景顏色 .shadow({ // 新增陰影效果 radius: 2, // 陰影半徑 color: this.lineColor, // 陰影顏色 offsetX: 0, // X軸偏移量 offsetY: 5 // Y軸偏移量 }); Scroll() { // 滾動檢視 Column() { // 內部列容器 // 工具介紹部分 Column() { Text('工具介紹').fontSize(20).fontWeight(600).fontColor(this.textColor); // 設定介紹文字樣式 Text('將數字轉換為中文格式,適用於票據填寫、合同文書、財務報表等多種場景。支援從最小單位“分”到最大單位“千兆”的數字轉換。') .textAlign(TextAlign.JUSTIFY) .fontSize(18).fontColor(this.textColor).margin({ top: `${this.basePadding / 2}lpx` }); // 設定介紹詳情文字樣式 } .alignItems(HorizontalAlign.Start) // 對齊方式 .width('650lpx') // 設定寬度 .padding(`${this.basePadding}lpx`) // 設定內邊距 .margin({ top: `${this.basePadding}lpx` }) // 設定外邊距 .borderRadius(10) // 設定圓角 .backgroundColor(Color.White) // 設定背景顏色 .shadow({ // 新增陰影效果 radius: 10, // 陰影半徑 color: this.lineColor, // 陰影顏色 offsetX: 0, // X軸偏移量 offsetY: 0 // Y軸偏移量 }); // 輸入區 Column() { Row() { // 行容器 Text('示例') .fontColor("#5871ce") // 設定字型顏色 .fontSize(18) // 設定字型大小 .padding(`${this.basePadding / 2}lpx`) // 設定內邊距 .backgroundColor("#f2f1fd") // 設定背景顏色 .borderRadius(5) // 設定圓角 .clickEffect({ level: ClickEffectLevel.LIGHT, scale: 0.8 }) // 設定點選效果 .onClick(() => { // 點選事件 this.inputText = `${this.exampleNumber}`; // 設定輸入框文字為示例數字 }); Blank(); // 佔位符 Text('清空') .fontColor("#e48742") // 設定字型顏色 .fontSize(18) // 設定字型大小 .padding(`${this.basePadding / 2}lpx`) // 設定內邊距 .clickEffect({ level: ClickEffectLevel.LIGHT, scale: 0.8 }) // 設定點選效果 .backgroundColor("#ffefe6") // 設定背景顏色 .borderRadius(5) // 設定圓角 .onClick(() => { // 點選事件 this.inputText = ""; // 清空輸入框 }); }.height(45) // 設定高度 .justifyContent(FlexAlign.SpaceBetween) // 子元素水平分佈方式 .width('100%'); // 設定寬度 Divider().margin({ top: 5, bottom: 5 }); // 分割線 TextInput({ text: $$this.inputText, placeholder: `請輸入數字,例如:${this.exampleNumber}` }) // 輸入框 .width('100%') // 設定寬度 .fontSize(18) // 設定字型大小 .caretColor(this.textColor) // 設定游標顏色 .fontColor(this.textColor) // 設定字型顏色 .margin({ top: `${this.basePadding}lpx` }) // 設定外邊距 .padding(0) // 設定內邊距 .backgroundColor(Color.Transparent) // 設定背景顏色 .borderRadius(0) // 設定圓角 .type(InputType.NUMBER_DECIMAL); // 設定輸入型別為數字 } .alignItems(HorizontalAlign.Start) // 對齊方式 .width('650lpx') // 設定寬度 .padding(`${this.basePadding}lpx`) // 設定內邊距 .margin({ top: `${this.basePadding}lpx` }) // 設定外邊距 .borderRadius(10) // 設定圓角 .backgroundColor(Color.White) // 設定背景顏色 .shadow({ // 新增陰影效果 radius: 10, // 陰影半徑 color: this.lineColor, // 陰影顏色 offsetX: 0, // X軸偏移量 offsetY: 0 // Y軸偏移量 }); // 結果區 Column() { Row() { Text(`小寫:${this.chineseLowercase}`).fontColor(this.textColor).fontSize(18).layoutWeight(1); // 顯示小寫結果 Text('複製') .fontColor(Color.White) // 設定字型顏色 .fontSize(18) // 設定字型大小 .padding(`${this.basePadding / 2}lpx`) // 設定內邊距 .backgroundColor("#0052d9") // 設定背景顏色 .borderRadius(5) // 設定圓角 .clickEffect({ level: ClickEffectLevel.LIGHT, scale: 0.8 }) // 設定點選效果 .onClick(() => { // 點選事件 this.copyToClipboard(this.chineseLowercase); // 複製小寫結果到剪貼簿 }); }.constraintSize({ minHeight: 45 }) // 最小高度 .justifyContent(FlexAlign.SpaceBetween) // 子元素水平分佈方式 .width('100%'); // 設定寬度 Divider().margin({ top: 5, bottom: 5 }); // 分割線 Row() { Text(`大寫:${this.chineseUppercase}`).fontColor(this.textColor).fontSize(18).layoutWeight(1); // 顯示大寫結果 Text('複製') .fontColor(Color.White) // 設定字型顏色 .fontSize(18) // 設定字型大小 .padding(`${this.basePadding / 2}lpx`) // 設定內邊距 .backgroundColor("#0052d9") // 設定背景顏色 .borderRadius(5) // 設定圓角 .clickEffect({ level: ClickEffectLevel.LIGHT, scale: 0.8 }) // 設定點選效果 .onClick(() => { // 點選事件 this.copyToClipboard(this.chineseUppercase); // 複製大寫結果到剪貼簿 }); }.constraintSize({ minHeight: 45 }) // 最小高度 .justifyContent(FlexAlign.SpaceBetween) // 子元素水平分佈方式 .width('100%'); // 設定寬度 Divider().margin({ top: 5, bottom: 5 }); // 分割線 Row() { Text(`大寫金額:${this.chineseUppercaseAmount}`).fontColor(this.textColor).fontSize(18).layoutWeight(1); // 顯示大寫金額結果 Text('複製') .fontColor(Color.White) // 設定字型顏色 .fontSize(18) // 設定字型大小 .padding(`${this.basePadding / 2}lpx`) // 設定內邊距 .backgroundColor("#0052d9") // 設定背景顏色 .borderRadius(5) // 設定圓角 .clickEffect({ level: ClickEffectLevel.LIGHT, scale: 0.8 }) // 設定點選效果 .onClick(() => { // 點選事件 this.copyToClipboard(this.chineseUppercaseAmount); // 複製大寫金額結果到剪貼簿 }); }.constraintSize({ minHeight: 45 }) // 最小高度 .justifyContent(FlexAlign.SpaceBetween) // 子元素水平分佈方式 .width('100%'); // 設定寬度 } .alignItems(HorizontalAlign.Start) // 對齊方式 .width('650lpx') // 設定寬度 .padding(`${this.basePadding}lpx`) // 設定內邊距 .margin({ top: `${this.basePadding}lpx` }) // 設定外邊距 .borderRadius(10) // 設定圓角 .backgroundColor(Color.White) // 設定背景顏色 .shadow({ // 新增陰影效果 radius: 10, // 陰影半徑 color: this.lineColor, // 陰影顏色 offsetX: 0, // X軸偏移量 offsetY: 0 // Y軸偏移量 }); } }.scrollBar(BarState.Off).clip(false); // 關閉捲軸,不允許裁剪 } .height('100%') // 設定高度 .width('100%') // 設定寬度 .backgroundColor("#f4f8fb"); // 設定頁面背景顏色 } }