C語言 將字串按照指定字元分離並且反轉(三級指標)列子
C語言 將字串分離並且反轉(三級指標)
本程式完成功能
1、將輸入的字串按照指定字元分離為子字串
2、將子字串進行反轉
使用方法
在棧空間分配一個三級指標,指向堆記憶體空間的指標陣列的位置,每個指標陣列成員又指向一個字串,必須明確如下的
記憶體四區圖這裡只畫最為複雜的分離字元函式,而不畫反轉函式,因為反轉函式模型非常簡單,而且畫太多太麻煩。
在圖中反應出strsplrev中的int len和 char** str為輸出變數,也就是說str用來接受malloc出來的二級指標的值,那麼我們應該
將三級指標傳入,另外argv輸入的字串放到了全域性區,這個區域是不能更改的,所以用一個堆instr將他接收過來,這也是典
型的場景下圖(圖中將i m p3個棧變數放到了一起),重點在於堆中的記憶體理解問題。
程式輸出如下:
gaopeng@bogon:~/stepup/3rd$ ./splitstr oracle-mysql-mongodb -
----len is 3
oracle
mysql
mongodb
----reverse now!
elcaro
lqsym
bdognom
----free mem!
四區圖如下:
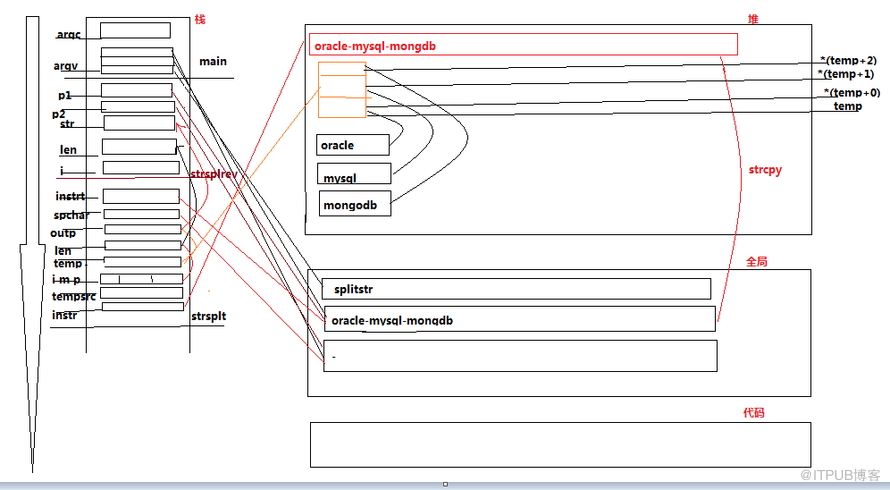
最後給出實現程式碼:
本程式完成功能
1、將輸入的字串按照指定字元分離為子字串
2、將子字串進行反轉
使用方法
在棧空間分配一個三級指標,指向堆記憶體空間的指標陣列的位置,每個指標陣列成員又指向一個字串,必須明確如下的
記憶體四區圖這裡只畫最為複雜的分離字元函式,而不畫反轉函式,因為反轉函式模型非常簡單,而且畫太多太麻煩。
在圖中反應出strsplrev中的int len和 char** str為輸出變數,也就是說str用來接受malloc出來的二級指標的值,那麼我們應該
將三級指標傳入,另外argv輸入的字串放到了全域性區,這個區域是不能更改的,所以用一個堆instr將他接收過來,這也是典
型的場景下圖(圖中將i m p3個棧變數放到了一起),重點在於堆中的記憶體理解問題。
程式輸出如下:
gaopeng@bogon:~/stepup/3rd$ ./splitstr oracle-mysql-mongodb -
----len is 3
oracle
mysql
mongodb
----reverse now!
elcaro
lqsym
bdognom
----free mem!
