基於百度飛漿PaddlePaddle的影像分割學習心得
基於百度飛漿PaddlePaddle的影像分割學習心得
學習的步驟:
a.百度AI Studio平臺上notebook的使用規則;
b.PaddlePaddle的建構神經網路模型的基本流程與所需呼叫的基本函式,並進行簡單的模型的構建與訓練;
c.學習相關課程,影像分割。百度高階工程師手敲程式碼,幫助理解。附課程連結:https://aistudio.baidu.com/aistudio/education/group/info/1767
d.實踐調整程式碼,利用API實現自己的完整程式碼。前期基本包括,資料匯入(basic_dataload)、資料預處理(basic_transform)以及模型構建(basic_modle)。最後,進行網路的完整構建,包括有VGG、U-Net、PSPNet以及DeepLab等的構建。
使用notebook的一些體驗:
在使用notebook時,是以cell為基本執行單位,一個cell就是一個執行主體。cell有兩種模式,一種為程式碼執行模式(Code模式)、一種為文字類模式(Markdown模式)。如下圖所示,兩種cell自身直接也可以切換,但注意,執行程式碼時需要採用Code模式。


在使用AI Studio過程中,掌握資料的匯入也是常規操作。在匯入資料夾的時候需要傳入壓縮檔案,如你匯入格式為.zip檔案,則需要在cell上寫!unzip + 'work/dummy_data'(絕對路徑),並執行才成功將其匯入。
在notebook右下方有變數監控、執行歷史、效能監控,可以方便檢視執行過程以及CPU/GPU的使用情況。
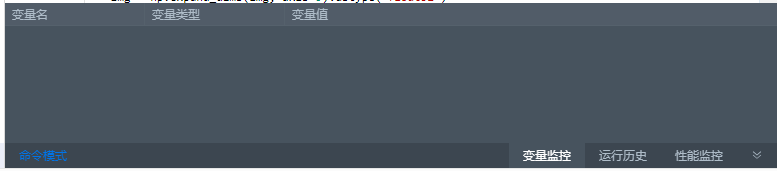
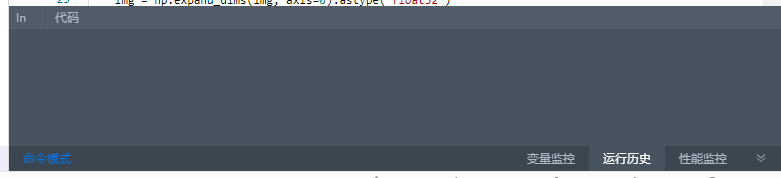
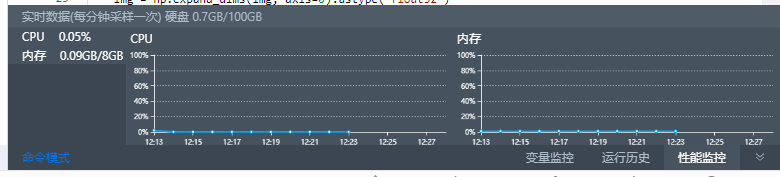
前期基本程式碼的構建(都已經執行檢測,可成功run)
basic_dataload程式碼,可在百度AI Studio上使用並進行基本測試。
import os
import random
import numpy as np
import cv2
import paddle.fluid as fluid
class Transform(object):
def __init__(self, size=256):
self.size = size
def __call__(self, input, label):
input = cv2.resize(input, (self.size, self.size), interpolation=cv2.INTER_LINEAR)
label = cv2.resize(label, (self.size, self.size), interpolation=cv2.INTER_LINEAR)
return input,label
class BasicDataLoader():
def __init__(self,
image_folder,
image_list_file,
transform=None,
shuffle=True):
self.image_folder = image_folder
self.image_list_file = image_list_file
self.transform = transform
self.shuffle = shuffle
self.data_list = self.read_list()
def read_list(self):
data_list = []
with open(self.image_list_file,'r') as infile:
for line in infile.readlines():
data_path = os.path.join(self.image_folder, line.split()[0])
label_path = os.path.join(self.image_folder, line.split()[1])
data_list.append((data_path, label_path))
random.shuffle(data_list)
return data_list
def preprocess(self, data, label):
h, w, c = data.shape
h_gt, w_gt = label.shape
assert h == h_gt, "Error"
assert w == w_gt, 'Error'
if self.transform:
data,label = self.transform(data, label)
label = label[:, :, np.newaxis]
return data, label
def __len__(self):
return len(self.data_list)
def __call__(self):
for data_path, label_path in self.data_list:
data = cv2.imread(data_path, cv2.IMREAD_COLOR)
data = cv2.cvtColor(data, cv2.COLOR_BGR2RGB)
label = cv2.imread(label_path, cv2.IMREAD_GRAYSCALE)
print(data.shape, label.shape)
data, label = self.preprocess(data, label)
yield data, label
def main():
batch_size = 5
place = fluid.CPUPlace()
with fluid.dygraph.guard(place):
# TODO: craete BasicDataloder instance
# image_folder="./dummy_data"
# image_list_file="./dummy_data/list.txt"
# TODO: craete fluid.io.DataLoader instance
# TODO: set sample generator for fluid dataloader
transform = Transform(256)
# create BasicDataloder instance
basic_dataloader = BasicDataLoader(
image_folder=r'work/dummy_data',
image_list_file=r'work/dummy_data/list.txt',
transform=transform,
shuffle=True
)
# create fluid.io.DataLoader instance
dataloader = fluid.io.DataLoader.from_generator(capacity=1, use_multiprocess=False)
# set sample generator for fluid dataloader
dataloader.set_sample_generator(basic_dataloader,
