ALU實現
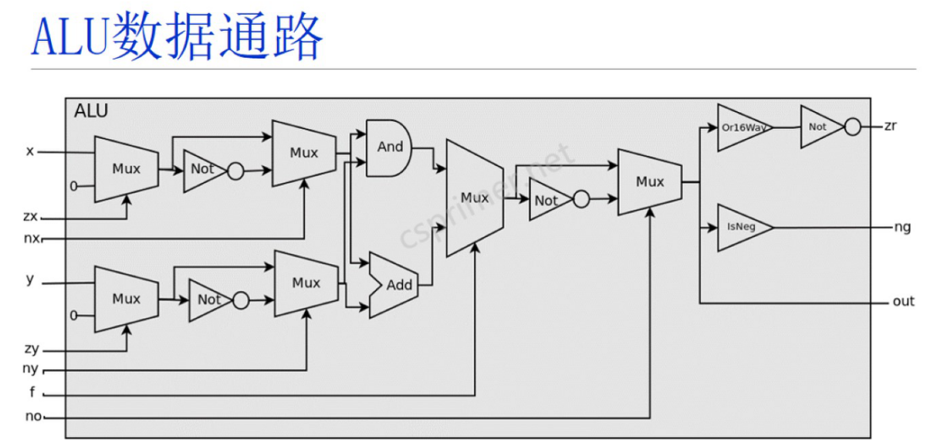
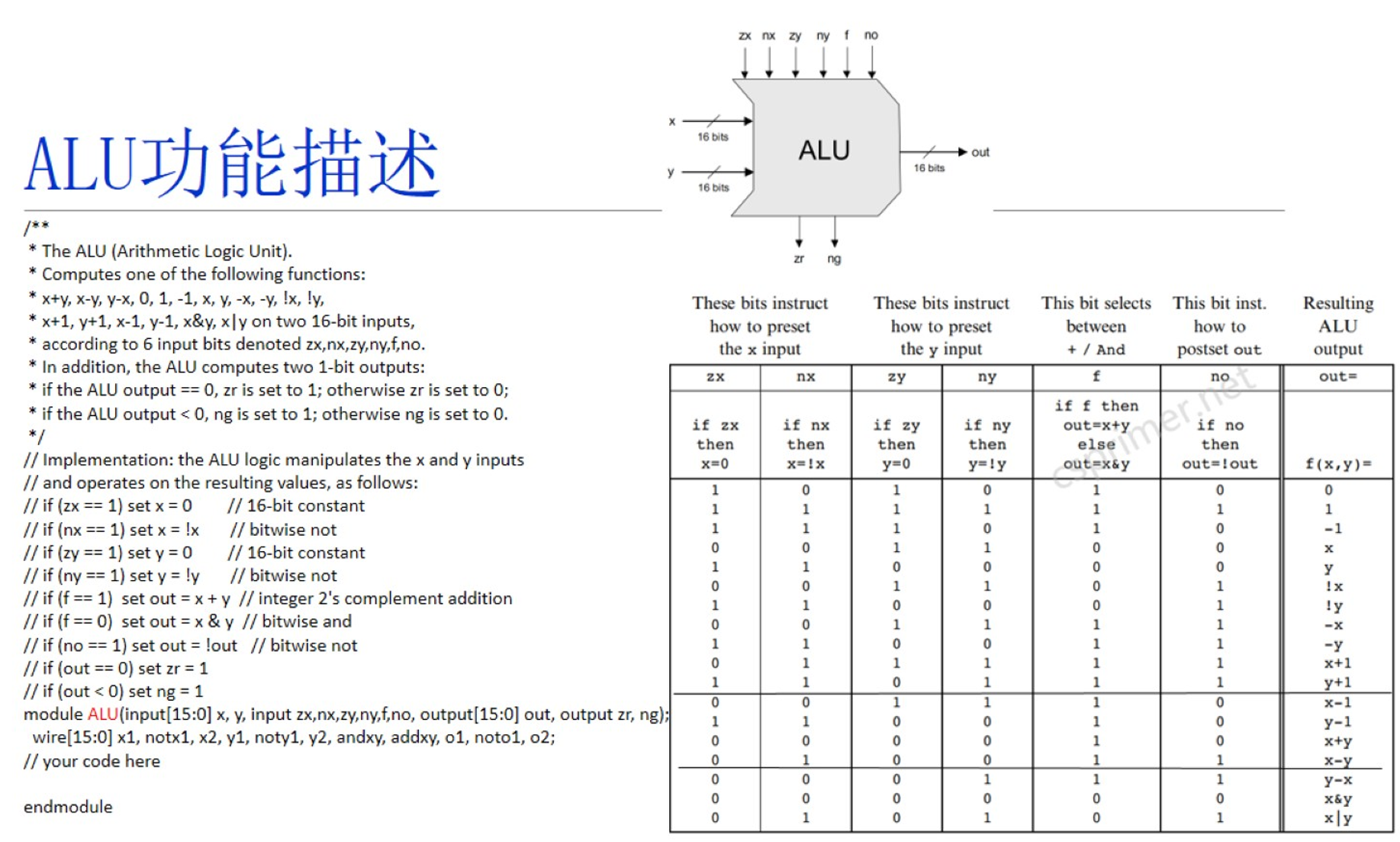
Or16way
/**
* 16-way Or:
* out = (in[0] or in[1] or ... or in[15])
*/
module Or16Way(input[15:0] in,output out);
// your code here
wire out1,out2,out3,out4,out5,out6,out7,out8,out9,out10,out11,out12,out13,out14;
Or g1(in[0],in[1],out1);
Or g2(out1,in[2],out2);
Or g3(out2,in[3],out3);
Or g4(out3,in[4],out4);
Or g5(out4,in[5],out5);
Or g6(out5,in[6],out6);
Or g7(out6,in[7],out7);
Or g8(out7,in[8],out8);
Or g9(out8,in[9],out9);
Or g10(out9,in[10],out10);
Or g11(out10,in[11],out11);
Or g12(out11,in[12],out12);
Or g13(out12,in[13],out13);
Or g14(out13,in[14],out14);
Or g15(out14,in[15],out);
endmodule
IsNeg
module IsNeg(input[15:0] in, output out);
// your code here
Or g1(in[15],1'b0,out);
endmodule
alu
/**
* The ALU (Arithmetic Logic Unit).
* Computes one of the following functions:
* x+y, x-y, y-x, 0, 1, -1, x, y, -x, -y, !x, !y,
* x+1, y+1, x-1, y-1, x&y, x|y on two 16-bit inputs,
* according to 6 input bits denoted zx,nx,zy,ny,f,no.
* In addition, the ALU computes two 1-bit outputs:
* if the ALU output == 0, zr is set to 1; otherwise zr is set to 0;
* if the ALU output < 0, ng is set to 1; otherwise ng is set to 0.
*/
// Implementation: the ALU logic manipulates the x and y inputs
// and operates on the resulting values, as follows:
// if (zx == 1) set x = 0 // 16-bit constant
// if (nx == 1) set x = !x // bitwise not
// if (zy == 1) set y = 0 // 16-bit constant
// if (ny == 1) set y = !y // bitwise not
// if (f == 1) set out = x + y // integer 2's complement addition
// if (f == 0) set out = x & y // bitwise and
// if (no == 1) set out = !out // bitwise not
// if (out == 0) set zr = 1
// if (out < 0) set ng = 1
module ALU(input[15:0] x, y, input zx,nx,zy,ny,f,no, output[15:0] out, output zr, ng);
wire[15:0] x1, notx1, x2, y1, noty1, y2, andxy, addxy, o1, noto1, o2;
wire notzr;
// your code here, use Mux16,Not16,Add16,And16,Or16Way,IsNeg to implement
// if (zx == 1) set x = 0
Mux16 g1(x,16'h0,zx,x1);
// if (nx == 1) set x = !x
Not16 g2(x1,notx1);
Mux16 g3(x1,notx1,nx,x2);
// if (zy == 1) set y = 0
Mux16 g4(y,16'h0,zy,y1);
// if (ny == 1) set y = !y
Not16 g5(y1,noty1);
Mux16 g6(y1,noty1,ny,y2);
// addxy = x + y
Add16 g7(x2,y2,addxy);
// andxy = x & y
And16 g8(x2,y2,andxy);
// if (f == 1) set out = x + y else set out = x & y
Mux16 g9(andxy,addxy,f,o1);
// if (no == 1) set out = !out
Not16 g10(o1,noto1);
Mux16 g11(o1,noto1,no,out);
// zr
Or16Way g12(out,notzr);
Not g13(notzr,zr);
// ng
IsNeg g14(out,ng);
endmodule