Java Filter過濾器
一:過濾器簡介
Filter也稱之為過濾器,它是Servlet技術中最實用的技術,Web開發人員透過Filter技術,對web伺服器管理的所有web資源:例如Jsp, Servlet, 靜態圖片檔案或靜態 html 檔案等進行攔截,從而實現一些特殊的功能。例如實現URL級別的許可權訪問控制、過濾敏感詞彙、壓縮響應資訊等一些高階功能。
它主要用於對使用者請求進行預處理,也可以對HttpServletResponse進行後處理。使用Filter的完整流程:Filter對使用者請求進行預處理,接著將請求交給Servlet進行處理並生成響應,最後Filter再對伺服器響應進行後處理。
二:過濾器功能
在HttpServletRequest到達 Servlet 之前,攔截客戶的HttpServletRequest 。根據需要檢查HttpServletRequest,也可以修改HttpServletRequest 頭和資料。
在HttpServletResponse到達客戶端之前,攔截HttpServletResponse 。根據需要檢查HttpServletResponse,也可以修改HttpServletResponse頭和資料。
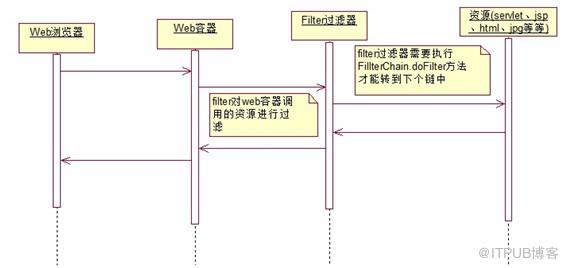
三:過濾器開發
Filter介面中有一個doFilter方法,當開發人員編寫好Filter,並配置對哪個web資源進行攔截後,Web伺服器每次在呼叫web資源的service方法之前,都會先呼叫一下filter的doFilter方法,因此,在該方法內編寫程式碼可達到如下目的:
呼叫目標資源之前,讓一段程式碼執行。 是否呼叫目標資源(即是否讓使用者訪問web資源)。 web伺服器在呼叫doFilter方法時,會傳遞一個filterChain物件進來,filterChain物件是filter介面中最重要的一個物件,它也提供了一個doFilter方法,開發人員可以根據需求決定是否呼叫此方法,呼叫該方法,則web伺服器就會呼叫web資源的service方法,即web資源就會被訪問,否則web資源不會被訪問。
在一個web應用中,可以開發編寫多個Filter,這些Filter組合起來稱之為一個Filter鏈。
web伺服器根據Filter在web.xml檔案中的註冊順序,決定先呼叫哪個Filter,當第一個Filter的doFilter方法被呼叫時,web伺服器會建立一個代表Filter鏈的FilterChain物件傳遞給該方法。在doFilter方法中,開發人員如果呼叫了FilterChain物件的doFilter方法,則web伺服器會檢查FilterChain物件中是否還有filter,如果有,則呼叫第2個filter,如果沒有,則呼叫目標資源。
Filter的生命週期
public void init(FilterConfig filterConfig) throws ServletException;//初始化
和我們編寫的Servlet程式一樣,Filter的建立和銷燬由WEB伺服器負責。 web 應用程式啟動時,web 伺服器將建立Filter 的例項物件,並呼叫其init方法,讀取web.xml配置,完成物件的初始化功能,從而為後續的使用者請求作好攔截的準備工作(filter物件只會建立一次,init方法也只會執行一次)。開發人員透過init方法的引數,可獲得代表當前filter配置資訊的FilterConfig物件。
public void doFilter(ServletRequest request, ServletResponse response, FilterChain CHAIN) throws IOException, ServletException;//攔截請求
這個方法完成實際的過濾操作。當客戶請求訪問與過濾器關聯的URL的時候,Servlet過濾器將先執行doFilter方法。FilterChain引數用於訪問後續過濾器。
public void destroy();//銷燬
Filter物件建立後會駐留在記憶體,當web應用移除或伺服器停止時才銷燬。在Web容器解除安裝 Filter 物件之前被呼叫。該方法在Filter的生命週期中僅執行一次。在這個方法中,可以釋放過濾器使用的資源。
FilterConfig介面
使用者在配置filter時,可以使用為filter配置一些初始化引數,當web容器例項化Filter物件,呼叫其init方法時,會把封裝了filter初始化引數的filterConfig物件傳遞進來。因此開發人員在編寫filter時,透過filterConfig物件的方法,就可獲得以下內容:
STRING getFilterName();//得到filter的名稱。
STRING getInitParameter(STRING NAME);//返回在部署描述中指定名稱的初始化引數的值。如果不存在返回null.