batch_size=batch_size,
places=place)
num_epoch = 2
for epoch in range(1, num_epoch+1):
print(f'Epoch [{epoch}/{num_epoch}]:')
for idx, (data, label) in enumerate(dataloader):
print(f'Iter {idx}, Data shape: {data.shape}, Label shape: {label.shape}')
if __name__ == "__main__":
main()
basic_modle程式碼:
import paddle
import paddle.fluid as fluid
from paddle.fluid.dygraph import Pool2D #TODO
from paddle.fluid.dygraph import Conv2D #TODO
from paddle.fluid.dygraph import to_variable #TODO
import numpy as np
np.set_printoptions(precision=2)
class BasicModel(fluid.dygraph.Layer):
# BasicModel contains:
# 1. pool: 4x4 max pool op, with stride 4
# 2. conv: 3x3 kernel size, takes RGB image as input and output num_classes channels,
# note that the feature map size should be the same
# 3. upsample: upsample to input size
#
# TODOs:
# 1. The model takes an random input tensor with shape (1, 3, 8, 8)
# 2. The model outputs a tensor with same HxW size of the input, but C = num_classes
# 3. Print out the model output in numpy format
def __init__(self, num_classes=59):
super(BasicModel, self).__init__()
self.Pool=Pool2D(pool_size=4,pool_stride=4,pool_type='max')#TODO
self.Conv=Conv2D(num_channels=3,num_filters=num_classes,filter_size=1)
def forward(self, inputs):
x = self.Pool(inputs)#TODO
x = fluid.layers.interpolate(x, out_shape=(inputs.shape[2], inputs.shape[3]))
x = self.Conv(x)#TODO
return x
def main():
place = paddle.fluid.CPUPlace()
with fluid.dygraph.guard(place):
model = BasicModel(num_classes=59)
model.eval()
input_data = np.random.rand(1,3,8,8).astype(np.float32)# TODO
print('Input data shape: ', input_data.shape)
input_data = to_variable(input_data)# TODO
output_data = model.forward(input_data)# TODO
output_data = output_data.numpy()# TODO
print('Output data shape: ', output_data.shape)
if __name__ == "__main__":
main()
(需要訓練的基本資料可以評論區留言!)
VGG、PSPNet、U-Net均在此基礎上進行新增修改,未完待續!
相關文章
- 百度飛槳PaddlePaddle影像分割7日學習收穫
- 飛槳paddlepaddle影像分割課程筆記筆記
- 飛漿(paddle)實現機器學習機器學習
- 基於深度學習的影像分割在高德的實踐深度學習
- 基於PaddlePaddle的影像分類實戰 | 深度學習基礎任務教程系列(一)深度學習
- 【影像分割】基於四叉樹影像分割matlabMatlab
- 基於飛槳PaddlePaddle的多種影像分類預訓練模型強勢釋出模型
- 百度飛槳(PaddlePaddle)- 張量(Tensor)
- 基於PaddlePadlle的影像分割の新手入門
- 百度飛槳(PaddlePaddle)-數字識別
- 百度影像分割7日打卡訓練營學習筆記筆記
- 真香!使用飛槳PaddlePaddle2.0高層API高效完成基於VGG16的影像分類任務API
- 關於SCRUM的學習心得Scrum
- 基於PaddlePaddle的詞向量實戰 | 深度學習基礎任務教程系列深度學習
- 百度深度學習平臺PaddlePaddle框架解析深度學習框架
- 基於PaddlePaddle的詞向量實戰 | 深度學習基礎任務教程系列(二)深度學習
- 基於深度學習的醫學影像配準學習筆記2深度學習筆記
- 基於PaddlePaddle的影象分類實戰 | 深度學習基礎任務教程系列(一)深度學習
- 基於PaddlePaddle的圖片分類實戰 | 深度學習基礎任務教程系列深度學習
- 基於深度學習的影像超解析度重建深度學習
- 【百度飛漿】YOLO系列目標檢測演算法詳解YOLO演算法
- 百度飛槳(PaddlePaddle) - PaddleOCR 文字識別簡單使用
- 關於資料結構的學習心得資料結構
- 小年小盤點 百度深度學習PaddlePaddle未來可期深度學習
- 百度正式釋出PaddlePaddle深度強化學習框架PARL強化學習框架
- 超多,超快,超強!百度飛槳釋出工業級影像分割利器PaddleSeg
- 美團如何基於深度學習實現影像的智慧稽核?深度學習
- 基於PaddlePaddle的官方NLP模型總覽模型
- 如何用百度深度學習框架PaddlePaddle實現智慧春聯深度學習框架
- 百度AI攻略:Paddlehub實現影像分割AI
- 使用LabVIEW實現基於pytorch的DeepLabv3影像語義分割ViewPyTorch
- 基於深度學習的影像超解析度重建技術的研究深度學習
- 影像分割中的深度學習:U-Net 體系結構深度學習
- 深入淺出eslint——關於我學習eslint的心得EsLint
- 讀“基於深度學習的影像風格遷移研究綜述”有感深度學習
- 基線,移動視窗,AWR學習心得
- PaddlePaddle車牌識別實戰和心得
- APScheduler 學習心得