計算機二級python指導用書程式設計題答案
個人手寫,歡迎指正
更新中。。。
#!/user/bin/env python
#-*- coding:utf-8 -*-
#author:M10
import random
#3-1輸入整數,輸出百分位及以上的數字
'''
s = input("輸入整數哦")
print(s[:-2])
'''
#3-2獲取輸入字串,按照空格分割,按行列印
'''
i = input("輸入字串")
s = i.split()
for j in s:
print(j)
'''
#3-3輸入數字1-7,輸出星期幾
"""
i = int(input('input a number between 1-7:'))
if i == 1:
print('monday')
elif i == 2:
print('tuesday')
elif i == 3:
print('wednesday')
elif i == 4:
print('thursday')#後續省略
else:
print('sunday')
"""
#3-4迴文數,正反排列相同的數
"""
s = input("input a 5-num")
if s[0]==s[-1] and s[1]==s[-2]:
print('迴文')
else:
print('不是迴文')
"""
#3-5輸入十進位制整數,分別輸出二進位制、八進位制、十六進位制字串
"""
s = int(input('a number:'))
print('二進位制:{:b}'.format(s))
print('八進位制:{:o}'.format(s))
print('十六進位制:{:x}'.format(s))
"""
#4-1輸入一個年份,判斷是否是閏年
"""
i = int(input('input a year:'))
if (i%4==0 and i%100 != 0) or i%400==0 :
print(i,'閏年')
else:
print('不是閏年')
"""
#4-2最大公約數與最小公倍數
"""
a,b = map(int,input('輸入兩個數,逗號分割:').split(','))
x = a*b
if a>b:
while True:
s = a%b
if s ==0:
print('最大公約數:',b)
print('最小公倍數:',int(x/b))
break
b,a = s,b
elif a<b:
while True:
s = b%a
if s ==0:
print('最大公約數:',a)
print('最小公倍數',int(x/a))
break
a,b = s,a
else:
print('最大公約數:',a)#兩數相等
print('最小公倍數',int(x/a))
"""
#4-3統計不同字元個數
"""
z,s,k,q = 0,0,0,0
str = input('輸入字元:')
l = len(str)
for i in range(l):
if '0' <= str[i] <='9':
z += 1
elif 'A'<=str[i]<='z':
s += 1
elif str[i] == ' ':
k +=1
else:q+=1
print('整數',z)
print('英文字元',s)
print('空格',k)
print('其他',q)
"""
#4-4猜數有戲續
"""
ran = random.randint(1,1000)
count=0
while True:
try:
i = eval(input('pls input a num:'))
except:
print('型別錯誤,重新輸入')
continue
if type(i) != type(1):
print('型別錯誤,重新')
continue
else:
count += 1
if i >ran:
print("it's bigger,{}次".format(count))
elif i<ran:
print("it's smaller,{}次".format(count))
else:
print("Right,{}次".format(count))
break
"""
#4-5羊車門問題
#十萬次隨機實驗,不換猜到車的次數為count_n,交換猜到車次數為count
"""
j=1
count_n = 0
count = 0
while j<=100000:
j +=1
i= random.randint(0,2)#隨機猜一個數字,0車,12羊
#若猜對車則不換+1,若猜對羊則換+1
if i == 0:
count_n +=1
else:
count +=1
print("don't change",count_n/100000)
print("change",count/100000)
"""
#5-1實現isNum函式
"""
def isNum(s):
if type(s)==type(1+1j) or type(s)==type(1) or type(s)==type(1.1):
return 1
else:
return 0
"""
#5-2實現isPrime函式
"""
def isPrime(a):
try:
if a%1 == 0 and a%2!=0 and a%3 !=0 and a%5 !=0 and a%7!=0:
return True
else:
return False
except:
print('pls input a intger')
print(isPrime(123))
"""
#5-3記數函式
"""
def count(s):
l = len(s)
count_int,count_str,count_sp,count_or = 0,0,0,0
for i in range(l):
if '0'<=s[i]<='9':
count_int +=1
elif 'A'<=s[i]<='z':
count_str +=1
elif s[i]==' ':
count_sp +=1
else:
count_or +=1
print('數字、字母、空格、其他分別為:',count_int,count_str,count_sp,count_or)
count('1232h1 2huuusnu2 u1ue2ue #$ ')
"""
#5-4列印200以內所有素數,空格分開
"""
#b記錄整除次數,只能被1和自身整除的是質數
def print_prime():
for j in range(1,201):
b = 0
if j == 1 :
print(1,end=' ')
else:
for i in range(2,j+1):
if j%i ==0:
b += 1
if b<=1:
print(j,end=' ')
print_prime()
"""
#5-5引數為n,獲取斐波那契數列第n個數並返回
"""
def Fibonacci(n):
a,b=1,1
i=1
while i<n:
b,a = a+b,b
i +=1
print(a)
Fibonacci(9)
"""
#6-1英文字元頻率統計
"""
s = 'ahduh aidhiufhbfuh adnuasdnba'
d={}
for i in s:
if 'a'<=i<='z':
d[i]=d.get(i,0)+1
s = sorted(d,reverse=True)
for i in s:
print(i,d[i])
"""
#6-2中文字元頻率統計
"""
s = 'hsua uhduahu阿虎毒啊 阿好速度啊哈shauhdia a d i2 213231huwqusn1 我是'
d = {}
for i in s:
d[i]=d.get(i,0)+1
s = sorted(d,reverse=True)
for i in s:
print(i,d[i])
"""
#6-3隨機密碼的生成
"""
import string
d = string.digits # 輸出包含數字0~9的字串
lo = string.ascii_lowercase # 包含所有小寫字母的字串
up = string.ascii_uppercase # 包含所有大寫字母的字串
list = list(d)+list(lo)+list(up)
n = len(list)
st=''
for j in range(0,10):
for i in range(0,8):
s = random.randint(0,n-1)
st=st+str(list[s])
print(st)
st = ''
"""
#6-4重複元素的判定
"""
def judge_list(list):
dic = {}
for i in list:
dic[i]=dic.get(i,0)+1
if max(dic.values())>1:
print('pass')
return True
else:
print('no pass')
list=[1,2,3,4,5,6,7,7,7,8,9]
judge_list(list)
list=[1,2,3,4,5,6,7,10,8,123,9]
judge_list(list)
"""
#6-5上題續
"""
def judge_list(list):
l1 = len(list)
s=len(set(list))
if l1==s:
print('pass')
return True
else:
print('no pass')
list1=[1,2,3,4,5,6,7,7,7,8,9]
judge_list(list1)
list=[1,2,3,4,5,6,7,10,8,123,9]
judge_list(list)
"""
#7-1輸入一個檔案和字元,統計該字元在檔案中出現的次數
txt = input('input a txtpath:')
str = input('input a str:')
s=0
f = open(txt,'r')
text = f.read()
for i in text:
if i==str:
s +=1
print(str,'has',s,'in txt')
"""
測試結果如下
input a txtpath:/Users/wangxingfan/Desktop/1.txt
input a strpath:s
s has 402 in txt
"""
