在 Linux 多執行緒程式設計中,互斥鎖(Mutex)是一種常用的同步機制,用於保護共享資源,防止多個執行緒同時訪問導致的競爭條件。在 POSIX 執行緒庫中,互斥鎖通常透過 pthread_mutex_t 型別表示,相關的函式包括 pthread_mutex_init、pthread_mutex_lock、pthread_mutex_unlock 等。
下面為一個demo,舉例說明在多執行緒中使用互斥鎖:
#include <stdio.h> #include <pthread.h> #define NUM_THREADS 5 // 共享資源 int shared_resource = 0; // 互斥鎖 pthread_mutex_t mutex; // 執行緒函式 void *thread_function(void *arg) { int thread_id = *((int *)arg); // 加鎖 pthread_mutex_lock(&mutex); // 訪問共享資源 printf("Thread %d: Shared resource before modification: %d\n", thread_id, shared_resource); sleep(1); shared_resource++; printf("Thread %d: Shared resource after modification: %d\n", thread_id, shared_resource); // 解鎖 pthread_mutex_unlock(&mutex); pthread_exit(NULL); } int main() { pthread_t threads[NUM_THREADS]; int thread_args[NUM_THREADS]; int i; // 初始化互斥鎖 pthread_mutex_init(&mutex, NULL); // 建立多個執行緒 for (i = 0; i < NUM_THREADS; ++i) { thread_args[i] = i; pthread_create(&threads[i], NULL, thread_function, (void *)&thread_args[i]); } // 等待所有執行緒完成 for (i = 0; i < NUM_THREADS; ++i) { pthread_join(threads[i], NULL); } // 銷燬互斥鎖 pthread_mutex_destroy(&mutex); return 0; }
執行結果如下:
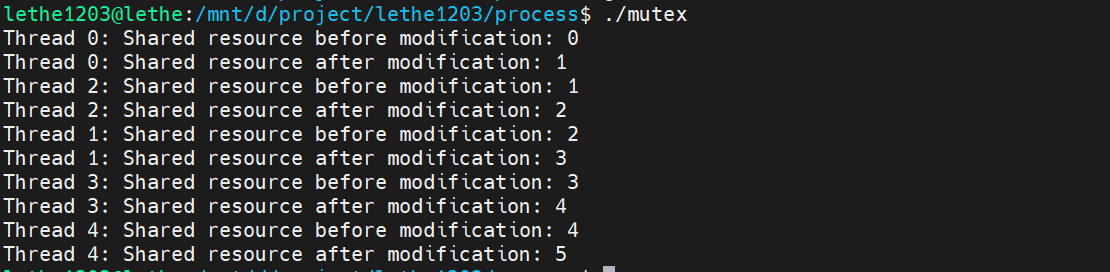