Enumeration getInitParameterNames();//返回過濾器的所有初始化引數的名字的列舉集合。
public ServletContext getServletContext();//返回Servlet上下文物件的引用。
四:過濾器例項
本例項的作用是使頁面支援gzip壓縮資源請求(Content-Encoding:gzip)
web.xml配置
程式碼:
Filter也稱之為過濾器,它是Servlet技術中最實用的技術,Web開發人員透過Filter技術,對web伺服器管理的所有web資源:例如Jsp, Servlet, 靜態圖片檔案或靜態 html 檔案等進行攔截,從而實現一些特殊的功能。例如實現URL級別的許可權訪問控制、過濾敏感詞彙、壓縮響應資訊等一些高階功能。
它主要用於對使用者請求進行預處理,也可以對HttpServletResponse進行後處理。使用Filter的完整流程:Filter對使用者請求進行預處理,接著將請求交給Servlet進行處理並生成響應,最後Filter再對伺服器響應進行後處理。
二:過濾器功能
在HttpServletRequest到達 Servlet 之前,攔截客戶的HttpServletRequest 。根據需要檢查HttpServletRequest,也可以修改HttpServletRequest 頭和資料。
在HttpServletResponse到達客戶端之前,攔截HttpServletResponse 。根據需要檢查HttpServletResponse,也可以修改HttpServletResponse頭和資料。
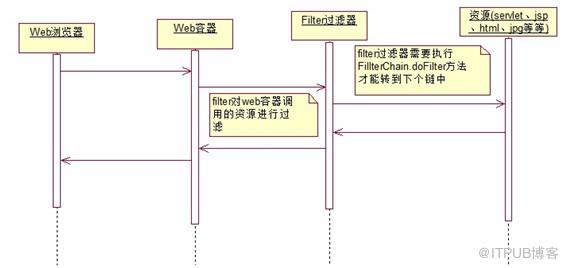
三:過濾器開發
點選(此處)摺疊或開啟
-
public interface Filter {
-
-
/**
-
* Called by the web container to indicate to a filter that it is being placed into
-
* service. The servlet container calls the init method exactly once after instantiating the
-
* filter. The init method must complete successfully before the filter is asked to do any
-
* filtering work. <br><br>
-
-
* The web container cannot place the filter into service if the init method either<br>
-
* 1.Throws a ServletException <br>
-
* 2.Does not return within a time period defined by the web container
-
*/
-
public void init(FilterConfig filterConfig) throws ServletException;
-
-
-
/**
-
* The <code>doFilter</code> method of the Filter is called by the container
-
* each time a request/response pair is passed through the chain due
-
* to a client request for a resource at the end of the chain. The FilterChain passed in to this
-
* method allows the Filter to pass on the request and response to the next entity in the
-
* chain.
-
* A typical implementation of this method would follow the following pattern:- <br>
-
* 1. Examine the request<br>
-
* 2. Optionally wrap the request object with a custom implementation to
-
* filter content or headers for input filtering <br>
-
* 3. Optionally wrap the response object with a custom implementation to
-
* filter content or headers for output filtering <br>
-
* 4. a) Either invoke the next entity in the chain using the FilterChain object (<code>chain.doFilter()</code>), <br>
-
** 4. b) or not pass on the request/response pair to the next entity in the filter chain to block the request processing<br>
-
** 5. Directly set headers on the response after invocation of the next entity in the filter chain.
-
**/
-
public void doFilter ( ServletRequest request, ServletResponse response, FilterChain chain ) throws IOException, ServletException;
-
-
/**
-
* Called by the web container to indicate to a filter that it is being taken out of service. This
-
* method is only called once all threads within the filter's doFilter method have exited or after
-
* a timeout period has passed. After the web container calls this method, it will not call the
-
* doFilter method again on this instance of the filter. <br><br>
-
*
-
* This method gives the filter an opportunity to clean up any resources that are being held (for
-
* example, memory, file handles, threads) and make sure that any persistent state is synchronized
-
* with the filter's current state in memory.
-
*/
-
-
public void destroy();
-
-
- }
呼叫目標資源之前,讓一段程式碼執行。 是否呼叫目標資源(即是否讓使用者訪問web資源)。 web伺服器在呼叫doFilter方法時,會傳遞一個filterChain物件進來,filterChain物件是filter介面中最重要的一個物件,它也提供了一個doFilter方法,開發人員可以根據需求決定是否呼叫此方法,呼叫該方法,則web伺服器就會呼叫web資源的service方法,即web資源就會被訪問,否則web資源不會被訪問。
在一個web應用中,可以開發編寫多個Filter,這些Filter組合起來稱之為一個Filter鏈。
web伺服器根據Filter在web.xml檔案中的註冊順序,決定先呼叫哪個Filter,當第一個Filter的doFilter方法被呼叫時,web伺服器會建立一個代表Filter鏈的FilterChain物件傳遞給該方法。在doFilter方法中,開發人員如果呼叫了FilterChain物件的doFilter方法,則web伺服器會檢查FilterChain物件中是否還有filter,如果有,則呼叫第2個filter,如果沒有,則呼叫目標資源。
Filter的生命週期
public void init(FilterConfig filterConfig) throws ServletException;//初始化
和我們編寫的Servlet程式一樣,Filter的建立和銷燬由WEB伺服器負責。 web 應用程式啟動時,web 伺服器將建立Filter 的例項物件,並呼叫其init方法,讀取web.xml配置,完成物件的初始化功能,從而為後續的使用者請求作好攔截的準備工作(filter物件只會建立一次,init方法也只會執行一次)。開發人員透過init方法的引數,可獲得代表當前filter配置資訊的FilterConfig物件。
public void doFilter(ServletRequest request, ServletResponse response, FilterChain CHAIN) throws IOException, ServletException;//攔截請求
這個方法完成實際的過濾操作。當客戶請求訪問與過濾器關聯的URL的時候,Servlet過濾器將先執行doFilter方法。FilterChain引數用於訪問後續過濾器。
public void destroy();//銷燬
Filter物件建立後會駐留在記憶體,當web應用移除或伺服器停止時才銷燬。在Web容器解除安裝 Filter 物件之前被呼叫。該方法在Filter的生命週期中僅執行一次。在這個方法中,可以釋放過濾器使用的資源。
FilterConfig介面
使用者在配置filter時,可以使用為filter配置一些初始化引數,當web容器例項化Filter物件,呼叫其init方法時,會把封裝了filter初始化引數的filterConfig物件傳遞進來。因此開發人員在編寫filter時,透過filterConfig物件的方法,就可獲得以下內容:
STRING getFilterName();//得到filter的名稱。
STRING getInitParameter(STRING NAME);//返回在部署描述中指定名稱的初始化引數的值。如果不存在返回null.