#7-2英文檔案,大小寫字母互換
path = '/Users/wangxingfan/Desktop/1.txt'
f = open(path,'r')
str = f.read()
j=''
for i in str:
if i<'a':
s = i.lower()
j=j+s
elif i>='a':
s = i.upper()
j=j+s
print(j)
print('-------------')
print(str)
f.close()
"""
測試結果如下
aabuhyybnuhybuyyBUAYDABNubqdywubYBYBQWYBDYQBdqbdlhqbdBUBBDEQBSQHUKJBDqbwDHQ,
DNuQDbbqdubhqd,
DnqnwdqdDNQDNQI,
DQWNDQUJDNUndnjwndj
-------------
AABUHYYBNUHYBUYYbuaydabnUBQDYWUBybybqwybdyqbDQBDLHQBDbubbdeqbsqhukjbdQBWdhq,
dnUqdBBQDUBHQD,
dNQNWDQDdnqdnqi,
dqwndqujdnuNDNJWNDJ
"""
#7-3隨機生成10*10矩陣並儲存csv
import string
import numpy as np
path = '/Users/wangxingfan/Desktop/1.csv'
d = string.digits # 輸出包含數字0~9的字串
lo = string.ascii_lowercase # 包含所有小寫字母的字串
up = string.ascii_uppercase # 包含所有大寫字母的字串
list = list(d)+list(lo)+list(up)
for c in range(0,10):
j = np.random.randint(0, len(list), 10)
a = ''
for i in j:
s = list[i]+','
a = a+s
f = open(path,'a')
f.write(a+'\n')
f.close()
#檢視結果
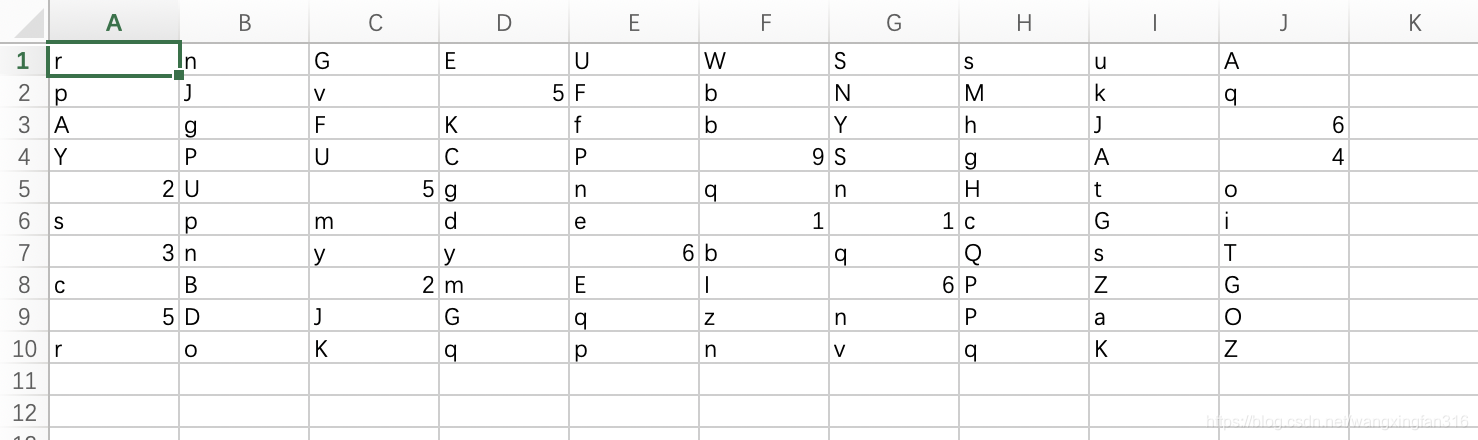
#7-4
相關文章
- Python 計算機二級模擬題賞析Python計算機
- 程式設計師如何備戰全國計算機二級(Python)考試?程式設計師計算機Python
- (計算機二級C語言)程式修改題<99>計算機C語言
- Java計算機二級(上機真題)Java計算機
- Python快速程式設計入門課後程式題答案Python程式設計
- 物件導向程式設計-java語言 第二週程式設計題物件程式設計Java
- 雲端計算面試題筆試錦集,雲端計算實用面試題答案二面試題筆試
- 2024 計算導論與程式設計程式設計
- 電腦科學和Python程式設計導論(一) 計算機相關理論Python程式設計計算機
- Java程式設計(2021春)——第二章課後題(選擇題+程式設計題)答案與詳解Java程式設計
- 【新聞】室溫超導體來了?可以在超級計算機上寫程式碼,用超級計算機打遊戲?計算機遊戲
- C語言程式設計實驗指導書 王明衍pdfC語言程式設計
- Epson機器人程式設計初級階(二)機器人程式設計
- Python物件導向程式設計Python物件程式設計
- Python 物件導向程式設計Python物件程式設計
- Contravariance 概念在計算機程式設計中的應用計算機程式設計
- 計算機二級Java複習1計算機Java
- Javascript 物件導向程式設計(二)JavaScript物件程式設計
- 全國計算機等級考試二級教程--C語言程式設計(2018年版) 隨手筆記(二)計算機C語言程式設計筆記
- 【計算機二級Python】考試攻略及資料彙總計算機Python
- 用python和計算機對話(計算機的語句)Python計算機
- FPB 2.0:免費的計算機程式設計類中文書籍 2.0計算機程式設計
- 程式設計師的計算機配置程式設計師計算機
- 計算機程式設計心得總結計算機程式設計
- 10 早期計算機如何程式設計計算機程式設計
- 電腦科學和Python程式設計導論(二 ) Python簡介Python程式設計
- 計算機導論計算機
- Python OOP 物件導向程式設計PythonOOP物件程式設計
- python技能--物件導向程式設計Python物件程式設計
- Python物件導向程式設計(1)Python物件程式設計
- Python - 物件導向程式設計 - super()Python物件程式設計
- Python - 物件導向程式設計 - @propertyPython物件程式設計
- 《計算機基礎與程式設計》第二週學習總結計算機程式設計
- 程式設計師面試常問計算機網路問題程式設計師面試計算機網路
- Python核心程式設計第2版第六章習題答案Python程式設計
- 月薪20+的程式設計師面試都問這些高階技術題(含答案+面試指導)程式設計師面試
- 雲端計算面試題筆試錦集,雲端計算實用面試題答案一面試題筆試
- 用Python開發實用程式 – 計算器Python