Django預設是前後端繫結的,提供了Template和Form,現在流行前後端分離專案,Python大佬坐不住了,於是便有了Django REST framework:https://github.com/tomchristie
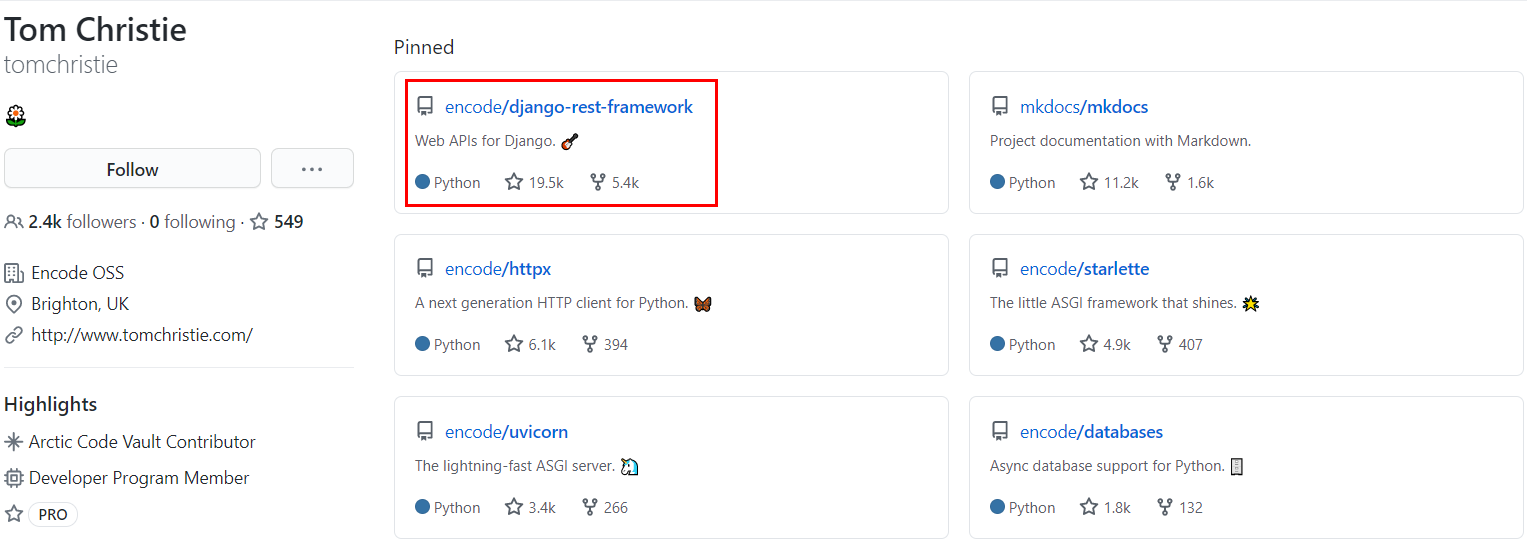
官網:https://www.django-rest-framework.org/
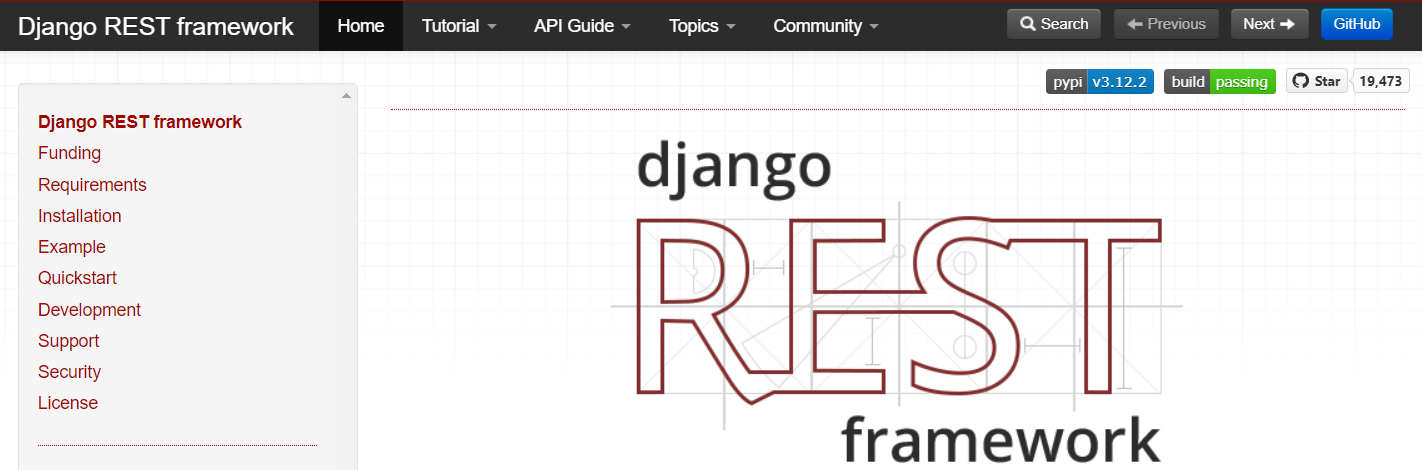
Django REST framework(簡稱DRF)是個Python技術棧的後端框架,用來構建RESTful API。
RESTful API
REST,是指REpresentational State Transfer,有個精闢的解釋什麼是RESTful:
- 看URL就知道要什麼
- 看Method就知道幹什麼
- 看Status Code就知道結果如何
良好的RESTful API設計的基本原則是:
- 返回JSON
- 嚴禁亂用狀態碼
- 處理好分頁
- 返回具體的實體資料而不是返回通用的JSON資料
- 請求物件有預設值
建立專案
接下來我們使用DRF建立一個簡單的API,允許管理員檢視和編輯使用者和組。
先建立名為tutorial
的project和名為quickstart
的app:
# 建立專案目錄
mkdir tutorial
cd tutorial
# 建立Python虛擬環境
python -m venv env
# 啟用虛擬環境
env\Scripts\activate.bat
# Mac中使用`source env/bin/activate`
# 在虛擬環境中安裝Django和Django REST framework
pip install django
pip install djangorestframework
# 建立project,注意最後有個“.”,表示在當前目錄建立
django-admin startproject tutorial .
cd tutorial
# 建立app
django-admin startapp quickstart
cd ..
建立好的目錄結構如下:
$ pwd
<some path>/tutorial
$ find .
.
./manage.py
./tutorial
./tutorial/__init__.py
./tutorial/quickstart
./tutorial/quickstart/__init__.py
./tutorial/quickstart/admin.py
./tutorial/quickstart/apps.py
