linux下使用mysql的C語言API
系統環境Ubuntu 12.04
1、安裝mysql
ubuntu下安裝mysql是比較簡單的,直接通過apt-get安裝

3、mysql的基本命令
在mysql中,輸入help或者?命令,即可用檢視mysql支援的內部操作命令。
(1)顯示資料庫列表
顯示資料庫列表命令比較簡單,直接輸入show databases;即可。
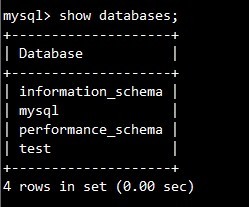
(2)選擇一個資料庫
選擇一個資料庫比較簡單,使用use dbname,其中dbname為要選擇的資料庫名字。比如,這裡我們選擇test資料庫:
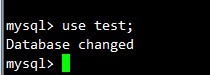
(3)檢視一個資料庫中的所有表
通過show tables,可以檢視一個資料庫中所有的資料庫表。
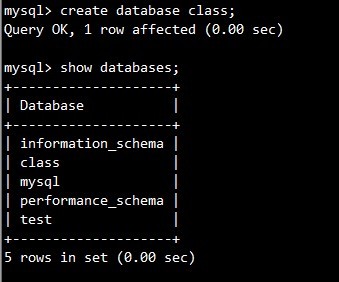
(2)建立學生資訊表

(3)插入基本資料
向已經建立好的資料表中插入3條基本記錄,SQL語句如下:
1、安裝mysql
ubuntu下安裝mysql是比較簡單的,直接通過apt-get安裝
- sudo apt-get install mysql-server-5.5
2、登入mysql
- mysql [-h host_name] [-u user_name] [-p password]
其中引數-h後面要給出連線的資料庫的IP地址或者域名、引數-u後面要給出登入的使用者名稱、引數-p表示登入的密碼。
有時連線本機預設的Mysql資料庫伺服器,則可以直接在命令列中輸入如下簡寫形式(root使用者):
之後,系統會提示你輸入root使用者的密碼
有時連線本機預設的Mysql資料庫伺服器,則可以直接在命令列中輸入如下簡寫形式(root使用者):
- mysql -u root -p

3、mysql的基本命令
在mysql中,輸入help或者?命令,即可用檢視mysql支援的內部操作命令。
(1)顯示資料庫列表
顯示資料庫列表命令比較簡單,直接輸入show databases;即可。
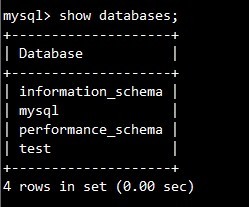
(2)選擇一個資料庫
選擇一個資料庫比較簡單,使用use dbname,其中dbname為要選擇的資料庫名字。比如,這裡我們選擇test資料庫:
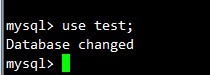
(3)檢視一個資料庫中的所有表
通過show tables,可以檢視一個資料庫中所有的資料庫表。
檢視Mysql表結構的命令,如下:
show columns from 表名;
describe 表名;
desc 表名;
show create table 表名;(檢視create指令碼)
(4)退出
簡單的命令,quit;
需要注意的是,每個命令後面需要加上分號“;”,因為分號表示一個事務的結束。
(5) mysqldump工具備份和恢復
幾個常用用例:
1.匯出整個資料庫
mysqldump -u 使用者名稱 -p 資料庫名 > 匯出的檔名
mysqldump -u wcnc -p smgp_apps_wcnc > wcnc.sql
2.匯出一個表
mysqldump -u 使用者名稱 -p 資料庫名 表名> 匯出的檔名
mysqldump -u wcnc -p smgp_apps_wcnc users> wcnc_users.sql
3.匯出一個資料庫結構
mysqldump -u wcnc -p -d --add-drop-table smgp_apps_wcnc >/home/dk/wcnc_db.sql
mysqldump -u 使用者名稱 -p 資料庫名 > 匯出的檔名
mysqldump -u wcnc -p smgp_apps_wcnc > wcnc.sql
2.匯出一個表
mysqldump -u 使用者名稱 -p 資料庫名 表名> 匯出的檔名
mysqldump -u wcnc -p smgp_apps_wcnc users> wcnc_users.sql
3.匯出一個資料庫結構
mysqldump -u wcnc -p -d --add-drop-table smgp_apps_wcnc >/home/dk/wcnc_db.sql
-d 沒有資料 --add-drop-table 在每個create語句之前增加一個drop table
4.匯入資料庫
常用source 命令
進入mysql資料庫控制檯,
如mysql -u root -p
mysql>use 資料庫
常用source 命令
進入mysql資料庫控制檯,
如mysql -u root -p
mysql>use 資料庫
然後使用source命令,後面引數為指令碼檔案(如這裡用到的.sql)