Enumeration getInitParameterNames();//返回過濾器的所有初始化引數的名字的列舉集合。
public ServletContext getServletContext();//返回Servlet上下文物件的引用。
四:過濾器例項
本例項的作用是使頁面支援gzip壓縮資源請求(Content-Encoding:gzip)
web.xml配置
點選(此處)摺疊或開啟
-
<filter>
-
<filter-name>GzipJsFilter</filter-name>
-
<filter-class>com.gemdale.gmap.manager.web.filter.GzipJsFilter</filter-class>
-
<init-param>
-
<param-name>headers</param-name>
-
<param-value>Content-Encoding=gzip</param-value>
-
</init-param>
-
</filter>
-
<filter-mapping>
-
<filter-name>GzipJsFilter</filter-name>
-
<url-pattern>*.gzjs</url-pattern>
- </filter-mapping>
點選(此處)摺疊或開啟
-
public class GzipJsFilter implements Filter {
-
Map headers = new HashMap();
-
-
public void destroy() {
-
}
-
-
public void doFilter(ServletRequest req, ServletResponse res, FilterChain chain)
-
throws IOException, ServletException {
-
if (req instanceof HttpServletRequest) {
-
doFilter((HttpServletRequest) req, (HttpServletResponse) res, chain);
-
}
-
else {
-
chain.doFilter(req, res);
-
}
-
}
-
-
public void doFilter(HttpServletRequest request, HttpServletResponse response, FilterChain chain)
-
throws IOException, ServletException {
-
request.setCharacterEncoding("UTF-8");
-
for (Iterator it = headers.entrySet().iterator(); it.hasNext();) {
-
Map.Entry entry = (Map.Entry) it.next();
-
response.addHeader((String) entry.getKey(), (String) entry.getValue());
-
}
-
chain.doFilter(request, response);
-
}
-
-
public void init(FilterConfig config) throws ServletException {
-
String headersStr = config.getInitParameter("headers");
-
String[] headers = headersStr.split(",");
-
for (int i = 0; i < headers.length; i++) {
-
String[] temp = headers[i].split("=");
-
this.headers.put(temp[0].trim(), temp[1].trim());
-
}
-
}
- }
來自 “ ITPUB部落格 ” ,連結:http://blog.itpub.net/28624388/viewspace-2129713/,如需轉載,請註明出處,否則將追究法律責任。
相關文章
- Filter過濾器Filter過濾器
- PHP 過濾器(Filter)PHP過濾器Filter
- lucene Filter過濾器Filter過濾器
- Java 中的 Filter 過濾器詳解JavaFilter過濾器
- 【Java基礎】--filter過濾器原理解析JavaFilter過濾器
- Filter過濾器的使用Filter過濾器
- JavaWeb 中 Filter過濾器JavaWebFilter過濾器
- 布隆過濾器(Bloom Filter)的java實現過濾器OOMFilterJava
- 布隆過濾器(Bloom Filter)過濾器OOMFilter
- 布隆過濾器 Bloom Filter過濾器OOMFilter
- Bloom Filter 布隆過濾器OOMFilter過濾器
- servlet的過濾器filter類Servlet過濾器Filter
- angular內建過濾器-filterAngular過濾器Filter
- filter過濾Filter
- 攔截器(Interceptor)與過濾器(Filter)過濾器Filter
- java中listFiles(Filefilter filter)檔案過濾器的實現過程JavaFilter過濾器
- Vue定義全域性過濾器filterVue過濾器Filter
- HBase Filter 過濾器之 ValueFilter 詳解Filter過濾器
- 布隆過濾器(Bloom Filter)詳解過濾器OOMFilter
- 如何在vue中使用過濾器filterVue過濾器Filter
- 走進AngularJs(七) 過濾器(filter)AngularJS過濾器Filter
- Filter(過濾器)與Listener(監聽器)詳解Filter過濾器
- java8 多條件的filter過濾JavaFilter
- AngularJS教程二十一—— 過濾器(filter)AngularJS過濾器Filter
- 【SSO】--單點登入之過濾器(filter)過濾器Filter
- 過濾器 Filter 與 攔截器 Interceptor 的區別過濾器Filter
- Elasticsearch——filter過濾查詢ElasticsearchFilter
- JavaWeb - 【Filter】敏感詞過濾JavaWebFilter
- Java Servlet (1) —— Filter過濾請求與響應JavaServletFilter
- 微信小程式 使用filter過濾器幾種方式微信小程式Filter過濾器
- 探索C#之布隆過濾器(Bloom filter)C#過濾器OOMFilter
- Laravel 模型過濾(Filter)設計Laravel模型Filter
- OGG -FILTER 引數過濾Filter
- Filter不過濾CSS和JSFilterCSSJS
- Solon 的過濾器 Filter 和兩種攔截器 Handler、 Interceptor過濾器Filter
- Web中的監聽器【Listener】與過濾器【Filter】 例項Web過濾器Filter
- OGG 行過濾filter 引數Filter
- Django(69)最好用的過濾器外掛Django-filterDjango過濾器Filter