Mybatis介紹與入門
1.官方文件
Mybatis中文手冊:mybatis – MyBatis 3 或者 MyBatis中文網
Maven倉庫:Maven Repository: org.mybatis » mybatis » 3.5.7 (mvnrepository.com)
2.概述
2.1傳統Java程式操作DB
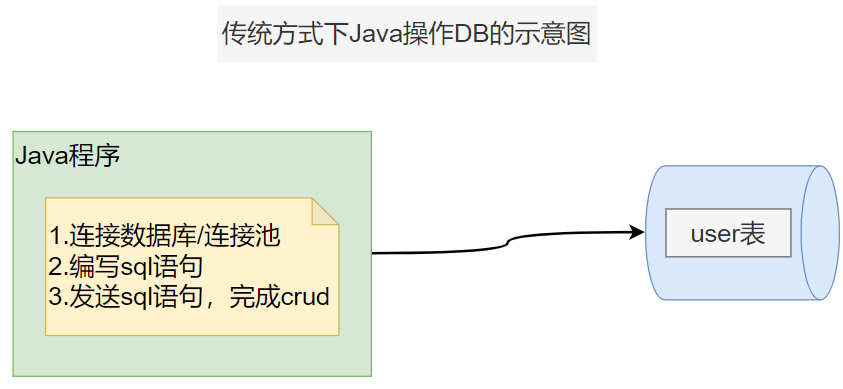
傳統方式運算元據庫的問題:
-
需要自己去連線資料庫,這段程式碼由程式設計師編寫(不一定標準)
-
程式不是OOP的方式來操作DB(我們希望在程式碼層面上是以物件的形式來操作,如insert(Object))
-
SQL語句寫在程式中,屬於硬編碼,沒有解耦
2.2Mybatis基本介紹
- Mybatis 是一個持久層框架
- 前身是 ibatis,在ibatis3.x 時,更名為Mybatis
- Mybatis 在 Java 和 sql 之間提供更加靈活的對映方案
- mybatis 可以對資料庫表的操作(sql,方法)等等直接剝離,寫到 xml 配置檔案,實現和 java 程式碼的解耦
- mybatis 透過 SQL 操作 DB,建庫建表的操作仍需要程式設計師完成
2.3Mybatis工作原理
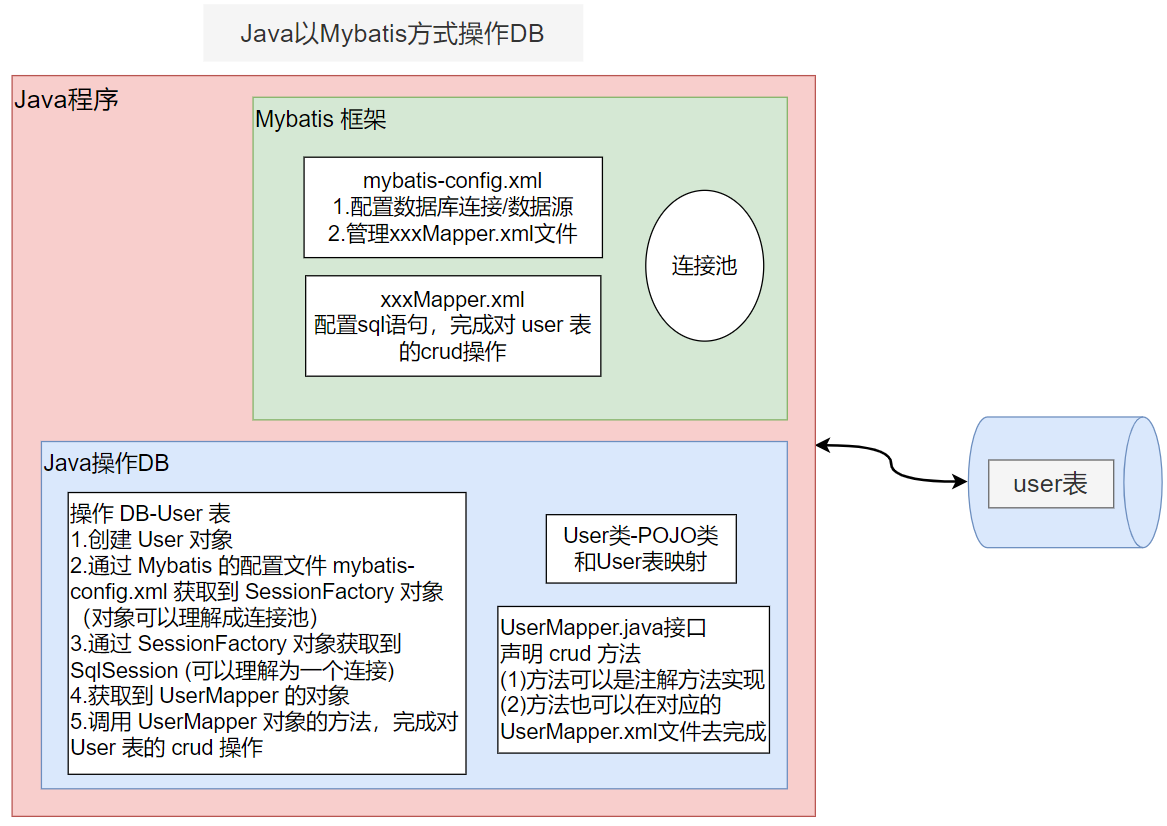
相對於傳統方式,Mybatis的優勢:
- 資料庫的連線/連線池,只需要配置即可,標準可以統一
- 程式以OOP的方式來運算元據庫
- sql語句可以寫在xml檔案中,實現瞭解耦
- Mybatis可以對DB操作進行最佳化,提高效率,比如配置快取
3.Mybatis快速入門
3.1需求說明
要求:開發一個Mybatis專案,透過Mybatis的方式可以對monster表進行crud操作。
3.2環境搭建
(1)建立maven父專案,方便專案的jar包管理
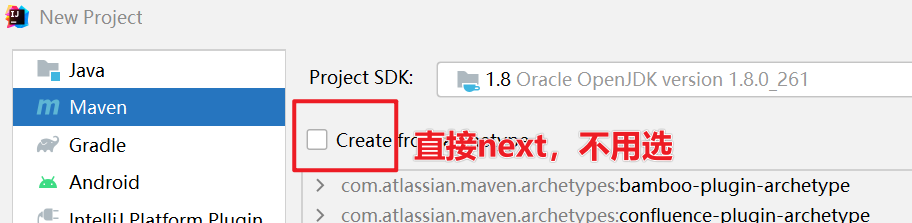
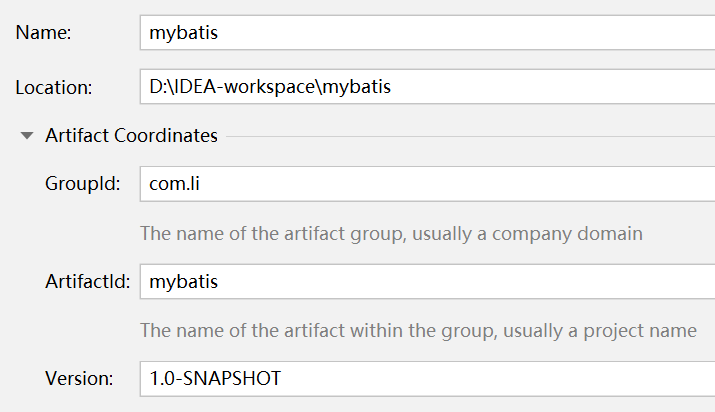
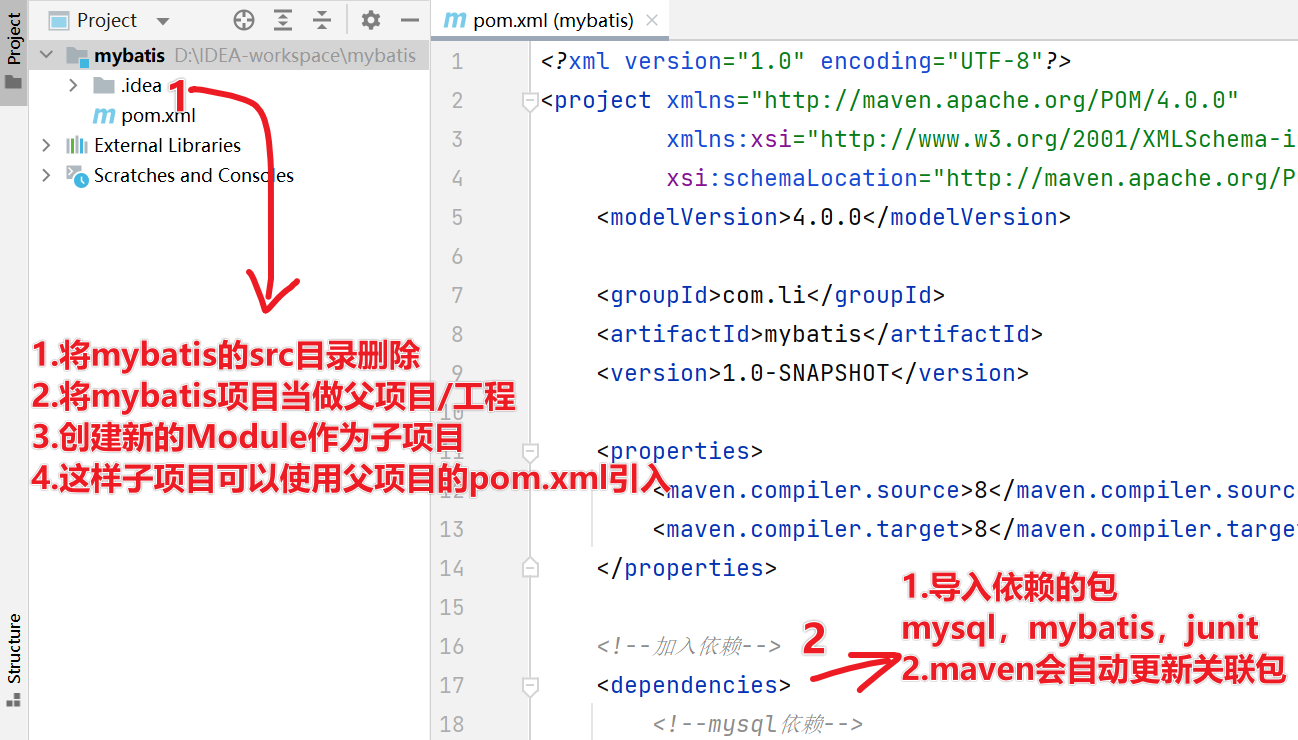
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.li</groupId>
<artifactId>mybatis</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
<!--加入依賴-->
<dependencies>
<!--mysql依賴-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.49</version>
</dependency>
<!--mybatis依賴-->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.7</version>
</dependency>
<!--junit依賴-->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<!--指定該jar的作用範圍在test目錄下-->
<scope>test</scope>
</dependency>
</dependencies>
</project>
(2)建立maven父專案的Module,視為子專案
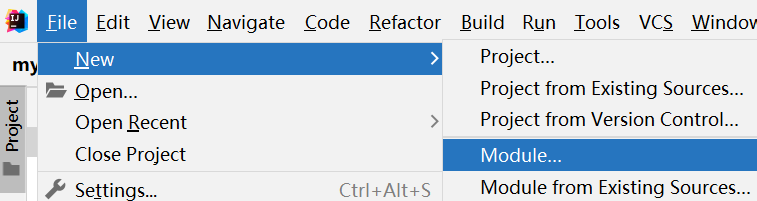
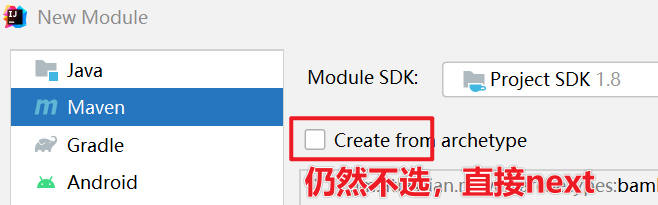
此時父專案的pom.xml檔案如下:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<!--說明:
1.將 mybatis作為父專案管理多個子模組/子專案
2.父專案的完整的座標 groupId[組織名]+artifactId[專案名]
3.後面該父專案會管理多個子模組/子專案,將來父專案中的引入的依賴可以直接給子專案用,
這樣開發簡單,提高複用性,也便於管理
4.<packaging>pom</packaging> 表示父專案以多個子模組/子專案管理工程
5. 在 modules指定管理哪些子模組
-->
<groupId>com.li</groupId>
<artifactId>mybatis</artifactId>
<packaging>pom</packaging>
<version>1.0-SNAPSHOT</version>
<modules>
<module>mybatis_quickstart</module>
</modules>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
<!--加入依賴,略,詳見上面-->
<dependencies>
<!--mysql依賴-->
<!--mybatis依賴-->
<!--junit依賴-->
</dependencies>
</project>
子模組的pom.xml檔案:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<!--說明
1.parent指定了該模組的父專案的座標 artifactId+groupId
2.這樣配置後,子模組就可以使用到父專案的依賴
-->
<parent>
<artifactId>mybatis</artifactId>
<groupId>com.li</groupId>
<version>1.0-SNAPSHOT</version>
</parent>
<modelVersion>4.0.0</modelVersion>
<!--子模組的名稱,當前子模組的groupId預設和父專案的groupId相同,即com.li-->
<artifactId>mybatis_quickstart</artifactId>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
</project>
3.2程式碼實現
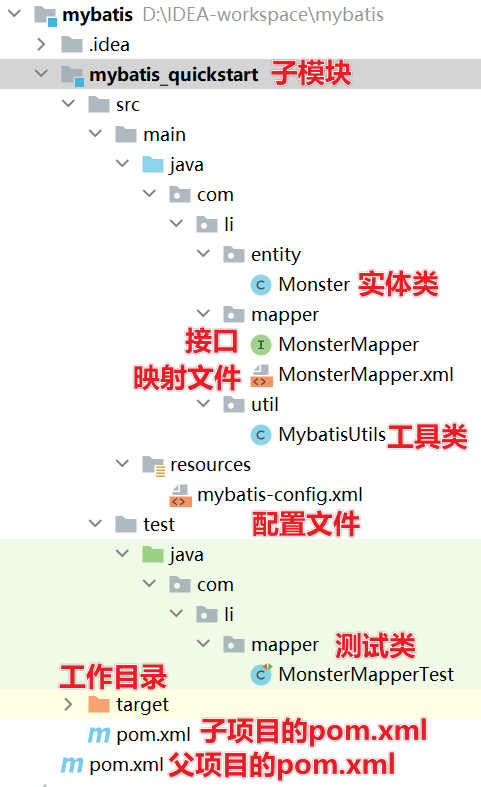
(1)建立資料庫和表
-- 建立資料庫
CREATE DATABASE `mybatis`;
USE `mybatis`;
-- 建立monster表
CREATE TABLE `monster`(