四區圖如下:
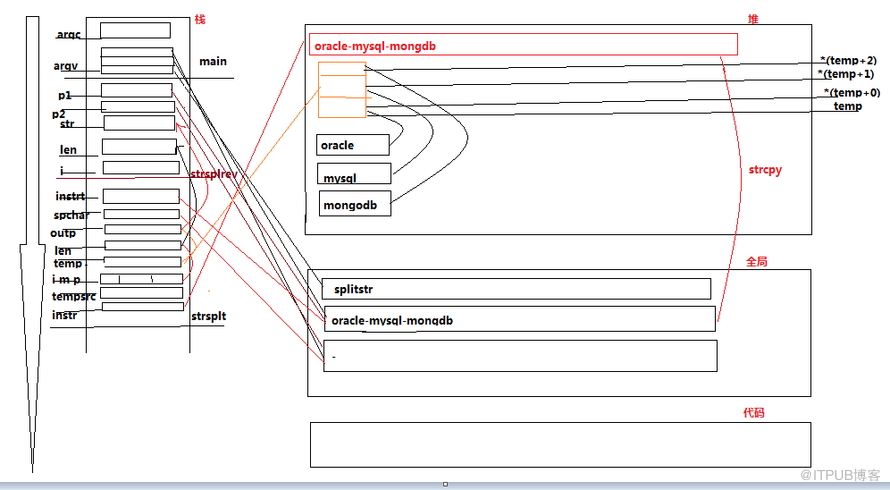
最後給出實現程式碼:
點選(此處)摺疊或開啟
-
介面標頭檔案:
-
/*************************************************************************
-
> File Name: fun.h
-
> Author: gaopeng QQ:22389860 all right reserved
-
> Mail: gaopp_200217@163.com
-
> Created Time: Tue 24 Jan 2017 03:50:07 PM CST
-
************************************************************************/
-
-
-
int strsplt(const char* instrt/*in*/,const char* spchar/*in*/,char** *outp/*out*/,int* len/*out*/);
-
int strrevs(char* instrt/*in out*/);
-
int strsplrev(char *p1,char *p2);
-
-
介面實現檔案:
-
/*************************************************************************
-
> File Name: fun.c
-
> Author: gaopeng QQ:22389860 all right reserved
-
> Mail: gaopp_200217@163.com
-
> Created Time: Tue 24 Jan 2017 02:02:20 PM CST
-
************************************************************************/
-
-
#include<stdio.h>
-
#include<stdlib.h>
-
#include<string.h>
-
-
int mfree(char** *freep,int len)
-
{
-
int i=0;
-
if(*freep==NULL)
-
{
-
return 0;
-
}
-
for(i=0;i<len;i++)
-
{
-
if(*(*freep+i) !=NULL)
-
{
-
printf("%d ",i);
-
free(*(*freep+i));
-
*(*freep+i)=NULL;
-
}
-
}
-
free(*freep);
-
freep = NULL;
-
return 0;
-
}
-
-
-
/*-1 flase 0 ture*/
-
int strsplt( char* instr,const char* spchar,char** *outp,int* len )
-
{
-
char** temp=NULL;
-
int i=0,m=0,p=0;
-
int templen=0;
-
char* tempsrc=NULL;
-
-
if(instr==NULL||spchar==NULL||outp==NULL||len==NULL)
-
{
-
printf("strsplt error:instr==NULL||spchar==NULL||outp==NULL||len==NULL\n");
-
goto err;
-
}
-
p = 1;
-
for(i=0;i<strlen(instr);i++)
-
{
-
if(instr[i]==spchar[0])
-
{
-
if (i == strlen(instr)-1)
-
{
-
printf("strsplt error:last char is spchar \n");
-
goto err;
-
}
-
if(instr[i+1]==spchar[0])
-
{
-
printf("strsplt error:two or more spchar \n");
-
goto err;
-
}
-
-
p++;
-
}
-
}
-
-
*len = p;
-
templen = p;//used mfree fun
-
-
if((temp=(char**)calloc(1,p*sizeof(char*)))==NULL)
-
{
-
printf("strsplt error:mem calloc error\n");
-
goto err;
-
}
-
*outp=temp;
-
for(i=0,p=0,m=0;i<strlen(instr);i++)//i is step p is step of pointer m is len of substr
-
{
-
if(instr[i]==spchar[0])
-
{
-
tempsrc=instr+m;
-
if( (*(temp+p)= (char*)calloc(1,i-m+1))==NULL)
-
{
-
printf("strsplt error:mem calloc error\n");
-
goto err;
-
}
-
strncpy(*(temp+p),tempsrc,i-m);
-
p++;
-
m=i+1;
-
}
-
}
-
//last copy
-
tempsrc=instr+m;
-
if( (*(temp+p)= (char*)calloc(1,i-m+1)) == NULL)
-
{
-
printf("strsplt error:mem calloc error\n");
-
goto err;
-
}
-
strncpy(*(temp+p),tempsrc,i-m);
-
return 0;
-
-
err:
-