./tutorial/quickstart/migrations
./tutorial/quickstart/migrations/__init__.py
./tutorial/quickstart/models.py
./tutorial/quickstart/tests.py
./tutorial/quickstart/views.py
./tutorial/settings.py
./tutorial/urls.py
./tutorial/wsgi.py
一般不會把app放到project裡面,這裡是為了避免命名衝突。
接著同步資料庫:
python manage.py migrate
然後建立一個超級管理員,密碼password123
:
python manage.py createsuperuser --email admin@example.com --username admin
Serializers
序列化是指把資料庫模型轉換為JSON。新建模組tutorial/quickstart/serializers.py
:
from django.contrib.auth.models import User, Group
from rest_framework import serializers
class UserSerializer(serializers.HyperlinkedModelSerializer):
class Meta:
model = User
fields = ['url', 'username', 'email', 'groups']
class GroupSerializer(serializers.HyperlinkedModelSerializer):
class Meta:
model = Group
fields = ['url', 'name']
Views
檢視用來接受Web請求並且返回Web響應。開啟tutorial/quickstart/views.py
,新增程式碼:
from django.contrib.auth.models import User, Group
from rest_framework import viewsets
from rest_framework import permissions
from tutorial.quickstart.serializers import UserSerializer, GroupSerializer
class UserViewSet(viewsets.ModelViewSet):
"""
API endpoint that allows users to be viewed or edited.
