import os.path
from PyQt5 import QtWidgets
from PyQt5 import QtCore, QtGui
import sys
import cv2
class SpinBoxPanel(QtWidgets.QWidget):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
select_btn = QtWidgets.QPushButton("影像選擇")
self.path_label = QtWidgets.QLabel()
self.path_label.setText("當前未顯示影像路徑")
self.path_label.setAlignment(QtCore.Qt.AlignCenter) # label上居中顯示
self.path_label.setMaximumHeight(50) # label最大高度設定
self.path_label.setStyleSheet("background-color:pink;color:green") # 背景顏色設定
font = QtGui.QFont()
font.setBold(True)
font.setPointSizeF(10)
self.path_label.setFont(font)
self.image_label = QtWidgets.QLabel()
# 方法二:使用cv2顯示
src = cv2.imread("./image/img1.png") # BGR
image = cv2.cvtColor(src, cv2.COLOR_BGR2RGB) # 將BGR轉為RGB
h, w, c = image.shape
img = QtGui.QImage(image.data, w, h, 3 * w, QtGui.QImage.Format_RGB888)
pixmap = QtGui.QPixmap(img)
pix = pixmap.scaled(QtCore.QSize(640, 640), QtCore.Qt.KeepAspectRatio) # 自動保持比例放縮方式
self.image_label.setPixmap(pix) # 設定影像顯示
self.image_label.setAlignment(QtCore.Qt.AlignCenter) # label上的內容居中顯示
self.image_label.setStyleSheet("background-color:blue;color:green") # 背景顏色設定
self.win_spinbox = QtWidgets.QSpinBox()
self.win_spinbox.setRange(0, 100)
self.win_spinbox.setSingleStep(1)
self.win_spinbox.setValue(0)
self.sigma_spinbox = QtWidgets.QSpinBox()
self.sigma_spinbox.setRange(0, 100)
self.sigma_spinbox.setSingleStep(1)
self.sigma_spinbox.setValue(15)
paramPanel = QtWidgets.QGroupBox("引數設定")
hbox = QtWidgets.QHBoxLayout()
hbox.addWidget(QtWidgets.QLabel("視窗:"))
hbox.addWidget(self.win_spinbox)
hbox.addWidget(QtWidgets.QLabel("方差:"))
hbox.addWidget(self.sigma_spinbox)
# hbox.addStretch(1)
paramPanel.setLayout(hbox)
self.combox = QtWidgets.QComboBox()
self.combox.addItem("原圖")
self.combox.addItem("高斯模糊")
comboxPanel = QtWidgets.QGroupBox("方法選擇")
hbox2 = QtWidgets.QHBoxLayout()
hbox2.addWidget(self.combox)
comboxPanel.setLayout(hbox2)
btn_panel = QtWidgets.QGroupBox("影像檔案選擇")
hboxlayout = QtWidgets.QHBoxLayout()
hboxlayout.addWidget(self.path_label)
hboxlayout.addWidget(select_btn)
hboxlayout.addStretch(1)
btn_panel.setLayout(hboxlayout)
self.image_label.setAlignment(QtCore.Qt.AlignCenter) # label上的內容居中顯示
self.image_label.setStyleSheet("background-color:pink;color:green") # 背景顏色設定
panel1 = QtWidgets.QWidget()
hbox3 = QtWidgets.QHBoxLayout()
hbox3.addWidget(comboxPanel)
# hbox3.addStretch(1)
hbox3.addWidget(paramPanel)
hbox3.addStretch(1)
panel1.setLayout(hbox3)
vboxlayout = QtWidgets.QVBoxLayout()
vboxlayout.addWidget(panel1)
vboxlayout.addWidget(self.image_label)
vboxlayout.addWidget(btn_panel)
vboxlayout.addStretch(1)
self.setLayout(vboxlayout)
# 繫結點選
select_btn.clicked.connect(self.on_select_image)
self.win_spinbox.valueChanged.connect(self.on_blur_image)
self.sigma_spinbox.valueChanged.connect(self.on_blur_image)
self.combox.currentIndexChanged.connect(self.on_select_change)
def on_select_change(self):
if self.combox.currentIndex() == 1:
# 做高斯模糊處理
self.on_blur_image()
else:
# 顯示原圖
fileName = self.path_label.text()
pixmap = QtGui.QPixmap(fileName)
pix = pixmap.scaled(QtCore.QSize(640, 640), QtCore.Qt.KeepAspectRatio) # 自動保持比例放縮方式
self.image_label.setPixmap(pix) # 設定影像顯示
def on_blur_image(self):
file_path = self.path_label.text()
file_path = os.path.join('.\\image', file_path.split('/')[-1])
src = cv2.imread(file_path) # BGR
win_size = self.win_spinbox.value() * 2 + 1 # 需要是奇數
sigma = self.sigma_spinbox.value()
blur = cv2.GaussianBlur(src, (win_size, win_size), sigma) # 將BGR轉為gray
rbg = cv2.cvtColor(blur, cv2.COLOR_BGR2RGB) # 將BGR轉為RGB
h, w, c = src.shape
img = QtGui.QImage(rbg.data, w, h, 3 * w, QtGui.QImage.Format_RGB888)
pixmap = QtGui.QPixmap(img)
pix = pixmap.scaled(QtCore.QSize(640, 640), QtCore.Qt.KeepAspectRatio) # 自動保持比例放縮方式
self.image_label.setPixmap(pix) # 設定影像顯示
# self.image_label.setAlignment(QtCore.Qt.AlignCenter) # label上的內容居中顯示
# self.image_label.setStyleSheet("background-color:blue;color:green") # 背景顏色設定
def on_select_image(self):
fileinfo = QtWidgets.QFileDialog.getOpenFileName(self, "開啟影像檔案", ".", "影像檔案(*.jpg *.png)")
fileName = fileinfo[0]
if fileName != "":
self.path_label.setText(fileName)
pixmap = QtGui.QPixmap(fileName)
pix = pixmap.scaled(QtCore.QSize(640, 640), QtCore.Qt.KeepAspectRatio) # 自動保持比例放縮方式
self.image_label.setPixmap(pix) # 設定影像顯示
# def on_txt_chkbox(self):
# pass
#
# def on_wm_chkbox(self):
# pass
#
# def on_time_chkbox(self):
# pass
if __name__ == '__main__':
app = QtWidgets.QApplication(sys.argv)
main_win = QtWidgets.QMainWindow()
main_win.setWindowTitle("計數調節器")
myPanel = SpinBoxPanel()
main_win.setCentralWidget(myPanel)
main_win.setMinimumSize(1080, 720)
main_win.show()
app.exec_()
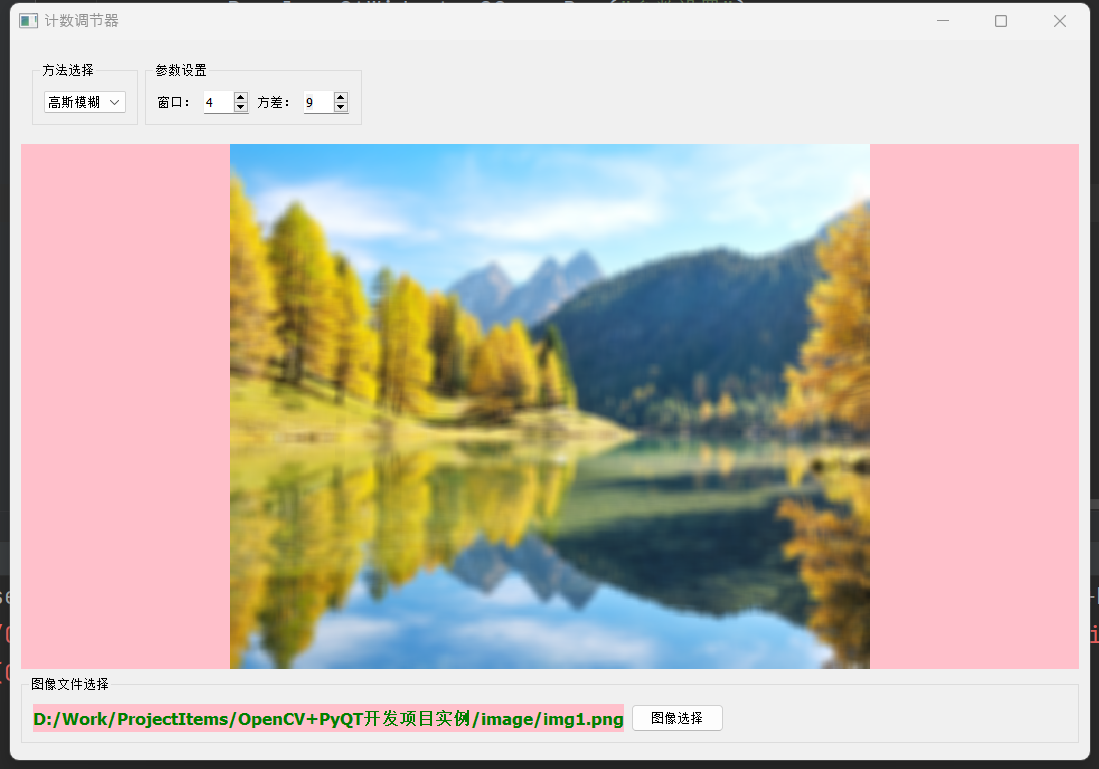