mysql>source /home/wcnc_db.sql
mysql>source /home/wcnc_db.sql
4、mysql資料庫操作例項。
(1)建立班級資料庫
建立資料庫的命令式create databases dbname,其中dbname為資料庫名,例如當前要建立的資料庫名為class,因此需要輸入下面的命令:
- mysq->create database class;
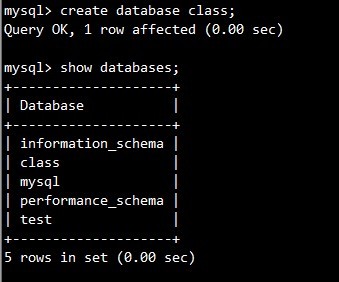
(2)建立學生資訊表
- mysql>use class;
- mysql>create table student (nid INT UNIQUE,name VARCHAR(20),age int);

(3)插入基本資料
- mysql->insert into student values(100, 'Lee', 16);
- mysql->insert into student values(101, 'Tom', 17);
- mysql->insert into student values(102, 'Harry', 15);
(4)查詢全部資料
利用select語句查詢全部記錄。
(5)刪除其中一條記錄
這裡我們假設Tom同學轉學了,那麼他已經不在我們的班級class裡面了,所以需要把他從class刪除。
mysql->delete from student where name = 'Tom';
(6)更新其中一條記錄
班級class中還存在一種情況,就是有學生要改名了,比如Lee要改名為Tony了。具體的sql語句如下:
mysql->update student set name = 'Tony' where name = 'Lee';
(7)刪除資料庫
如果學生畢業,那麼我們這個班級就沒有存在的必要了,這是就可以刪除掉這個資料庫class了。
刪除的步驟如下:
a、刪除所有的資料表,SQL語句為:
mysql->drop table class;
b、刪除資料庫,SQL語句為:
mysql->delete database class;
5、Mysql資料庫連線之C語言API
首先要安裝一個包libmysql++-dev包,不然編譯程式碼的時候會出現“mysql/mysql.h: No such file or directory”錯誤
sudo apt-get install libmysql++-dev
示例程式碼:
利用select語句查詢全部記錄。
- mysql->select * from student;
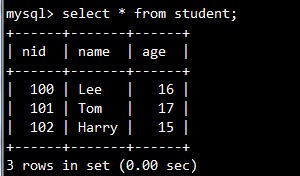
(5)刪除其中一條記錄
這裡我們假設Tom同學轉學了,那麼他已經不在我們的班級class裡面了,所以需要把他從class刪除。
mysql->delete from student where name = 'Tom';
(6)更新其中一條記錄
班級class中還存在一種情況,就是有學生要改名了,比如Lee要改名為Tony了。具體的sql語句如下:
mysql->update student set name = 'Tony' where name = 'Lee';
(7)刪除資料庫
如果學生畢業,那麼我們這個班級就沒有存在的必要了,這是就可以刪除掉這個資料庫class了。
刪除的步驟如下:
a、刪除所有的資料表,SQL語句為:
mysql->drop table class;
b、刪除資料庫,SQL語句為:
mysql->delete database class;
5、Mysql資料庫連線之C語言API
首先要安裝一個包libmysql++-dev包,不然編譯程式碼的時候會出現“mysql/mysql.h: No such file or directory”錯誤
sudo apt-get install libmysql++-dev
示例程式碼:
- #include <mysql.h>
#include <stdio.h>
int main()
{
MYSQL mysql; // need a instance to init
MYSQL_RES* res;
MYSQL_ROW row;
char* query;
int t, r;
// connect the database
mysql_init(&mysql);
if (!mysql_real_connect(&mysql, "localhost", "root", "123456", "test", 0, NULL, 0))
{
printf("Error connecting to database: %s\n", mysql_error(&mysql));
}
else
{