`id` INT NOT NULL AUTO_INCREMENT,
`age` INT NOT NULL,
`birthday` DATE DEFAULT NULL,
`email` VARCHAR(255) NOT NULL,
`gender` TINYINT NOT NULL,-- 1 male, 0 female
`name` VARCHAR(255) NOT NULL,
`salary` DOUBLE NOT NULL,
PRIMARY KEY(`id`)
)CHARSET=utf8
(2)在子模組 mybatis_quickstart 的 resources 目錄建立配置檔案 mybatis-config.xml(名稱隨意)
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"https://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<environments default="development">
<environment id="development">
<!--配置事務管理器-->
<transactionManager type="JDBC"/>
<!--配置資料來源-->
<dataSource type="POOLED">
<!--配置驅動-->
<property name="driver" value="com.mysql.jdbc.Driver"/>
<!--配置連線mysql的url
1.jdbc:mysql 協議
2.127.0.0.1:3306 連線資料庫的 ip+port
3.mybatis 連線的資料庫名稱
4.useSSL=true 表示使用安全連線
5.& 表示 & 符號,這裡這樣寫是為了轉義,防止解析錯誤
6.useUnicode=true 使用Unicode,防止編碼錯誤
7.characterEncoding=UTF-8 使用utf-8,防止中文亂碼
-->
<property name="url" value="jdbc:mysql://127.0.0.1:3306/mybatis?
useSSL=true&useUnicode=true&characterEncoding=UTF-8"/>
<!--使用者名稱-->
<property name="username" value="root"/>
<!--密碼-->
<property name="password" value="123456"/>
</dataSource>
</environment>
</environments>
<!--這裡配置需要管理的mapper.xml檔案-->
<mappers>
<mapper resource="com/li/mapper/MonsterMapper.xml"/>
</mappers>
</configuration>
(3)建立實體類 Monster.java
package com.li.entity;
import java.util.Date;
/**
* @author 李
* @version 1.0
* 1.Monster和 monster表有對應關係
* 2.原生的sql語句查詢結果還是要封裝成物件
* 3.因此要求這裡的實體類屬性名和表的欄位名保持相同
*/
public class Monster {
//屬性和表的欄位對應
private Integer id;
private Integer age;
private String name;
private String email;
private Date birthday;
private double salary;
private Integer gender;
public Monster() {
}
public Monster(Integer id, Integer age, String name, String email,
Date birthday, double salary, Integer gender) {
this.id = id;
this.age = age;
this.name = name;
this.email = email;
this.birthday = birthday;
this.salary = salary;
this.gender = gender;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Date getBirthday() {
return birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
public Integer getGender() {
return gender;
}
public void setGender(Integer gender) {
this.gender = gender;
}
@Override
public String toString() {
return "Monster{" +
"id=" + id +
", age=" + age +
", name='" + name + '\'' +
", email='" + email + '\'' +
", birthday=" + birthday +
", salary=" + salary +
", gender=" + gender +
'}';
}
}
(4)建立MonsterMapper介面
package com.li.mapper;
import com.li.entity.Monster;
/**
* @author 李
* @version 1.0
* 1.MonsterMapper介面用於宣告操作monster表的方法
* 2.這些方法可以透過註解或者xml檔案來實現
*/
public interface MonsterMapper {
//新增monster
public void addMonster(Monster monster);
}
(5)建立介面對應的對映檔案,實現方法
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"https://mybatis.org/dtd/mybatis-3-mapper.dtd">
<!--說明
1.這是一個mapper.xml檔案
2.該檔案可以去實現對應的介面的方法
3.namespace 指定當前xml檔案和哪個介面對應!
-->
<mapper namespace="com.li.mapper.MonsterMapper">
<!--配置addMonster方法
1.id 就是對應介面的方法名
2.parameterType 方法的入參
3.寫入sql語句
4.(`age`,`birthday`,`email`,`gender`,`name`,`salary`) 表的欄位
5.#{age},#{birthday},#{email},#{gender},#{name},#{salary} 從傳入的Monster物件屬性中獲取
6.注意這裡的 #{age}對應Monster物件的屬性名,其他類推
-->
<insert id="addMonster" parameterType="com.li.entity.Monster">
INSERT INTO `monster`
(`age`,`birthday`,`email`,`gender`,`name`,`salary`)
VALUES (#{age},#{birthday},#{email},#{gender},#{name},#{salary})
</insert>
</mapper>
(6)工具類MybatisUtils,用於獲取連線
package com.li.util;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import java.io.IOException;
import java.io.InputStream;
/**
* @author 李
* @version 1.0
* MybatisUtils工具類可以得到 SqlSession
*/
public class MybatisUtils {
private static SqlSessionFactory sqlSessionFactory;
//靜態程式碼塊中初始化 SqlSessionFactory-會話工廠
static {
try {
//指定資原始檔(配置檔案)
String resource = "mybatis-config.xml";
//獲取配置檔案對應的inputStream
//說明:載入檔案時,預設到resource目錄對應的==>執行後的工作目錄target/classes找
InputStream resourceAsStream = Resources.getResourceAsStream(resource);
sqlSessionFactory = new SqlSessionFactoryBuilder().build(resourceAsStream);
System.out.println("sqlSessionFactory=" + sqlSessionFactory.getClass());
} catch (IOException e) {
e.printStackTrace();
}
}
//編寫方法,返回一個SqlSession物件-會話
public static SqlSession getSqlSession() {
return sqlSessionFactory.openSession();
}
}
3.3測試
3.3.1新增
test 目錄中建立測試類 MonsterMapperTest.java
package com.li.mapper;
import com.li.entity.Monster;
import com.li.util.MybatisUtils;
import org.apache.ibatis.session.SqlSession;
import org.junit.Before;
import org.junit.Test;
import java.util.Date;
/**
* @author 李
* @version 1.0
*/
public class MonsterMapperTest {
//屬性
private SqlSession sqlSession;
private MonsterMapper monsterMapper;
/**
* 編寫方法,完成初始化
* 當一個A方法標註了註解 @Before時,表示在執行你的目標測試方法前會先執行A方法
*/
@Before
public void init() {
sqlSession = MybatisUtils.getSqlSession();
//獲取到 monsterMapper物件 =class com.sun.proxy.$Proxy7代理物件)
//底層使用了動態代理機制,返回一個代理物件,透過這個物件去執行你的方法
monsterMapper = sqlSession.getMapper(MonsterMapper.class);
System.out.println("monsterMapper的型別=" + monsterMapper.getClass());
}
@Test
public void addMonster() {
for (int i = 0; i < 2; i++) {
Monster monster = new Monster();
monster.setAge(10 + i);
monster.setBirthday(new Date());
monster.setEmail("kate@qq.com");
monster.setGender(1);
monster.setName("松鼠精" + i + "號");
monster.setSalary(1000 + i * 10);
monsterMapper.addMonster(monster);
System.out.println("新增物件--" + monster);
}
//如果是增刪改,需要提交事務,因為它預設不提交
if (sqlSession != null) {
sqlSession.commit();
//將連線釋放回連線池
sqlSession.close();
}
System.out.println("儲存成功....");
}
}
上面的程式碼一般會出現找不到xxxmapper.xml錯誤,原因是載入的資原始檔時是到target目錄下載入的,當maven專案執行時,源工程的MonsterMapper.xml並沒有被複製到target目錄下。
解決方法:在父工程的pom.xml加入build配置,作用是配置resource,防止資源匯出失敗
<!--將指定的目錄以及它們的子目錄下的xml、properties檔案在build專案時
匯出到對應的target目錄下-->
<build>
<resources>
<resource>
<directory>src/main/java</directory>
<includes>
<include>**/*.xml</include>
</includes>
</resource>
<resource>
<directory>src/main/resources</directory>
<includes>
<include>**/*.xml</include>
<include>**/*.properties</include>
</includes>
</resource>
</resources>
</build>
然後選擇build project

如果不行,可能是maven版本衝突,在setting中切換maven版本,然後點選父工程的maven的clean重新構建。
測試結果:

資料庫monster表增加了兩條記錄:

3.3.2返回自增長
有時候我們需要獲取資料庫中自動生成(AUTO_INCREMENT)的主鍵,這時候可以使用Mybatis的useGeneratedKeys
(開啟新增主鍵返回功能)和 keyProperty
(實體的主鍵屬性)來配合獲取。
(1)修改對映檔案MonsterMapper.xml,在該檔案中的方法對映中新增 useGenerateKeys 和 keyProperty
<mapper namespace="com.li.mapper.MonsterMapper">
<insert id="addMonster" parameterType="com.li.entity.Monster" useGeneratedKeys="true" keyProperty="id">
INSERT INTO `monster`
(`age`,`birthday`,`email`,`gender`,`name`,`salary`)
VALUES (#{age},#{birthday},#{email},#{gender},#{name},#{salary})
</insert>
</mapper>
(2)修改測試類,輸出主鍵

(3)測試結果:

資料庫表格:

3.3.3刪除
(1)修改 MonsterMapper.java 介面,宣告 delMonster() 方法
//根據id刪除一個Monster
public void delMonster(Integer id);
(2)在對映檔案 MonsterMapper.xml 中實現 delMonster() 方法
<!--配置delMonster方法
1.java.lang.Integer是Java型別,可以簡寫為Integer
2.寫入delete語句
-->
<delete id="delMonster" parameterType="Integer">
DELETE FROM `monster` WHERE `id`=#{id};
</delete>
(3)修改測試類,新增測試方法
package com.li.mapper;
import com.li.entity.Monster;
import com.li.util.MybatisUtils;
import org.apache.ibatis.session.SqlSession;
import org.junit.Before;
import org.junit.Test;
import java.util.Date;
/**
* @author 李
* @version 1.0
*/
public class MonsterMapperTest {
//屬性
private SqlSession sqlSession;
private MonsterMapper monsterMapper;
@Before
public void init() {
sqlSession = MybatisUtils.getSqlSession();
monsterMapper = sqlSession.getMapper(MonsterMapper.class);
System.out.println("monsterMapper的型別=" + monsterMapper.getClass());
}
@Test
public void delMonster() {
//刪除id=2的記錄
monsterMapper.delMonster(2);
//如果是增刪改操作,需要提交事務,因為它預設不提交
if (sqlSession != null) {
sqlSession.commit();
//將連線釋放回連線池
sqlSession.close();
}
System.out.println("刪除成功....");
}
}
測試結果:

資料庫id=2的記錄成功被刪除:

3.3.4修改&查詢
(1)修改 MonsterMapper.java 介面,宣告修改和查詢的方法
//修改Monster
public void updateMonster(Monster monster);
//查詢-根據id
public Monster getMonsterById(Integer id);
//查詢所有的Monster
public List<Monster> findAllMonster();
(2) 對映檔案 MonsterMapper.xml 中實現方法
<!--配置/實現updateMonster方法
這裡的入參可以使用型別別名,為Java型別設定一個縮寫名字,可以降低冗餘的全限定類名的書寫
型別別名在mybatis的配置檔案中配置,而不是當前的檔案-->
<update id="updateMonster" parameterType="Monster">
UPDATE `monster`
SET `age`=#{age},`birthday`=#{birthday},`email`=#{email},
`gender`=#{gender},`name`=#{name},`salary`=#{salary}
WHERE `id`=#{id};
</update>
<!--配置/實現getMonsterById方法,
resultType 期望從這條語句中返回結果的類全限定名或別名。
注意,如果返回的是集合,那應該設定為集合包含的型別,而不是集合本身的型別。-->
<select id="getMonsterById" resultType="Monster">
SELECT * FROM `monster` WHERE id=#{id};
</select>
<!--實現findAllMonster方法
如果返回的是集合,那應該設定為集合包含的型別,而不是集合本身的型別-->
<select id="findAllMonster" resultType="Monster">
SELECT * FROM `monster`;
</select>
(3)配置別名

(4)修改測試類,新增測試方法
package com.li.mapper;
import com.li.entity.Monster;
import com.li.util.MybatisUtils;
import org.apache.ibatis.session.SqlSession;
import org.junit.Before;
import org.junit.Test;
import java.util.Date;
import java.util.List;
/**
* @author 李
* @version 1.0