"""
queryset = User.objects.all().order_by('-date_joined')
serializer_class = UserSerializer
permission_classes = [permissions.IsAuthenticated]
class GroupViewSet(viewsets.ModelViewSet):
"""
API endpoint that allows groups to be viewed or edited.
"""
queryset = Group.objects.all()
serializer_class = GroupSerializer
permission_classes = [permissions.IsAuthenticated]
URLs
配置路由,開啟tutorial/urls.py
,新增程式碼:
from django.urls import include, path
from rest_framework import routers
from tutorial.quickstart import views
router = routers.DefaultRouter()
router.register(r'users', views.UserViewSet)
router.register(r'groups', views.GroupViewSet)
# Wire up our API using automatic URL routing.
# Additionally, we include login URLs for the browsable API.
urlpatterns = [
path('', include(router.urls)),
path('api-auth/', include('rest_framework.urls', namespace='rest_framework'))
]
因為這裡用的不是view而是viewsets,所以可以自動生成API的URLconf,只需要註冊class即可。
也可以不用viewsets,用view,再自定義API URL。
Pagination
分頁用來控制每頁返回多少資料,在tutorial/settings.py
中新增:
REST_FRAMEWORK = {
'DEFAULT_PAGINATION_CLASS': 'rest_framework.pagination.PageNumberPagination',
'PAGE_SIZE': 10
}
Settings
在tutorial/settings.py
中,把'rest_framework'
新增到INSTALLED_APPS
:
INSTALLED_APPS = [
...
'rest_framework',
]
測試API
啟動專案:
python manage.py runserver
訪問http://127.0.0.1:8000/users/
,點選右上角用超管登入,即可看到:
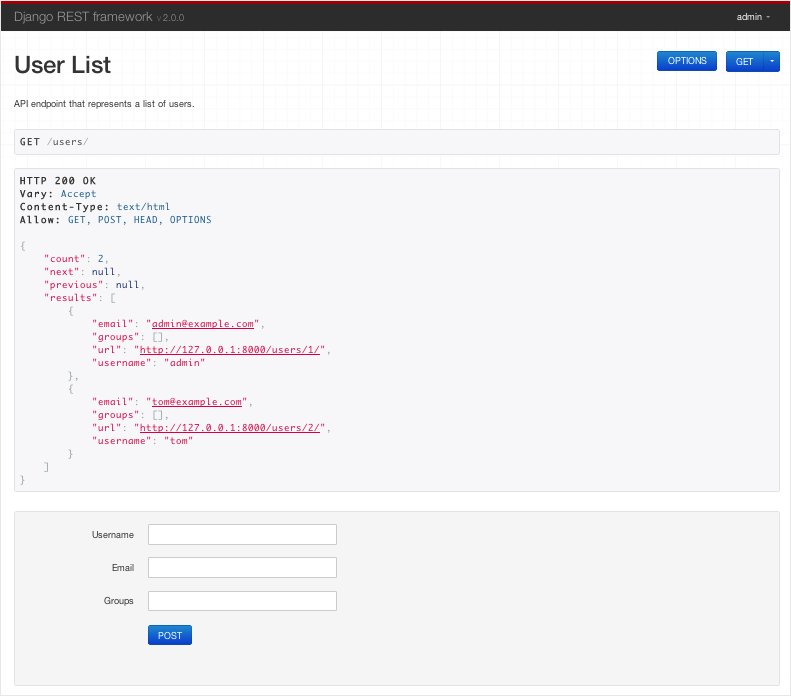
東方說
本文是Django REST framework系列的開篇,內容參考的是官網的Tutorial。學了Django再看DRF,思路清晰多了,雖然我程式碼能力不強,但總是在追求規範和標準,難道是因為做測試的職業病麼?
終於修復了從部落格園複製貼上到公眾號程式碼塊自動換行沒有滾動條的問題,F12看了才知道有個樣式被覆蓋了,加上這句就搞定了:
#topics .postBody pre { white-space: pre !important; }
參考資料: