JDBC批量Insert深度優化(有事務)
環境:
MySQL 5.1
RedHat Linux AS 5
JavaSE 1.5
DbConnectionBroker 微型資料庫連線池
測試的方案:
執行10萬次Insert語句,使用不同方式。
A組:靜態SQL,自動提交,沒事務控制(MyISAM引擎)
1、逐條執行10萬次
2、分批執行將10萬分成m批,每批n條,分多種分批方案來執行。
B組:預編譯模式SQL,自動提交,沒事務控制(MyISAM引擎)
1、逐條執行10萬次
2、分批執行將10萬分成m批,每批n條,分多種分批方案來執行。
-------------------------------------------------------------------------------------------
C組:靜態SQL,不自動提交,有事務控制(InnoDB引擎)
1、逐條執行10萬次
2、分批執行將10萬分成m批,每批n條,分多種分批方案來執行。
D組:預編譯模式SQL,不自動提交,有事務控制(InnoDB引擎)
1、逐條執行10萬次
2、分批執行將10萬分成m批,每批n條,分多種分批方案來執行。
本次主要測試C、D組,並得出測試結果。
SQL程式碼
DROP TABLE IF EXISTS tuser;
CREATE TABLE tuser (
id bigint(20) NOT NULL AUTO_INCREMENT,
name varchar(12) DEFAULT NULL,
remark varchar(24) DEFAULT NULL,
createtime datetime DEFAULT NULL,
updatetime datetime DEFAULT NULL,
PRIMARY KEY (id)
) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=utf8;
CREATE TABLE tuser (
id bigint(20) NOT NULL AUTO_INCREMENT,
name varchar(12) DEFAULT NULL,
remark varchar(24) DEFAULT NULL,
createtime datetime DEFAULT NULL,
updatetime datetime DEFAULT NULL,
PRIMARY KEY (id)
) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=utf8;
C、D組測試程式碼:
package testbatch;
import java.io.IOException;
import java.sql.*;
/**
* JDBC批量Insert優化(下)
*
* @author leizhimin 2009-7-29 10:03:10
*/
public class TestBatch {
public static DbConnectionBroker myBroker = null;
static {
try {
myBroker = new DbConnectionBroker("com.mysql.jdbc.Driver",
"jdbc:mysql://192.168.104.163:3306/testdb",
"vcom", "vcom", 2, 4,
"c:\\testdb.log", 0.01);
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* 初始化測試環境
*
* @throws SQLException 異常時丟擲
*/
public static void init() throws SQLException {
Connection conn = myBroker.getConnection();
conn.setAutoCommit(false);
Statement stmt = conn.createStatement();
stmt.addBatch("DROP TABLE IF EXISTS tuser");
stmt.addBatch("CREATE TABLE tuser (\n" +
" id bigint(20) NOT NULL AUTO_INCREMENT,\n" +
" name varchar(12) DEFAULT NULL,\n" +
" remark varchar(24) DEFAULT NULL,\n" +
" createtime datetime DEFAULT NULL,\n" +
" updatetime datetime DEFAULT NULL,\n" +
" PRIMARY KEY (id)\n" +
") ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=utf8");
stmt.executeBatch();
conn.commit();
myBroker.freeConnection(conn);
}
/**
* 100000條靜態SQL插入
*
* @throws Exception 異常時丟擲
*/
public static void testInsert() throws Exception {
init(); //初始化環境
Long start = System.currentTimeMillis();
for (int i = 0; i < 100000; i++) {
String sql = "\n" +
"insert into testdb.tuser \n" +
"\t(name, \n" +
"\tremark, \n" +
"\tcreatetime, \n" +
"\tupdatetime\n" +
"\t)\n" +
"\tvalues\n" +
"\t('" + RandomToolkit.generateString(12) + "', \n" +
"\t'" + RandomToolkit.generateString(24) + "', \n" +
"\tnow(), \n" +
"\tnow()\n" +
")";
Connection conn = myBroker.getConnection();
conn.setAutoCommit(false);
Statement stmt = conn.createStatement();
stmt.execute(sql);
conn.commit();
myBroker.freeConnection(conn);
}
Long end = System.currentTimeMillis();
System.out.println("單條執行100000條Insert操作,共耗時:" + (end - start) / 1000f + "秒!");
}
/**
* 批處理執行靜態SQL測試
*
* @param m 批次
* @param n 每批數量
* @throws Exception 異常時丟擲
*/
public static void testInsertBatch(int m, int n) throws Exception {
init(); //初始化環境
Long start = System.currentTimeMillis();
for (int i = 0; i < m; i++) {
//從池中獲取連線
Connection conn = myBroker.getConnection();
conn.setAutoCommit(false);
Statement stmt = conn.createStatement();
for (int k = 0; k < n; k++) {
String sql = "\n" +
"insert into testdb.tuser \n" +
"\t(name, \n" +
"\tremark, \n" +
"\tcreatetime, \n" +
"\tupdatetime\n" +
"\t)\n" +
"\tvalues\n" +
"\t('" + RandomToolkit.generateString(12) + "', \n" +
"\t'" + RandomToolkit.generateString(24) + "', \n" +
"\tnow(), \n" +
"\tnow()\n" +
")";
//加入批處理
stmt.addBatch(sql);
}
stmt.executeBatch(); //執行批處理
conn.commit();
// stmt.clearBatch(); //清理批處理
stmt.close();
myBroker.freeConnection(conn); //連線歸池
}
Long end = System.currentTimeMillis();
System.out.println("批量執行" + m + "*" + n + "=" + m * n + "條Insert操作,共耗時:" + (end - start) / 1000f + "秒!");
}
/**
* 100000條預定義SQL插入
*
* @throws Exception 異常時丟擲
*/
public static void testInsert2() throws Exception { //單條執行100000條Insert操作,共耗時:40.422秒!
init(); //初始化環境
Long start = System.currentTimeMillis();
String sql = "" +
"insert into testdb.tuser\n" +
" (name, remark, createtime, updatetime)\n" +
"values\n" +
" (?, ?, ?, ?)";
for (int i = 0; i < 100000; i++) {
Connection conn = myBroker.getConnection();
conn.setAutoCommit(false);
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, RandomToolkit.generateString(12));
pstmt.setString(2, RandomToolkit.generateString(24));
pstmt.setDate(3, new Date(System.currentTimeMillis()));
pstmt.setDate(4, new Date(System.currentTimeMillis()));
pstmt.executeUpdate();
conn.commit();
pstmt.close();
myBroker.freeConnection(conn);
}
Long end = System.currentTimeMillis();
System.out.println("單條執行100000條Insert操作,共耗時:" + (end - start) / 1000f + "秒!");
}
/**
* 批處理執行預處理SQL測試
*
* @param m 批次
* @param n 每批數量
* @throws Exception 異常時丟擲
*/
public static void testInsertBatch2(int m, int n) throws Exception {
init(); //初始化環境
Long start = System.currentTimeMillis();
String sql = "" +
"insert into testdb.tuser\n" +
" (name, remark, createtime, updatetime)\n" +
"values\n" +
" (?, ?, ?, ?)";
for (int i = 0; i < m; i++) {
//從池中獲取連線
Connection conn = myBroker.getConnection();
conn.setAutoCommit(false);
PreparedStatement pstmt = conn.prepareStatement(sql);
for (int k = 0; k < n; k++) {
pstmt.setString(1, RandomToolkit.generateString(12));
pstmt.setString(2, RandomToolkit.generateString(24));
pstmt.setDate(3, new Date(System.currentTimeMillis()));
pstmt.setDate(4, new Date(System.currentTimeMillis()));
//加入批處理
pstmt.addBatch();
}
pstmt.executeBatch(); //執行批處理
conn.commit();
// pstmt.clearBatch(); //清理批處理
pstmt.close();
myBroker.freeConnection(conn); //連線歸池
}
Long end = System.currentTimeMillis();
System.out.println("批量執行" + m + "*" + n + "=" + m * n + "條Insert操作,共耗時:" + (end - start) / 1000f + "秒!");
}
public static void main(String[] args) throws Exception {
init();
Long start = System.currentTimeMillis();
System.out.println("--------C組測試----------");
testInsert();
testInsertBatch(100, 1000);
testInsertBatch(250, 400);
testInsertBatch(400, 250);
testInsertBatch(500, 200);
testInsertBatch(1000, 100);
testInsertBatch(2000, 50);
testInsertBatch(2500, 40);
testInsertBatch(5000, 20);
Long end1 = System.currentTimeMillis();
System.out.println("C組測試過程結束,全部測試耗時:" + (end1 - start) / 1000f + "秒!");
System.out.println("--------D組測試----------");
testInsert2();
testInsertBatch2(100, 1000);
testInsertBatch2(250, 400);