*/
public class MonsterMapperTest {
//屬性
private SqlSession sqlSession;
private MonsterMapper monsterMapper;
@Before
public void init() {
sqlSession = MybatisUtils.getSqlSession();
monsterMapper = sqlSession.getMapper(MonsterMapper.class);
System.out.println("monsterMapper的型別=" + monsterMapper.getClass());
}
@Test
public void updateMonster() {
Monster monster = new Monster();
monster.setAge(50);
monster.setBirthday(new Date());
monster.setEmail("king@qq.com");
monster.setGender(0);
monster.setName("白骨精");
monster.setSalary(20000);
monster.setId(3);
monsterMapper.updateMonster(monster);
if (sqlSession != null) {
sqlSession.commit();
sqlSession.close();
}
System.out.println("修改成功..");
}
@Test
public void getMonsterById() {
Monster monster = monsterMapper.getMonsterById(3);
System.out.println("monster=" + monster);
if (sqlSession != null) {
sqlSession.close();
}
}
@Test
public void findAllMonster() {
List<Monster> monsters = monsterMapper.findAllMonster();
for (Monster monster : monsters) {
System.out.println("monster="+monster);
}
if (sqlSession != null) {
sqlSession.close();
}
}
}
測試updateMonster()方法:


測試getMonsterById()方法:

測試findAllMonster()方法:

4.日誌輸出-檢視SQL
在開發Mybatis程式時,比如執行測試方法,程式設計師往往需要檢視底層發給MySql的SQL語句到底長什麼樣,這時應該如何檢視?
解決方案:日誌輸出。

(1)修改Mybatis-config.xml檔案,加入日誌輸出配置,方便分析SQL語句

(2)比如測試updateMonster() 方法時,輸出的結果為:
Logging initialized using 'class org.apache.ibatis.logging.stdout.StdOutImpl' adapter.
PooledDataSource forcefully closed/removed all connections.
PooledDataSource forcefully closed/removed all connections.
PooledDataSource forcefully closed/removed all connections.
PooledDataSource forcefully closed/removed all connections.
sqlSessionFactory=class org.apache.ibatis.session.defaults.DefaultSqlSessionFactory
monsterMapper的型別=class com.sun.proxy.$Proxy7
Opening JDBC Connection
Wed Feb 22 19:09:14 CST 2023 WARN: Establishing SSL connection without server's identity verification is not recommended. According to MySQL 5.5.45+, 5.6.26+ and 5.7.6+ requirements SSL connection must be established by default if explicit option isn't set. For compliance with existing applications not using SSL the verifyServerCertificate property is set to 'false'. You need either to explicitly disable SSL by setting useSSL=false, or set useSSL=true and provide truststore for server certificate verification.
Created connection 1475491159.
Setting autocommit to false on JDBC Connection [com.mysql.jdbc.JDBC4Connection@57f23557]
==> Preparing: UPDATE `monster` SET `age`=?,`birthday`=?,`email`=?, `gender`=?,`name`=?,`salary`=? WHERE `id`=?;
==> Parameters: 50(Integer), 2023-02-22 19:09:14.079(Timestamp), king333@qq.com(String), 0(Integer), 白骨精(String), 20000.0(Double), 3(Integer)
<== Updates: 1
Committing JDBC Connection [com.mysql.jdbc.JDBC4Connection@57f23557]
Resetting autocommit to true on JDBC Connection [com.mysql.jdbc.JDBC4Connection@57f23557]
Closing JDBC Connection [com.mysql.jdbc.JDBC4Connection@57f23557]
Returned connection 1475491159 to pool.
修改成功..
Process finished with exit code 0
當進行多表聯查的時候,日誌輸出對我們的除錯很有幫助。
5.練習