mfree(&temp,templen);
-
return -1;
-
-
}
-
-
/* -1 false 0 true*/
-
int strrevs(char* instrt)
-
{
-
int templ=0;
-
int i=0,j=0;
-
char tempc=0;
-
templ = strlen(instrt);
-
if(instrt == NULL || templ==0)
-
{
-
printf("strrevs error:instrt == NULL || templ=0\n");
-
return -1;
-
}
-
for(i=0,j=templ-1;i<j;i++,j--)
-
{
-
tempc=instrt[j];
-
instrt[j]=instrt[i];
-
instrt[i]=tempc;
-
}
-
return 0;
-
}
-
-
int strsplrev(char *p1,char *p2)
-
{
-
-
char* *str=NULL;
-
int slen=0;
-
int i=0;
-
-
if(strsplt(p1,p2,&str,&slen) == 0)
-
{
-
printf("----len is %d\n",slen);
-
for(i=0;i<slen;i++)
-
{
-
printf("%s\n",*(str+i));
-
}
-
}
-
else
-
{
-
printf("error!\n");
-
return 3;
-
}
-
-
printf("----reverse now!\n");
-
for(i=0;i<slen;i++)
-
{
-
-
if(strrevs(*(str+i)) !=0)
-
{
-
-
printf("error!\n");
-
return 4;
-
}
-
else
-
{
-
-
printf("%s\n",*(str+i));
-
}
-
}
-
printf("----free mem!\n");
-
mfree(&str,slen);
-
return 0;
-
}
-
-
main呼叫檔案:
-
/*************************************************************************
-
> File Name: main.c
-
> Author: gaopeng QQ:22389860 all right reserved
-
> Mail: gaopp_200217@163.com
-
> Created Time: Tue 24 Jan 2017 02:02:09 PM CST
-
************************************************************************/
-
-
#include<stdio.h>
-
#include<stdlib.h>
-
#include<string.h>
-
#include"fun.h"
-
-
-
int main(int argc,char* argv[])
-
{
-
-
if(argc!=3||strcmp(argv[1],"--help")==0)
-
{
-
printf("usage ./tool str spchar \n");
-
return 1;
-
}
-
-
if(strlen(argv[2]) !=1)
-
{
-
printf("par2 split char only one char\n");
-
return 2;
-
}
-
-
strsplrev(argv[1],argv[2]);
-
return 0;
-
-
- }
來自 “ ITPUB部落格 ” ,連結:http://blog.itpub.net/7728585/viewspace-2131812/,如需轉載,請註明出處,否則將追究法律責任。
相關文章
- C語言指標(三):陣列指標和字串指標C語言指標陣列字串
- C語言知識彙總 | 51-C語言字串指標(指向字串的指標)C語言字串指標
- 如何將一個數字轉換為字串並且按照指定格式顯示?--TO_CHAR字串
- C語言學習之:指標與字串C語言指標字串
- C語言指標5分鐘教程C語言指標
- C 語言指標 5 分鐘教程指標
- C語言 C語言野指標C語言指標
- C語言(指標)C語言指標
- C語言指標C語言指標
- 字串指標與字元陣列 (轉)字串指標字元陣列
- C語言:使用指標將兩段字串連線起來輸出C語言指標字串
- c/c++ c語言字元與字串C++C語言字元字串
- C語言-指標操作C語言指標
- C語言之字串與指標C語言字串指標
- C語言sizeof()變數、字元、字串C語言變數字元字串
- C語言:利用指標檢查字串是否是迴文C語言指標字串
- 【c語言】把一個長整型給一個字元指標C語言字元指標
- 將一個字串進行反轉:將字串中指定部分進行反轉。比如“abcdefg”反轉為”abfedcg”字串
- C語言指標(二) 指標變數 ----by xhxhC語言指標變數
- c語言指標彙總C語言指標
- C語言指標學習C語言指標
- c語言指標詳解C語言指標
- C語言指標筆記C語言指標筆記
- C語言基礎-指標C語言指標
- C語言 函式指標C語言函式指標
- C語言:指標,C的靈魂C語言指標
- C語言指標安全及指標使用問題C語言指標
- 搞清楚C語言指標C語言指標
- C語言 指標與陣列C語言指標陣列
- C語言指標基本知識C語言指標
- C語言指標詳解(一)C語言指標
- C語言指標詳解(二)C語言指標
- C語言指標用法大全C語言指標
- c語言實現this指標效果C語言指標
- C語言指標細節_1C語言指標
- 指標——C語言的靈魂指標C語言
- C語言基礎-1、指標C語言指標
- C# 移除字串末尾指定字元C#字串字元