testInsertBatch2(400, 250);
testInsertBatch2(500, 200);
testInsertBatch2(1000, 100);
testInsertBatch2(2000, 50);
testInsertBatch2(2500, 40);
testInsertBatch2(5000, 20);
Long end2 = System.currentTimeMillis();
System.out.println("D組測試過程結束,全部測試耗時:" + (end2 - end1) / 1000f + "秒!");
}
}
import java.io.IOException;
import java.sql.*;
/**
* JDBC批量Insert優化(下)
*
* @author leizhimin 2009-7-29 10:03:10
*/
public class TestBatch {
public static DbConnectionBroker myBroker = null;
static {
try {
myBroker = new DbConnectionBroker("com.mysql.jdbc.Driver",
"jdbc:mysql://192.168.104.163:3306/testdb",
"vcom", "vcom", 2, 4,
"c:\\testdb.log", 0.01);
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* 初始化測試環境
*
* @throws SQLException 異常時丟擲
*/
public static void init() throws SQLException {
Connection conn = myBroker.getConnection();
conn.setAutoCommit(false);
Statement stmt = conn.createStatement();
stmt.addBatch("DROP TABLE IF EXISTS tuser");
stmt.addBatch("CREATE TABLE tuser (\n" +
" id bigint(20) NOT NULL AUTO_INCREMENT,\n" +
" name varchar(12) DEFAULT NULL,\n" +
" remark varchar(24) DEFAULT NULL,\n" +
" createtime datetime DEFAULT NULL,\n" +
" updatetime datetime DEFAULT NULL,\n" +
" PRIMARY KEY (id)\n" +
") ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=utf8");
stmt.executeBatch();
conn.commit();
myBroker.freeConnection(conn);
}
/**
* 100000條靜態SQL插入
*
* @throws Exception 異常時丟擲
*/
public static void testInsert() throws Exception {
init(); //初始化環境
Long start = System.currentTimeMillis();
for (int i = 0; i < 100000; i++) {
String sql = "\n" +
"insert into testdb.tuser \n" +
"\t(name, \n" +
"\tremark, \n" +
"\tcreatetime, \n" +
"\tupdatetime\n" +
"\t)\n" +
"\tvalues\n" +
"\t('" + RandomToolkit.generateString(12) + "', \n" +
"\t'" + RandomToolkit.generateString(24) + "', \n" +
"\tnow(), \n" +
"\tnow()\n" +
")";
Connection conn = myBroker.getConnection();
conn.setAutoCommit(false);
Statement stmt = conn.createStatement();
stmt.execute(sql);
conn.commit();
myBroker.freeConnection(conn);
}
Long end = System.currentTimeMillis();
System.out.println("單條執行100000條Insert操作,共耗時:" + (end - start) / 1000f + "秒!");
}
/**
* 批處理執行靜態SQL測試
*
* @param m 批次
* @param n 每批數量
* @throws Exception 異常時丟擲
*/
public static void testInsertBatch(int m, int n) throws Exception {
init(); //初始化環境
Long start = System.currentTimeMillis();
for (int i = 0; i < m; i++) {
//從池中獲取連線
Connection conn = myBroker.getConnection();
conn.setAutoCommit(false);
Statement stmt = conn.createStatement();
for (int k = 0; k < n; k++) {
String sql = "\n" +
"insert into testdb.tuser \n" +
"\t(name, \n" +
"\tremark, \n" +
"\tcreatetime, \n" +
"\tupdatetime\n" +
"\t)\n" +
"\tvalues\n" +
"\t('" + RandomToolkit.generateString(12) + "', \n" +
"\t'" + RandomToolkit.generateString(24) + "', \n" +
"\tnow(), \n" +
"\tnow()\n" +
")";
//加入批處理
stmt.addBatch(sql);
}
stmt.executeBatch(); //執行批處理
conn.commit();
// stmt.clearBatch(); //清理批處理
stmt.close();
myBroker.freeConnection(conn); //連線歸池
}
Long end = System.currentTimeMillis();
System.out.println("批量執行" + m + "*" + n + "=" + m * n + "條Insert操作,共耗時:" + (end - start) / 1000f + "秒!");
}
/**
* 100000條預定義SQL插入
*
* @throws Exception 異常時丟擲
*/
public static void testInsert2() throws Exception { //單條執行100000條Insert操作,共耗時:40.422秒!
