Android 使用graphics.Camera類實現自定義旋轉飄落
俺是一名小菜鳥,這是俺第一篇部落格。在文中有引用個別前輩大神的部落格。如有侵權請告知,俺會盡快處理。
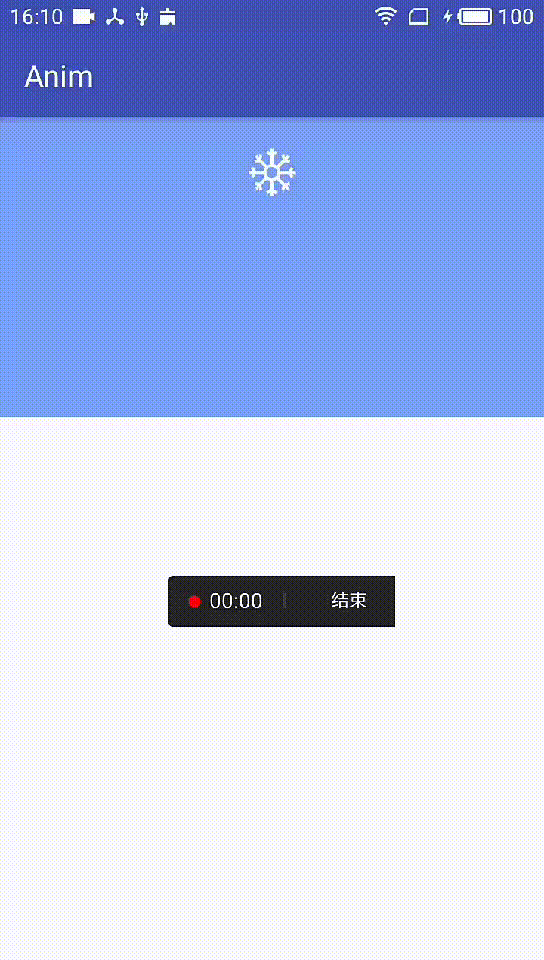
需求:
前段時間有個需求:做一個類似跑酷的綵帶散落動畫效果。
查資料:
按照初始想法+同事模糊建議,先在網上收羅了一堆雪花飄落效果。發現並不滿足需求,網上大多數案例都是平面的飄落效果。缺少一點靈動(旋轉)。在一個查詢資料裡的偶然中發現一個好東西:使用Camera實現3D旋轉飄落的效果部落格:模仿荷包啟動動畫點選開啟連結。發現一個之前從未用過的好東西graphics.Camera類。Camera可以實現普通雪花飄落沒有的旋轉效果,該的具體介紹及使用就不做格外介紹了。需要詳細瞭解的朋友們自行找一下度娘了。
想法:
最初想法將Camera的旋轉效果合成到網上已有的雪花飄落專案即可。因為兩個專案都不是自己寫的遇到一些列的困難。最後選擇了一步一步的,自己用自己掌握的自定義view去實現。
1.寫一個FallView 繼承View,實現單個雪花圖片旋轉飄落。
2.寫一個SnowFallView繼承RelativeLayout用於存放List<FallView>以實現多個雪花圖片飄落效果。
實現:
注:由於俺的水平有限,無暫未考慮效能優化的深入問題。僅求實現效果。
因為時間問題、程式碼成熟度不夠好等原因,本文只講第1點FallView的實現,直接上程式碼,裡面有詳細註釋
package com.walke.anim.camera;
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.Camera;
import android.graphics.Canvas;
import android.graphics.Matrix;
import android.graphics.Paint;
import android.support.annotation.Nullable;
import android.util.AttributeSet;
import android.view.View;
import com.walke.anim.R;
/**
* Created by walke.Z on 2018/4/20.
* 雪花飄落效果:
* 1,單個飄落效果
* a.onDraw 使用 canvas.drawBitmap()方法繪製雪花
* b.改變繪製位置實現動畫效果
*/
public class FallView extends View {
private Bitmap mSnow;
/**
* 控制元件寬度
*/
private int mWidth;
/**
* 控制元件高度
*/
private int mHeight;
/**
* 畫筆
*/
private Paint mPaint;
/**
*
*/
private float mX1;
/**
*
*/
private float mY1 = 0;
/**
* graphics 包中的類,用於實現旋轉
*/
Camera mCamera;
/**
* 飄落速度
*/
private float mSpeed = 1.5f;
public FallView(Context context) {
this(context, null);
}
public FallView(Context context, @Nullable AttributeSet attrs) {
this(context, attrs, 0);
}
public FallView(Context context, @Nullable AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
mSnow = BitmapFactory.decodeResource(getContext().getResources(), R.mipmap.image_snow_96);
mPaint = new Paint();
mCamera = new Camera();
}
private void resizeBitmap(float scale) {
Matrix m = new Matrix();
m.setScale(scale, scale);
mSnow = Bitmap.createBitmap(mSnow, 0, 0, mSnow.getWidth(), mSnow.getHeight(), m, true);
}
@Override
protected void onSizeChanged(int w, int h, int oldw, int oldh) {
super.onSizeChanged(w, h, oldw, oldh);
mWidth = w;
mHeight = h;
mX1 = (mWidth - mSnow.getWidth()) / 2;
}
private float degrees = 0;
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
//使用 graphics.Camera -------
Matrix mMatrix = new Matrix();
mCamera.save(); // 記錄一下初始狀態。save()和restore()可以將影像過渡得柔和一些。
//旋轉角度
if (degrees < 360) {
degrees += 6;
} else {
degrees = 0;
}
mCamera.rotateY(degrees);
// 平移--下落
if (mY1 > -(mHeight - mSnow.getHeight())) {
mY1 -= mSpeed;//注意這裡是 -
}
mCamera.translate(mX1, mY1, 0);// mY1: -50
mCamera.getMatrix(mMatrix);
int centerX = (mWidth) / 2;
int centerY = mSnow.getHeight() / 2;
mMatrix.preTranslate(-centerX, -centerY);
mMatrix.postTranslate(centerX, centerY);
mCamera.restore();
// Log.i("walke", "FallView draw: -------> mX1 = "+mX1+" ---> mY1 = "+mY1 );
canvas.drawBitmap(mSnow, mMatrix, mPaint);// 畫圖由左上角開始
// 普通圖片向下應用用以下兩行程式碼即可
// canvas.drawBitmap(mSnow, mX1, mY1, mPaint);// 畫圖由左上角開始
// mY1+=2;
invalidate();//60幀每秒
}
}
佈局使用:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<com.walke.anim.camera.FallView
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#79a1f7"/>
</LinearLayout>
效果圖片截圖,沒弄gif圖
分享一個線上mp4轉git的連結:
相關文章
- Android自定義View——從零開始實現雪花飄落效果AndroidView
- canvas實現的雪花飄落效果Canvas
- Android SeekBar 自定義thumb,thumb旋轉動畫效果Android動畫
- Android自定義View——從零開始實現可暫停的旋轉動畫效果AndroidView動畫
- 自定義實現Complex類
- Android自定義拍照實現Android
- Android 實現自定義圓環Android
- canvas實現的雪花飄落效果程式碼例項Canvas
- 基於HTML5+Webkit實現樹葉飄落動畫HTMLWebKit動畫
- 短視訊平臺搭建,Android自定義旋轉進度條Android
- Android中水波紋使用之自定義檢視實現Android
- Android程式碼實現自定義ButtonAndroid
- (轉)Android 自定義Dialog實現步驟及封裝Android封裝
- 【JAVA】自定義類載入器實現類隔離Java
- 由旋轉畫廊,看自定義RecyclerView.LayoutManagerView
- C#自定義控制元件—旋轉按鈕C#控制元件
- <轉>cocos2d-x學習筆記(五)模擬樹葉飄落效果的實現(精靈旋轉、翻轉、鐘擺運動等綜合運用)筆記
- QT 自定義QGraphicsItem 縮放後旋轉 圖形出現漂移問題QT
- SpringBoot應用使用自定義的ApplicationContext實現類Spring BootAPPContext
- 部落格園美化-隨季節變化實現不同的飄落效果
- 巧用fastjson自定義序列化類實現欄位的轉換ASTJSON
- Android 自定義圓形旋轉進度條,仿微博頭像載入效果Android
- | / - 的旋轉效果實現(轉)
- Android自定義控制元件之自定義ViewGroup實現標籤雲Android控制元件View
- 自定義View:自定義屬性(自定義按鈕實現)View
- 自定義圓環,跟隨手指旋轉角度加減layer
- Android 自定義實現switch開關按鈕Android
- Android自定義View實現文字輪播效果AndroidView
- Android自定義view實現數字時鐘AndroidView
- Elasticsearch實現自定義排序外掛(轉載)Elasticsearch排序
- iOS使用自定義URL實現控制器之間的跳轉iOS
- 一行程式碼實現自定義轉場動畫--iOS自定義轉場動畫集行程動畫iOS
- Android自定義幸運轉盤Android
- Android 自定義 View 實現橫行時間軸AndroidView
- Android自定義View:快遞時間軸實現AndroidView
- Android自定義View實現微信打飛機遊戲AndroidView遊戲
- php實現圖片旋轉PHP
- 自定義View實現箭頭沿圓轉動View