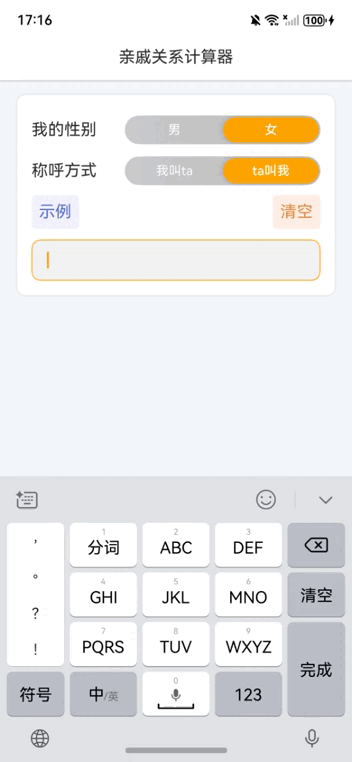
【引言】
在快節奏的現代生活中,人們往往因為忙碌而忽略了與親戚間的互動,特別是在春節期間,面對眾多的長輩和晚輩時,很多人會感到困惑,不知道該如何正確地稱呼每一位親戚。針對這一問題,我們開發了一款基於鴻蒙NEXT平臺的“親戚關係計算器”應用,旨在幫助使用者快速、準確地識別和稱呼他們的親戚。
【環境準備】
• 作業系統:Windows 10
• 開發工具:DevEco Studio NEXT Beta1 Build Version: 5.0.3.806
• 目標裝置:華為Mate60 Pro
• 開發語言:ArkTS
• 框架:ArkUI
• API版本:API 12
• 三方庫:@nutpi/relationship(核心演算法)
【應用背景】
中國社會有著深厚的家庭觀念,親屬關係複雜多樣。從血緣到姻親,從直系到旁系,每一種關係都有其獨特的稱呼方式。然而,隨著社會的發展,家庭成員之間的聯絡逐漸變得疏遠,尤其是對於年輕人來說,準確地稱呼每一位親戚成了一項挑戰。為了應對這一挑戰,“親戚關係計算器”應運而生。
【核心功能】
1. 關係輸入:使用者可以透過介面輸入或選擇具體的親戚關係描述,例如“爸爸的哥哥的兒子”。
2. 性別及稱呼選擇:考慮到不同地區的習俗差異,應用允許使用者選擇自己的性別和希望使用的稱呼方式,比如“哥哥”、“姐夫”等。
3. 關係計算:利用@nutpi/relationship庫,根據使用者提供的資訊,精確計算出正確的親戚稱呼。
4. 示例與清空:提供示例按鈕供使用者測試應用功能,同時也設有清空按鈕方便使用者重新開始。
5. 個性化設定:支援多種方言和地方習慣的稱呼方式,讓應用更加貼近使用者的實際需求。
【使用者介面】
應用的使用者介面簡潔明瞭,主要由以下幾個部分組成:
• 選擇性別:透過分段按鈕讓使用者選擇自己的性別。
• 選擇稱呼方式:另一個分段按鈕讓使用者選擇希望的稱呼方式。
• 輸入關係描述:提供一個文字輸入框,使用者可以在此處輸入具體的關係描述。
• 結果顯示區:在使用者提交資訊後,這裡會顯示出正確的親戚稱呼。
• 操作按鈕:包括示例按鈕、清空按鈕等,方便使用者操作。
【完整程式碼】
導包
ohpm install @nutpi/relationship
程式碼
// 匯入關係計算模組 import relationship from "@nutpi/relationship" // 匯入分段按鈕元件及配置型別 import { SegmentButton, SegmentButtonItemTuple, SegmentButtonOptions } from '@kit.ArkUI'; // 使用 @Entry 和 @Component 裝飾器標記這是一個應用入口元件 @Entry @Component // 定義一個名為 RelationshipCalculator 的結構體,作為元件主體 struct RelationshipCalculator { // 使用者輸入的關係描述,預設值為“爸爸的堂弟” @State private userInputRelation: string = "爸爸的堂弟"; // 應用的主題顏色,設定為橙色 @State private themeColor: string | Color = Color.Orange; // 文字顏色 @State private textColor: string = "#2e2e2e"; // 邊框顏色 @State private lineColor: string = "#d5d5d5"; // 基礎內邊距大小 @State private paddingBase: number = 30; // 性別選項陣列 @State private genderOptions: object[] = [Object({ text: '男' }), Object({ text: '女' })]; // 稱呼方式選項陣列 @State private callMethodOptions: object[] = [Object({ text: '我叫ta' }), Object({ text: 'ta叫我' })]; // 性別選擇按鈕的配置 @State private genderButtonOptions: SegmentButtonOptions | undefined = undefined; // 稱呼方式選擇按鈕的配置 @State private callMethodButtonOptions: SegmentButtonOptions | undefined = undefined; // 當前選中的性別索引 @State @Watch('updateSelections') selectedGenderIndex: number[] = [0]; // 當前選中的稱呼方式索引 @State @Watch('updateSelections') selectedCallMethodIndex: number[] = [0]; // 使用者輸入的關係描述 @State @Watch('updateSelections') userInput: string = ""; // 計算結果顯示 @State calculationResult: string = ""; // 輸入框是否獲得焦點 @State isInputFocused: boolean = false; // 當選擇發生改變時,更新關係計算 updateSelections() { // 根據索引獲取選中的性別(0為男,1為女) const gender = this.selectedGenderIndex[0] === 0 ? 1 : 0; // 判斷是否需要反轉稱呼方向 const reverse = this.selectedCallMethodIndex[0] === 0 ? false : true; // 呼叫關係計算模組進行計算 const result: string[] = relationship({ text: this.userInput, reverse: reverse, sex: gender }) as string[]; // 如果有計算結果,則更新顯示;否則顯示預設提示 if (result && result.length > 0) { this.calculationResult = `${reverse ? '對方稱呼我' : '我稱呼對方'}:${result[0]}`; } else { this.calculationResult = this.userInput ? '當前資訊未查到關係' : ''; } } // 元件即將顯示時,初始化性別和稱呼方式選擇按鈕的配置 aboutToAppear(): void { this.genderButtonOptions = SegmentButtonOptions.capsule({ buttons: this.genderOptions as SegmentButtonItemTuple, multiply: false, fontColor: Color.White, selectedFontColor: Color.White, selectedBackgroundColor: this.themeColor, backgroundColor: this.lineColor, backgroundBlurStyle: BlurStyle.BACKGROUND_THICK }); this.callMethodButtonOptions = SegmentButtonOptions.capsule({ buttons: this.callMethodOptions as SegmentButtonItemTuple, multiply: false, fontColor: Color.White, selectedFontColor: Color.White, selectedBackgroundColor: this.themeColor, backgroundColor: this.lineColor, backgroundBlurStyle: BlurStyle.BACKGROUND_THICK }); } // 構建元件介面 build() { // 建立主列布局 Column() { // 標題欄 Text('親戚關係計算器') .fontColor(this.textColor) .fontSize(18) .width('100%') .height(50) .textAlign(TextAlign.Center) .backgroundColor(Color.White) .shadow({ radius: 2, color: this.lineColor, offsetX: 0, offsetY: 5 }); // 內部列布局 Column() { // 性別選擇行 Row() { Text('我的性別').fontColor(this.textColor).fontSize(18); // 性別選擇按鈕 SegmentButton({ options: this.genderButtonOptions, selectedIndexes: this.selectedGenderIndex }).width('400lpx'); }.height(45).justifyContent(FlexAlign.SpaceBetween).width('100%'); // 稱呼方式選擇行 Row() { Text('稱呼方式').fontColor(this.textColor).fontSize(18); // 稱呼方式選擇按鈕 SegmentButton({ options: this.callMethodButtonOptions, selectedIndexes: this.selectedCallMethodIndex }).width('400lpx'); }.height(45).justifyContent(FlexAlign.SpaceBetween).width('100%'); // 示例與清空按鈕行 Row() { // 示例按鈕 Text('示例') .fontColor("#5871ce") .fontSize(18) .padding(`${this.paddingBase / 2}lpx`) .backgroundColor("#f2f1fd") .borderRadius(5) .clickEffect({ level: ClickEffectLevel.LIGHT, scale: 0.8 }) .onClick(() => { this.userInput = this.userInputRelation; }); // 空白間隔 Blank(); // 清空按鈕 Text('清空') .fontColor("#e48742") .fontSize(18) .padding(`${this.paddingBase / 2}lpx`) .clickEffect({ level: ClickEffectLevel.LIGHT, scale: 0.8 }) .backgroundColor("#ffefe6") .borderRadius(5) .onClick(() => { this.userInput = ""; }); }.height(45) .justifyContent(FlexAlign.SpaceBetween) .width('100%'); // 使用者輸入框 TextInput({ text: $$this.userInput, placeholder: !this.isInputFocused ? `請輸入稱呼。如:${this.userInputRelation}` : '' }) .placeholderColor(this.isInputFocused ? this.themeColor : Color.Gray) .fontColor(this.isInputFocused ? this.themeColor : this.textColor) .borderColor(this.isInputFocused ? this.themeColor : Color.Gray) .caretColor(this.themeColor) .borderWidth(1) .borderRadius(10) .onBlur(() => this.isInputFocused = false) .onFocus(() => this.isInputFocused = true) .height(45) .width('100%') .margin({ top: `${this.paddingBase / 2}lpx` }); } .alignItems(HorizontalAlign.Start) .width('650lpx') .padding(`${this.paddingBase}lpx`) .margin({ top: `${this.paddingBase}lpx` }) .borderRadius(10) .backgroundColor(Color.White) .shadow({ radius: 10, color: this.lineColor, offsetX: 0, offsetY: 0 }); // 結果顯示區 Column() { Row() { // 顯示計算結果 Text(this.calculationResult).fontColor(this.textColor).fontSize(18); }.justifyContent(FlexAlign.SpaceBetween).width('100%'); } .visibility(this.calculationResult ? Visibility.Visible : Visibility.None) .alignItems(HorizontalAlign.Start) .width('650lpx') .padding(`${this.paddingBase}lpx`) .margin({ top: `${this.paddingBase}lpx` }) .borderRadius(10) .backgroundColor(Color.White) .shadow({ radius: 10, color: this.lineColor, offsetX: 0, offsetY: 0 }); } .height('100%') .width('100%') .backgroundColor("#f4f8fb"); } }