test suite
- 測試套件,理解成測試用例集
- 一系列的測試用例,或測試套件,理解成測試用例的集合和測試套件的集合
- 當執行測試套件時,則執行裡面新增的所有測試用例
test runner
- 測試執行器
- 用於執行和輸出結果的元件
test suite、test runner基礎使用
單元測試類
1 # 建立單元測試類,繼承unittest.TestCase
2 class testCase(unittest.TestCase):
3
4 # 測試case
5 def test_01(self):
6 print("test01")
7
8 def test_03(self):
9 print("test03")
10
11 def test_04(self):
12 print("test04")
13
14 def test_05(self):
15 print("test05")
主函式
1 if __name__ == '__main__':
2 # 例項化測試套件
3 suite = unittest.TestSuite()
4 # 例項化第二個測試套件
5 suite1 = unittest.TestSuite()
6 # 新增測試用例 - 方式一
7 suite.addTest(testCase('test_03'))
8 suite.addTest(testCase('test_01'))
9 suite1.addTest(testCase('test_03'))
10 suite1.addTest(testCase('test_01'))
11 # 新增測試用例 - 方式二
12 testcase = (testCase('test_05'), testCase('test_04'))
13 suite.addTests(testcase)
14 # 測試套件新增測試套件
15 suite.addTest(suite1)
16 # 例項化TextTestRunner類
17 runner = unittest.TextTestRunner()
18 # 執行測試套件
19 runner.run(suite)
執行結果
1 test03
2 test01
3 test05
4 test04
5 test03
6 test01
7 ......
8 ----------------------------------------------------------------------
9 Ran 6 tests in 0.000s
10
11 OK
包含知識點
- 使用測試套件時,測試用例的執行順序可以自定義,按照新增的順序執行
- 有兩種新增測試用例的方式,推薦方式二,程式碼更少更快捷
- ,傳入的 tests 可以是list、tuple、set
addTests(tests)
- 新增的測試用例格式是:
單元測試類名(測試用例名)
- 使用測試套件執行測試用例的大致步驟是:例項化TestSuite - 新增測試用例 - 例項化TextTestRunner - 執行測試套件
- 測試套件也可以新增測試套件
測試用例批次執行
單元測試類檔案
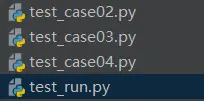
前三個檔案是包含了單元測試類的檔案,第四個檔案是負責執行所有單元測試類,不包含測試用例
列舉某個單元測試類檔案程式碼
1 # 建立單元測試類,繼承unittest.TestCase
2 class testCase02(unittest.TestCase):
3
4 # 測試case
5 def test_07(self):
6 print("testCase02 test07")
7
8 def test_06(self):
9 print("testCase02 test06")
10
11 def test_11(self):
12 print("testCase02 test11")
test_run.py 程式碼
批次執行測試用例方式一:
1 import unittest
2 from learn.unittestLearning import test_case02
3 from learn.unittestLearning.test_case03 import testCase03
4
5 if __name__ == '__main__':
6 # 透過模組
7 testcase02 = unittest.TestLoader().loadTestsFromModule(test_case02)
8 # 透過單元測試類
9 testcase03 = unittest.TestLoader().loadTestsFromTestCase(testCase03)
10 # 透過模組字串
11 testcase04 = unittest.TestLoader().loadTestsFromName('learn.unittestLearning.test_case04')
12 # 測試用例集
13 tests = [testcase02, testcase03, testcase04]
14 # 建立測試套件
15 suite = unittest.TestSuite(tests)
16 # 執行測試套件
17 unittest.TextTestRunner(verbosity=2).run(suite)
包含知識點
- :testCaseClass輸入單元測試類,但需要先import
loadTestsFromTestCase(testCaseClass)
- :module輸入單元測試類所在模組,也需要import
loadTestsFromModule(module, pattern=None)
- :name是一個string,需滿足以下格式: module.class.method ,可以只到輸入到class
loadTestsFromName(name, module=None)
- :表示測試結果的資訊詳細程,一共三個值,預設是1
- 0 (靜默模式):你只能獲得總的測試用例數和總的結果 比如 總共100個 失敗20 成功80
- 1 (預設模式):非常類似靜默模式 只是在每個成功的用例前面有個 每個失敗的用例前面有個 F
- 2 (詳細模式):測試結果會顯示每個測試用例的所有相關的資訊
verbosity
批次執行測試用例方式二(推薦!!):
1 import unittest
2
3 if __name__ == '__main__':
4 # 需要執行的單元測試檔案目錄
5 test_path = './'
6 # 例項化defaultTestLoader
7 discover = unittest.defaultTestLoader.discover(start_dir=test_path, pattern="test_case*.py")
8 # 執行測試用例集
9 unittest.TextTestRunner().run(discover)
優點:是不是簡潔。。是不是很快??只需三行程式碼!!
包含知識點
- :寫需要執行的單元測試檔案目錄
start_dir
- :單元測試檔案的匹配規則,預設是 test*.py ,可根據自己的命名規則修改此正則
pattern
- 方法可自動根據測試目錄start_dir 匹配查詢測試用例檔案 test*.py ,並將查詢到的測試用例組裝到測試套件,因此可以直接透過 run() 方法執行 discover
discover()
批次執行測試用例的結果
1 testCase02 test06
2 testCase02 test07
3 testCase02 test11
4 testCase03 test05
5 testCase03 test08
6 testCase03 test12
7 testCase04 test02
8 testCase04 test04
9 testCase04 test13
10 .........
11 ----------------------------------------------------------------------
12 Ran 9 tests in 0.000s
13
14 OK
最後感謝每一個認真閱讀我文章的人,禮尚往來總是要有的,這些資料,對於【軟體測試】的朋友來說應該是最全面最完整的備戰倉庫,雖然不是什麼很值錢的東西,如果你用得到的話可以直接拿走: