小洋的python入門筆記
起因:發現自己前三年做的Python專案很多都是現做先學的,修改理解語法錯誤多依仗對C/C++的理解,對python缺乏一個系統的學習。趁此有空,補上!
特別鳴謝:B站找到的“Python課程推薦”UP主的1039.8萬播放量的課程《【2025】Python小白零基礎如何正確入門?這期影片手把手教你從零開始,入門到起飛!》;以及原作者Mosh Hamedani的課程《[Complete Python Mastery](Complete Python Mastery)》😃;還有Python 3的官方文件《[Python Documentation contents](Python Documentation contents — Python 3.13.0 documentation)》😸
執行環境:pycharm + python 3.8(conda自建的虛擬環境)
- 小洋的python入門筆記
- 1.輸出
- 2.變數
- 3.輸入接收
- 4.型號轉換
- 5.字串
- 6.格式化字串
- 7.字串方法
- 1)len函式
- 2)全部轉換為大寫/小寫方法
- 3)find方法
- 4)replace方法
- 5)in函式
- 8.算術運算
- 9.運算子優先順序
- 10.數學函式
- 11.if條件分支
- 12.邏輯運算子
- 13.比較運算子
- 14.重量轉換器
- 15.while迴圈
- 1)猜謎遊戲
- 2)汽車遊戲
- 16.for迴圈
- 17.巢狀迴圈
- 18.列表方法
- 19.二維列表
- 20.列表方法
- 21.元組
- 22.拆包
- 23.字典
1.輸出
print("He Yang")#裡面是單引號也可以!
輸出結果:
He Yang
2.變數
變數定義:
price = 10 #全域性變數,也是整數
rating = 4.9#float型浮點數
name = 'HeYang'#字串
is_published = False#bool型,注意python是大小寫敏感的
#尤其是bool變數對應的值,它是關鍵字,有“False”和“True”兩種型別
print(price)#10
練習:
age = 20
name = "John"
new_or_not = True
print(age,name,new_or_not)
輸出結果:
20 John True
3.輸入接收
name = input('What is your name? ')
print('Hi '+ name)#表示式:使用一個變數的程式碼
#上面這串程式碼連線了兩個字串
PS:注意上面的?後面加了一個“ ”,便於讓終端中我們的輸入不和問題挨在一起
輸出結果:
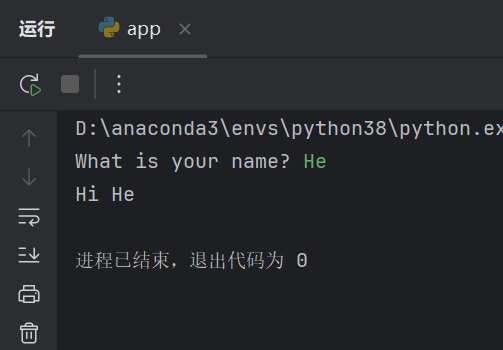
練習:
person_name = input("What's your name? ")
fav_color = input("What's your favourite color? ")
print(person_name +" likes "+fav_color)
輸出結果:
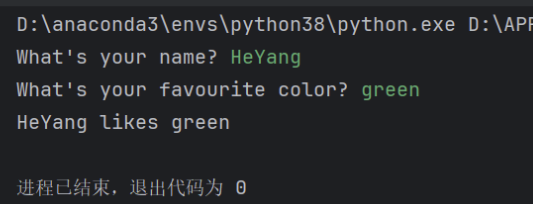
4.型號轉換
birth_year = input('Birth year: ')#注意birth_year是字串
print(type(birth_year))
age = 2019 - int(birth_year)
#下面是其他型別變數轉換為相應型別變數的函式
#int()
#float()
#bool()
print(type(age))
print(age)
練習:
pounds=input('weight: ')
kilograms=int(pounds)*0.45359237
print(kilograms)
print(type(kilograms))
輸出結果:
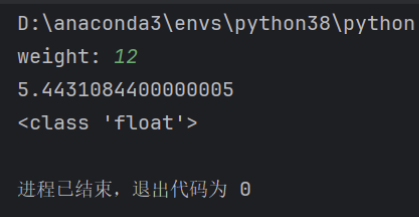
5.字串
#假如你想打出不換行的內部帶引號的內容
course = "Python's Course for Beginners"#內部只有單引號
print(course)#Python's Course for Beginners
course = 'Python for "Beginners"'
print(course)#Python for "Beginners"
#假如你想打內部跨越多行的
course='''
Hi John,
Here is our first email to you.
Thank you,
The support team
'''
print(course)
#
#Hi John,
#
#Here is our first email to you.
#
#Thank you,
#The support team
#
#
#
#python的索引
course = 'Python for Beginners'
print(course[0])#P
print(course[1])#y
print(course[-1])#s
print(course[-2])#r
print(course[0:3])#包含前,不包含後#Pyt
print(course[0:])#等價於print(course[0:字串的長度值])#Python for Beginners
print(course[1:])#ython for Beginners
print(course[:5])#等價於print(course[0:5])#Pytho
print(course[:])#Python for Beginners
another = course[:]#等價於複製
print(another)#Python for Beginners
name = 'Jennifer'
print(name[1:-1])#ennife
6.格式化字串
#用文字變數動態生成某些字串
#生成目標:John [Smith] is a coder
first = 'John'
last = 'Smith'
message = first + ' [' + last + '] is a coder'#不適用於複雜文字
print(message)
msg = f'{first} [{last}] is a coder'
print(msg)
輸出結果:
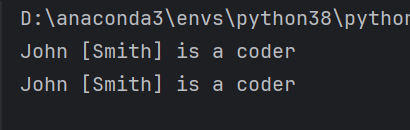
7.字串方法
1)len函式
course = 'Python for Beginner'
print(len(course))#len不僅僅用於字串,傳說還可以用於計算列表中專案的個數
#19
我們還有專門用於字串的函式
當一個函式針對某種特定的型別或物件,就是方法
下面的方法特定於字串,上面的函式len,print是通用方法
2)全部轉換為大寫/小寫方法
course.upper()不會改變原有字串,會創造一個新字串並返回它
course = 'Python for Beginner'
print(course.upper())#PYTHON FOR BEGINNER
print(course.lower())#python for beginner
print(course)#Python for Beginner
3)find方法
course = 'Python for Beginner'
print(course.find('P'))#會返回P在這個字串中第一次出現的索引#0
print(course.find('o'))#會返回o在這個字串中第一次出現的索引#4
#find方法是大小寫敏感的,如果找不到對應字母會返回-1
#比如找大寫O
print(course.find('O'))#-1
#我們還可以找字串
print(course.find('Beginner'))#返回11,因為Beginner是從第11個索引開始的#11
4)replace方法
course = 'Python for Beginner'
#我們還能替換字元/字串
print(course.replace('Beginner','Absolute Beginners'))
#Python for Absolute Beginners
#但是如果被替換的部分找不到,就會返回原字串,replace方法也是大小寫敏感的
print(course.replace('beginner','Absolute Beginners'))
#Python for Beginner
print(course.replace('P','J'))
#Jython for Beginner
5)in函式
course = 'Python for Beginner'
#當我們查詢原字串中是否包含某字串時,我們用in它會返回bool值
print('python' in course)
#False
8.算術運算
#算術運算
print(10+3)
print(10-3)
print(10*3)
print(10/3)#3.3333333333333335
print(10//3)#3
print(10%3)#1,表示取餘
print(10**3)#指數運算,1000
#增廣賦值運算子
x=10
x=x+2#等價於x+=2
x-=3#等價於x=x-3
print(x)#9
9.運算子優先順序
x=10+3*2**2
print(x)#22
#先是括號
#接著是指數 eg.2**3
#然後是乘法/除法
#最後是加減法
x=(2+3)*10-3
print(x)#47
10.數學函式
x=2.9
print(round(x))#四捨五入函式#3
print(abs(-2.9))#絕對值#2.9
import math
print(math.ceil(2.9))#上限函式#3
print(math.floor(2.9))#下限函式#2
round函式還可以指定四捨五入的位數,
# 四捨五入到小數點後兩位
print(round(3.14159, 2)) # 輸出: 3.14
所有數學函式 math — 數學函式 — Python 3.13.0 文件,如下:
數論和表示函式
函式 | 解釋 |
---|---|
ceil(x) | x 的上限,大於或等於 x 的最小整數 |
comb(n,k) | 從 n 個專案中選擇 k 個專案的方法數,不重複且無序 |
copysign(x,y) | x 的絕對值,帶有 y 的符號 |
fabs(x) | x 的絕對值 |
factorial(n) | n 的階乘 |
floor(x) | x 的下限,小於或等於 x 的最大整數 |
fma(x,y,z) | 融合乘加操作:(x*y)+z |
fmod(x,y) | x 除以 y 的餘數 |
frexp(x) | x 的尾數和指數 |
fsum(iterable) | 輸入可迭代物件中值的總和 |
gcd(*integers) | 整數引數的最大公約數 |
isclose(a,b, rel_tol, abs_tol) | 檢查 a 和 b 的值是否彼此接近 |
isfinite(x) | 檢查 x 是否既不是無窮大也不是 NaN |
isinf(x) | 檢查 x 是否是正無窮或負無窮大 |
isnan(x) | 檢查 x 是否是 NaN(不是一個數字) |
isqrt(n) | 非負整數 n 的整數平方根 |
lcm(*integers) | 整數引數的最小公倍數 |
ldexp(x,i) | x*(2**i),frexp() 函式的逆函式 |
modf(x) | x 的小數部分和整數部分 |
nextafter(x,y, steps) | 用於計算浮點數x 之後朝向y 的下一個浮點數 |
perm(n,k) | 從 n 個專案中選擇 k 個專案的方法數,不重複且有序 |
prod(iterable, start) | 輸入可迭代物件中元素的乘積,具有起始值 |
remainder(x,y) | x 相對於 y 的餘數 |
sumprod(p,q) | 兩個可迭代物件 p 和 q 的乘積之和 |
trunc(x) | x 的整數部分 |
ulp(x) | x 的最低有效位的值 |
冪函式和對數函式
函式 | 解釋 |
---|---|
cbrt(x) | x 的立方根 |
exp(x) | e 的 x 次冪 |
exp2(x) | 2 的 x 次冪 |
expm1(x) | e 的 x 次冪減去 1 |
log(x, base) | 以給定底數(預設為 e)計算 x 的對數 |
log1p(x) | 1+x 的自然對數(底數為 e) |
log2(x) | 以 2 為底數計算 x 的對數 |
log10(x) | 以 10 為底數計算 x 的對數 |
pow(x, y) | x 的 y 次冪 |
sqrt(x) | x 的平方根 |
三角函式
函式 | 解釋 |
---|---|
acos(x) | x 的反餘弦 |
asin(x) | x 的反正弦 |
atan(x) | x 的反正切 |
atan2(y, x) | atan(y / x) |
cos(x) | x 的餘弦 |
dist(p, q) | 兩點 p 和 q 之間的歐幾里得距離,給定為一個座標可迭代物件 |
hypot(*coordinates) | 一個座標可迭代物件的歐幾里得範數 |
sin(x) | x 的正弦 |
tan(x) | x 的正切 |
其他函式
函式分類 | 函式名稱 | 描述/定義 |
---|---|---|
角度轉換 | degrees(x) | 將角度x從弧度轉換為度數 |
radians(x) | 將角度x從度數轉換為弧度 | |
雙曲函式 | acosh(x) | x的反餘弦雙曲函式 |
asinh(x) | x反正弦雙曲函式 | |
atanh(x) | x反切線雙曲函式 | |
cosh(x) | x的雙曲餘弦函式 | |
sinh(x) | x的雙曲正弦函式 | |
tanh(x) | x的雙曲正切函式 | |
特殊函式 | erf(x) | x處的誤差函式 |
erfc(x) | x處的補誤差函式 | |
gamma(x) | x處的伽馬函式 | |
lgamma(x) | 伽馬函式在x處絕對值的自然對數 | |
常數 | pi | π = 3.141592... |
e | e = 2.718281... | |
tau | τ = 2π = 6.283185... | |
inf | 正無窮大 | |
nan | "Not a number" (NaN) |
11.if條件分支
is_hot = False
is_cold = True
if is_hot:
print("It's a hot day")
print("Drink plenty of water")
elif is_cold:
print("It's a cold day")
print("Wear warm clothes")
else:
print("It's a lovely day!")
print("Enjoy your day!")
輸出結果:
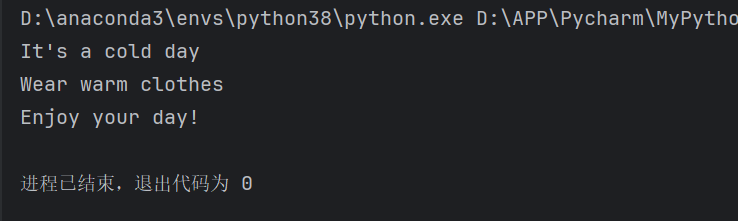
練習:
price=1000000#1M
good_credict = False
if good_credict:
down_payment = price * 0.1
print(price*0.1)
else:
down_payment = price * 0.2
print(price*0.2)
print(f"Down payment: ${down_payment}")#down_payment意為首付
輸出結果:
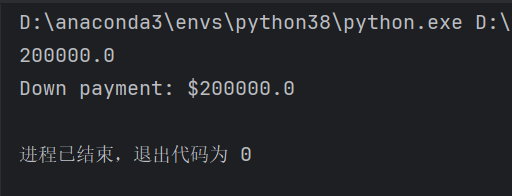
12.邏輯運算子
and or not
has_high_income = True
has_good_credict = True
if has_high_income and has_good_credict:#and:both
print("Eligible for loan")
if has_high_income or has_good_credict:#or:at least one
print("Eligible for loan 2")
has_criminal_record = True
if has_good_credict and not has_criminal_record:#not
print("Eligible for loan 3")
輸出結果:
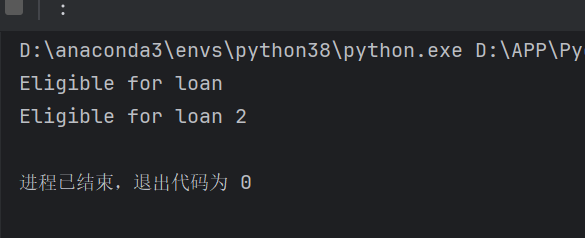
13.比較運算子
#比較運算子
temperature = 30
if temperature>30:#> < >= <= == != 沒有=,因為賦值不會生成bool值
print("It's a hot day")
else:
print("It's not a hot day")
輸出結果:
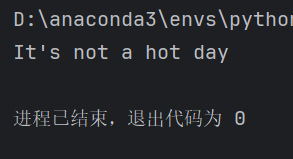
練習:
name = input("your name: ")
if len(name)<3:
print("name must be at least 3 characters")
elif len(name)>50:
print("name can be a maxinum of 50 characters")
else:
print("name looks good!")
輸出結果:
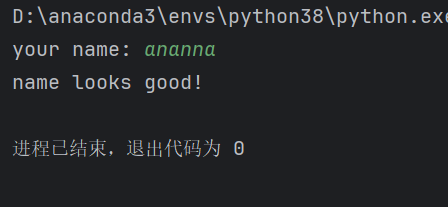
14.重量轉換器
weight = input("Weight: ")#也可以直接float(input("……"))
danwei = input("(L)bs or (K)g: ")
if danwei == "L" or danwei == "l":#磅轉KG,也可以 if danwei.upper()=="L"
ans = float(weight)*0.45
print(f"You are {ans} KG")
elif danwei == "K" or danwei == "k":#KG轉磅
ans = float(weight)*2.20#也可以ans=float(weight)/0.45
print(f"You are {ans} pounds")
輸出結果:
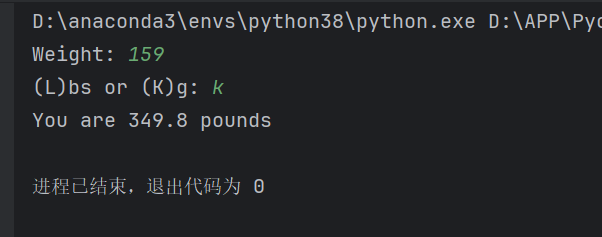
15.while迴圈
i = 1
while i<=5:
print('*'* i)
i = i + 1
print("Done")
輸出結果:
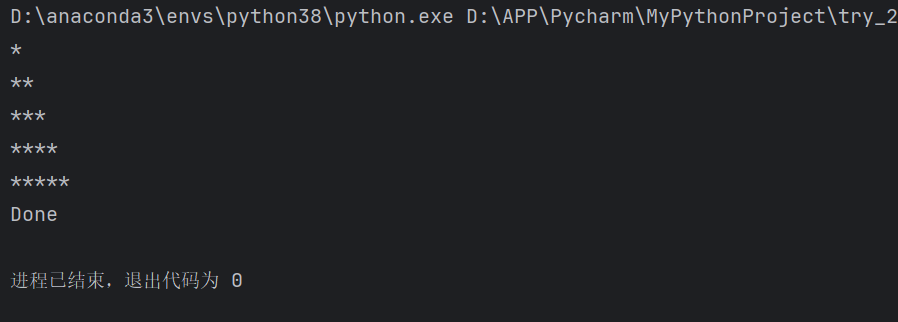
小技巧:變數的重新命名方法
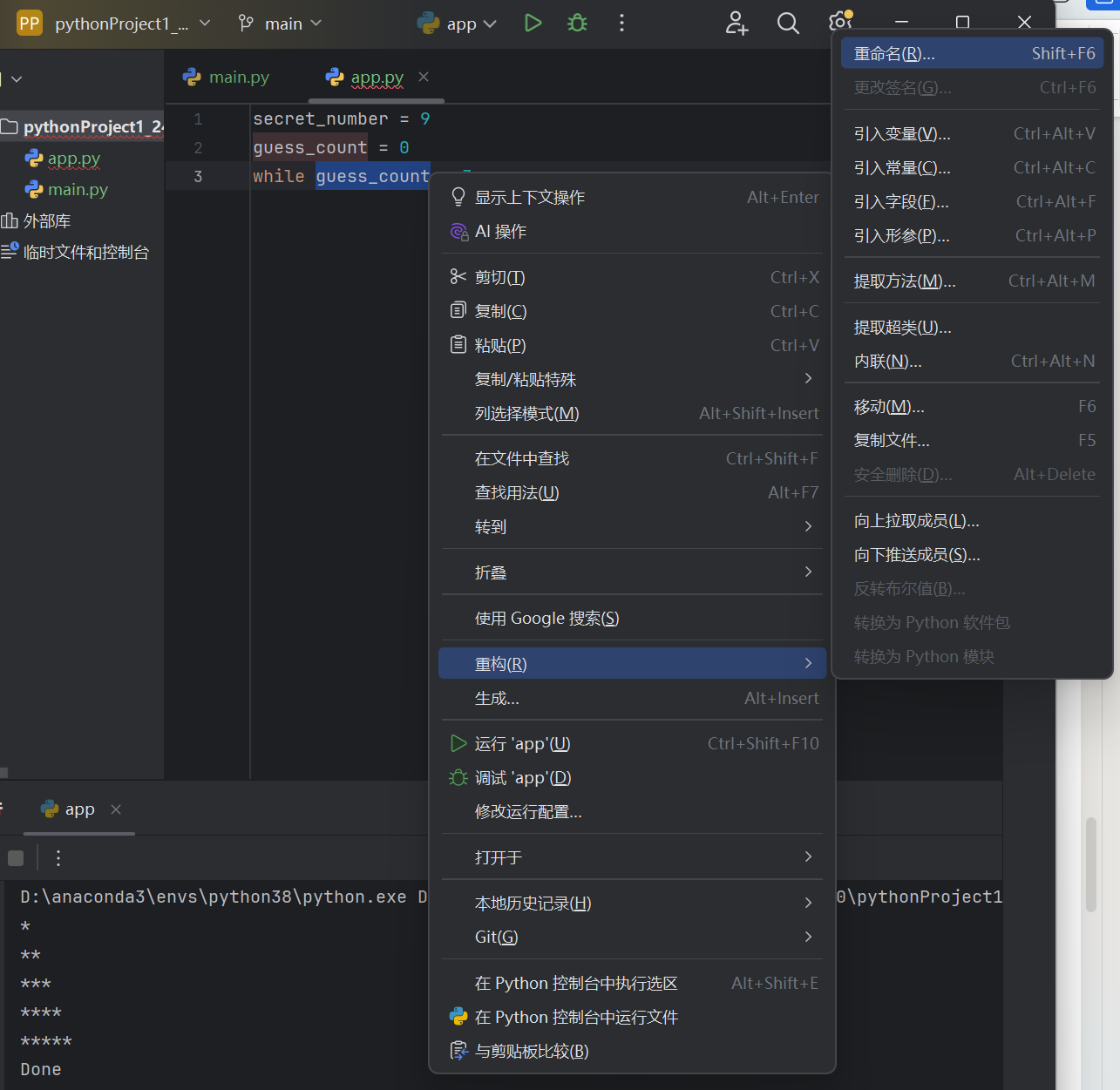
1)猜謎遊戲
secret_number = 9
guess_count = 0
guess_limit = 3
while guess_count < guess_limit:
guess = int(input('Guess: '))
guess_count += 1
if guess == secret_number:
print('You won!')
break
else:#在while迴圈中,可以選擇性含有else部分,當while迴圈中的條件不被滿足時會執行它
print('Sorry! You failed!')
輸出結果:
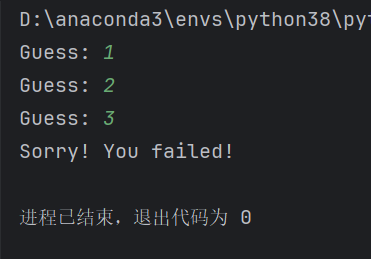
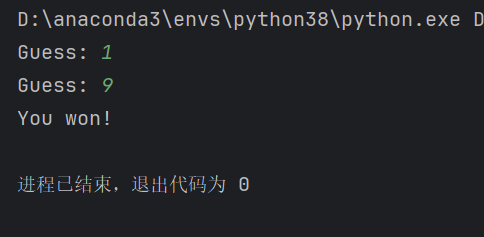
2)汽車遊戲
promot = input(">")
while promot.lower() != "quit":
if promot.lower() == "help":
print('''
start - to start the car
stop - to stop the car
quit - to exit
''')
elif promot.lower() == "start":
print("Car started...Ready to go!")
elif promot.lower() == "stop":
print("Car stopped.")
else:
print("I don't understand that...")
promot = input(">")
輸出結果:
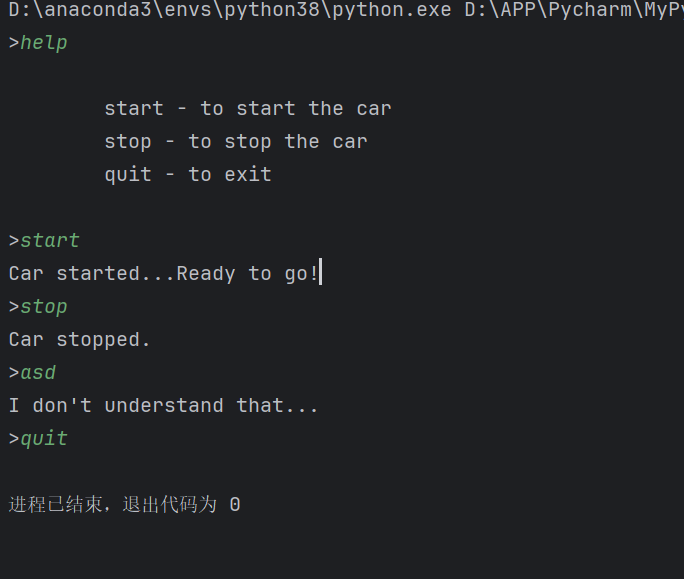
改進一:簡化指令版
promot = input(">").lower()
while promot!= "quit":
if promot == "help":
print('''
start - to start the car
stop - to stop the car
quit - to exit
''')
elif promot == "start":
print("Car started...Ready to go!")
elif promot == "stop":
print("Car stopped.")
else:
print("I don't understand that...")
promot = input(">").lower()#也可以提前把promot定義為空字串"",然後把這個判斷條件放在迴圈最前面
改進二:縮排調整版
command = ""#定義為空字串
started = False
while True:#這表示如果我們不主動break,迴圈會一直執行
command = input("> ").lower()
if command == "start":
if started:
print("Car is already started!")
else:
started = True
print("Car started...")
elif command == "stop":
if not started:
print("Car is already stopped!")
else:
started = False
print("Car stopped.")
elif command == "help":
print('''
start - to start the car
stop - to stop the car
quit - to exit
''')#當我們輸入三引號時,輸出會完全按照原樣列印,所以會多出縮排
elif command == "quit":
break
else:
print("Sorry,I don't understand that...")
16.for迴圈
for 用於迭代集合中的專案
1)迭代字串
for item in 'Python':
print(item)
輸出
P
y
t
h
o
n
2)迭代列表元素
列表中元素為字串:
for item in ['Mosh','John','Sarah']:
print(item)
輸出
Mosh
John
Sarah
列表中元素為數字:
for item in [1,2,3,4]:
print(item)
輸出
1
2
3
4
3)迭代range
for item in range(10):#從0開始,到10(但是不含10)
print(item)
#0
#1
#...
#8
#9
for item in range(5,10):#從5開始,到10(但是不含10)
print(item)
#5
#6
#7
#8
#9
for item in range(5,10,2):#這裡還設定了step
print(item)
#5
#7
#9
練習:
prices = [10,20,30]
sum = 0
for price in prices:
sum += price
print(f"sum:{sum}")
輸出:
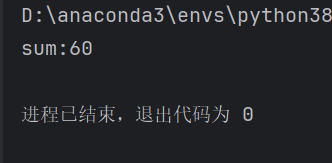
17.巢狀迴圈
#巢狀迴圈
for x in range(4):
for y in range(3):
print(f"({x},{y})")
輸出:
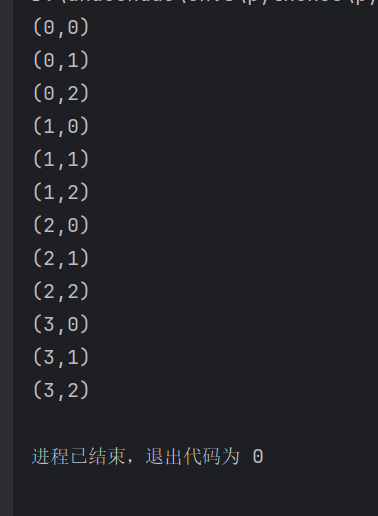
練習(輸出F):
#在python中,可以拿數字×一個字串
numbers = [5,2,5,2,2]
for number in numbers:
print('x'* number )
print("Done")
#方法二:(需要定義一個空字串)
for number in numbers:
output = ''
for item in range(number):
output += 'x'#這個補充字串的方法好奇妙!
print(output)
print("Done")
在python中,可以拿數字×一個字串,字串=字串+字串
輸出:
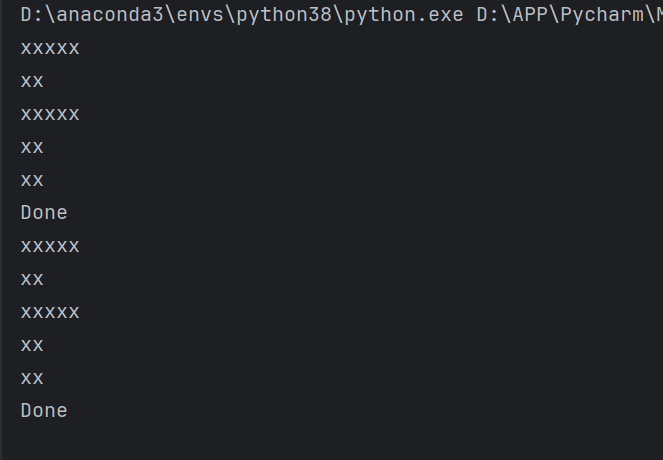
18.列表方法
names = ['John','Bob','Mosh','Sarah','Mary']
print(names[0])
print(names[2])
print(names[-1])
print(names[-2])
print("Done")
print(names[2:])
print(names[2:4])#含前不含後
print(names[0:])#等價於names[:]
#另外,這個就像字串一樣,這個的方括號不會修改最初的列表,他們只是返回一個新的列表
print(names)
print("lalala")
#當然,我們也能輕而易舉修改列表中的內容
names[0] = 'Jon'
print(names)
輸出:
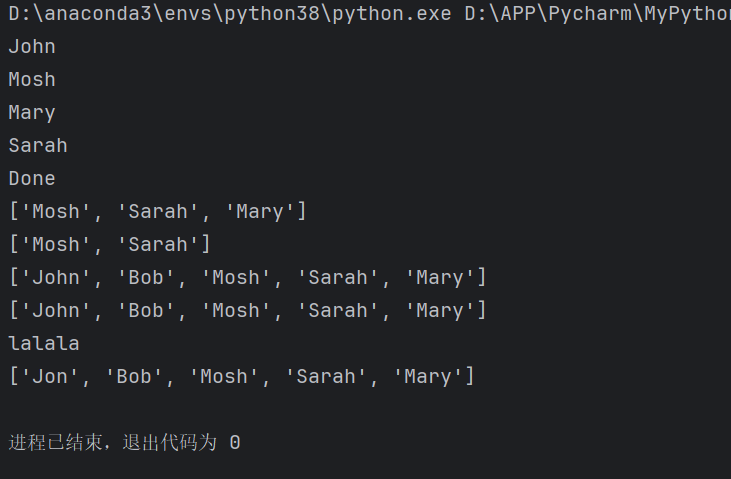
練習(找到列表中最大的元素):
numbers = [3,6,2,8,4,10]
max = numbers[0]
for number in numbers:
if number > max :
max = number
print(f"max is {max}")
輸出:
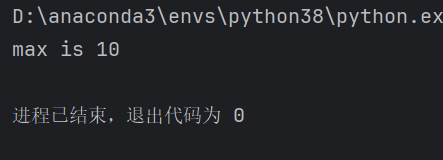
19.二維列表
1)訪問
matrix = [
[1,2,3],
[4,5,6],
[7,8,9]
]
#訪問
print(matrix[0][1])
#2
2)修改
matrix = [
[1,2,3],
[4,5,6],
[7,8,9]
]
matrix[0][1]=20
print(matrix[0][1])
#20
3)遍歷
for row in matrix:
for item in row:
print(item)
輸出:
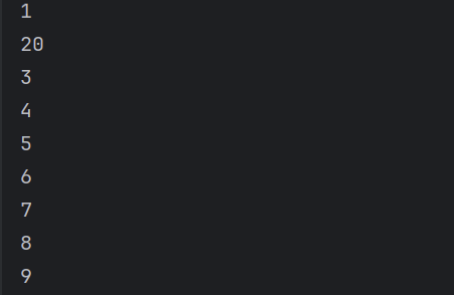
網址:Code with Mosh🃏講真好!(❤ ω ❤)
20.列表方法
1)尾部加數
numbers = [5,2,1,7,4]
numbers.append(20)
print(numbers)#[5, 2, 1, 7, 4, 20]
2)中間加數
numbers = [5,2,1,7,4]
numbers.insert(0,10)
print(numbers)#[10, 5, 2, 1, 7, 4]
3)列表刪數
numbers = [5,2,1,7,4]
numbers.remove(5)#這個remove每次只能刪一個
print(numbers)#[2, 1, 7, 4]
4)清空列表
numbers = [5,2,1,7,4]
numbers.clear()
print(numbers)#[]
5)彈出最後一個元素
numbers = [5,2,1,7,4]
numbers.pop()
print(numbers)#[5, 2, 1, 7]
6)查詢元素是否在某個列表中,在則返回索引
numbers = [5,2,1,7,4]
print(numbers.index(5))#0
#print(numbers.index(50))#ValueError: 50 is not in list
#返回bool值的方法,in,和字串中的用法差不多
print(50 in numbers)#False
7)你想知道一個元素在裡面的個數
numbers = [5,2,1,5,7,4]
print(numbers.count(5))#2
print(numbers.count(0))#0
8)列表排序
numbers = [5,2,1,5,7,4]
#print(numbers.sort())#None
#升序
numbers.sort()
print(numbers)#[1, 2, 4, 5, 5, 7]
#降序
numbers.reverse()
print(numbers)#[7, 5, 5, 4, 2, 1]
9)獨立複製列表
numbers = [5,2,1,5,7,4]
numbers2 = numbers.copy()
numbers.append(10)
print(numbers)#[5, 2, 1, 5, 7, 4, 10]
print(numbers2)#[5, 2, 1, 5, 7, 4]
練習(移除列表中的重複項)
我的方法:
numbers = [2,2,4,6,3,4,1,7]
for number in numbers:
if numbers.count(number)>1:
numbers.remove(number)
print(numbers)
輸出:

老師的方法:
numbers = [2,2,4,6,3,4,1,7]
uniques = []
for number in numbers:
if number not in uniques:
uniques.append(number)
print(uniques)
輸出:

21.元組
元組與列表不同的點在於,我們不能修改它們,這意味著我們不能新增新專案/刪除現有專案,或者彈出。
numbers = (1,2,3,1)
print(numbers.count(1))#返回此元素的個數#2
print(numbers.index(1))#返回此元素第一次出現時的索引#0
#其他有兩個下劃線的方法叫做魔術方法
#另外,元組不支援元素修改
#numbers[0]=10#'tuple' object does not support item assignment
#僅支援訪問
print(numbers[0])#1
22.拆包
不僅適用於元組,還適用於列表。
1)元組
coordinates = (1,2,3)
x = coordinates[0]
y = coordinates[1]
z = coordinates[2]
#上面三行等價於
x, y, z = coordinates
print(x)#1
print(y)#2
2)列表
coordinates = [1,2,3]
x, y, z = coordinates
print(y)#2
23.字典
注意字典中的鍵都應該是唯一的。
1)元素訪問
customer = {
"name": "John Smith",
"age":30,
"is_verified":True
}
#鍵除了字串還可以是數字
#但是值可以是任何東西,比如字串、數字、布林值、列表...
print(customer["name"])#John Smith
#如果使用不存在的鍵
#print(customer["birthdate"]) #KeyError: 'birthdate'
#同樣,字典也是大小寫敏感的
#print(customer["Name"])#KeyError: 'Name'
#get方法:不會在一個鍵不存在時向我們警告
print(customer.get("name"))#John Smith
print(customer.get("birthdate"))#None
#也可若未查到則返回預設值
print(customer.get("birthdate","Jan 1 1980"))#Jan 1 1980
2)元素更新
#另外,也可以更新字典中鍵對應的值
customer = {
"name": "John Smith",
"age":30,
"is_verified":True
}
customer["name"] = "Jack Smith"
print(customer["name"])#Jack Smith
#當然,我們還能新增新的鍵值對
customer["birthdate"] = "Jan 1 1980"
print(customer["birthdate"])#Jan 1 1980
練習(電話號碼翻譯):
phone_number = {
'0':"Zero",
'1':"One",
'2':"Two",
'3':"Three",
'4':"Four",
'5':"Five",
'6':"Six",
'7':"Seven",
'8':"Eight",
'9':"Nine"
}
output = ''
phone = input("Phone: ")
for item in phone:
#output += phone_number[item] + " "
#為了避免使用者輸入字典中沒有的鍵程式警告,我們使用get方法會更好
output +=phone_number.get(item,"!") + " "
print(output)
輸出1:
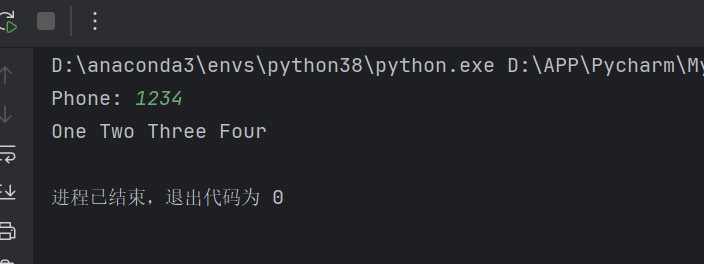
輸出2:
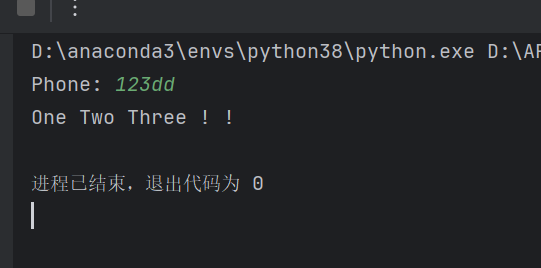
!正在更新中...