printf("Connected...\n");
}
// get the result from the executing select query
query = "SELECT * FROM TableName ORDER BY `id`";
t = mysql_real_query(&mysql, query, (unsigned int) strlen(query));
if (t)
{
printf("Error making query: %s\n", mysql_error(&mysql));
}
res = mysql_store_result(&mysql);
while (row = mysql_fetch_row(res))
{
for (t = 0; t < mysql_num_fields(res); t++)
{
printf("%s ", row[t]);
}
printf("\n");
}
mysql_free_result(res); //free result after you get the result
sleep(1);
// execute the insert query
query = "insert into TableName(id, name) values(2, 'kunp')";
t = mysql_real_query(&mysql, query, (unsigned int) strlen(query));
if (t)
{
printf("Error making query: %s\n", mysql_error(&mysql));
}
mysql_close(&mysql);
return 0;
}
編譯之~注意一定要先安裝libmysql++-dev包。編譯指令:gcc c_mysql.c -lmysqlclient -o c_mysql
或:
g++ -I/usr/include/mysql
-c c_mysql.c -o c_mysql.o
g++ -L/usr/lib/mysql -lmysqlclient c_mysql.o -o c_mysql
或64位系統g++ -L/usr/lib64/mysql lmysqlclient c_mysql.o -o c_mysql
執行./c_mysql
相關文章
- linux下c語言學習筆記——操作mysqlLinuxC語言筆記MySql
- Linux下C語言編譯的問題LinuxC語言編譯
- Linux下C語言驗證多程式LinuxC語言
- Linux下C語言程式設計(轉)LinuxC語言程式設計
- c語言巨集的使用C語言
- 【語言】Java 日期 API 的使用技巧JavaAPI
- Linux下跨語言呼叫C++實踐LinuxC++
- 【C語言】linux下多檔案編譯C語言Linux編譯
- linux下傳送email的c語言程式碼(轉)LinuxAIC語言
- java開發C語言編譯器:為C語言提供API呼叫JavaC語言編譯API
- C語言呼叫mysql資料庫API實現簡單的mysql客戶端的功能C語言MySql資料庫API客戶端
- Linux-C語言LinuxC語言
- c語言 5.9.2下載C語言
- Linux下C語言程式設計簡介(轉)LinuxC語言程式設計
- MySQL的C語言程式設計(一)MySqlC語言程式設計
- windows下ping程式使用C語言實現WindowsC語言
- Linux中的C語言妙用(轉)LinuxC語言
- C語言呼叫 Java(Linux)C語言JavaLinux
- Linux下關於時間概念的C語言程式設計LinuxC語言程式設計
- Linux下C語言程式設計基礎知識LinuxC語言程式設計
- C語言qsort函式的使用C語言函式
- C語言 檔案IO的使用C語言
- C語言MySQL程式設計示例C語言MySql程式設計
- Linux下C語言程式設計基礎知識(轉)LinuxC語言程式設計
- Swift中使用C語言的指標SwiftC語言指標
- C語言:記憶體使用C語言記憶體
- C語言 C語言野指標C語言指標
- C語言---“C語言 誰與爭鋒?”C語言
- linux下如何修改提示語言Linux
- 用C語言在Linux系統下建立守護程式(Daemon)C語言Linux
- Linux核心C語言將升級LinuxC語言
- c語言實現linux抓包C語言Linux
- C語言與嵌入式C語言的區別C語言
- C語言的本質(32)——C語言與彙編之C語言內聯彙編C語言
- Linuxcentos7/ubantu下:用C語言連線MySQL資料庫LinuxCentOSC語言MySql資料庫
- ARM下C語言棧幀機制C語言
- C語言的HelloWorldC語言
- go語言與c語言的相互呼叫GoC語言