init(); //初始化環境
Long start = System.currentTimeMillis();
String sql = "" +
"insert into testdb.tuser\n" +
" (name, remark, createtime, updatetime)\n" +
"values\n" +
" (?, ?, ?, ?)";
for (int i = 0; i < 100000; i++) {
Connection conn = myBroker.getConnection();
conn.setAutoCommit(false);
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, RandomToolkit.generateString(12));
pstmt.setString(2, RandomToolkit.generateString(24));
pstmt.setDate(3, new Date(System.currentTimeMillis()));
pstmt.setDate(4, new Date(System.currentTimeMillis()));
pstmt.executeUpdate();
conn.commit();
pstmt.close();
myBroker.freeConnection(conn);
}
Long end = System.currentTimeMillis();
System.out.println("單條執行100000條Insert操作,共耗時:" + (end - start) / 1000f + "秒!");
}
/**
* 批處理執行預處理SQL測試
*
* @param m 批次
* @param n 每批數量
* @throws Exception 異常時丟擲
*/
public static void testInsertBatch2(int m, int n) throws Exception {
init(); //初始化環境
Long start = System.currentTimeMillis();
String sql = "" +
"insert into testdb.tuser\n" +
" (name, remark, createtime, updatetime)\n" +
"values\n" +
" (?, ?, ?, ?)";
for (int i = 0; i < m; i++) {
//從池中獲取連線
Connection conn = myBroker.getConnection();
conn.setAutoCommit(false);
PreparedStatement pstmt = conn.prepareStatement(sql);
for (int k = 0; k < n; k++) {
pstmt.setString(1, RandomToolkit.generateString(12));
pstmt.setString(2, RandomToolkit.generateString(24));
pstmt.setDate(3, new Date(System.currentTimeMillis()));
pstmt.setDate(4, new Date(System.currentTimeMillis()));
//加入批處理
pstmt.addBatch();
}
pstmt.executeBatch(); //執行批處理
conn.commit();
// pstmt.clearBatch(); //清理批處理
pstmt.close();
myBroker.freeConnection(conn); //連線歸池
}
Long end = System.currentTimeMillis();
System.out.println("批量執行" + m + "*" + n + "=" + m * n + "條Insert操作,共耗時:" + (end - start) / 1000f + "秒!");
}
public static void main(String[] args) throws Exception {
init();
Long start = System.currentTimeMillis();
System.out.println("--------C組測試----------");
testInsert();
testInsertBatch(100, 1000);
testInsertBatch(250, 400);
testInsertBatch(400, 250);
testInsertBatch(500, 200);
testInsertBatch(1000, 100);
testInsertBatch(2000, 50);
testInsertBatch(2500, 40);
testInsertBatch(5000, 20);
Long end1 = System.currentTimeMillis();
System.out.println("C組測試過程結束,全部測試耗時:" + (end1 - start) / 1000f + "秒!");
System.out.println("--------D組測試----------");
testInsert2();
testInsertBatch2(100, 1000);
testInsertBatch2(250, 400);
testInsertBatch2(400, 250);
testInsertBatch2(500, 200);
testInsertBatch2(1000, 100);
testInsertBatch2(2000, 50);
testInsertBatch2(2500, 40);
testInsertBatch2(5000, 20);
Long end2 = System.currentTimeMillis();
System.out.println("D組測試過程結束,全部測試耗時:" + (end2 - end1) / 1000f + "秒!");
}
}
執行結果:
--------C組測試----------
單條執行100000條Insert操作,共耗時:103.656秒!
批量執行100*1000=100000條Insert操作,共耗時:31.328秒!
批量執行250*400=100000條Insert操作,共耗時:31.406秒!
批量執行400*250=100000條Insert操作,共耗時:31.75秒!
批量執行500*200=100000條Insert操作,共耗時:31.438秒!
批量執行1000*100=100000條Insert操作,共耗時:31.968秒!
批量執行2000*50=100000條Insert操作,共耗時:32.938秒!
批量執行2500*40=100000條Insert操作,共耗時:33.141秒!
批量執行5000*20=100000條Insert操作,共耗時:35.265秒!
C組測試過程結束,全部測試耗時:363.656秒!
--------D組測試----------
單條執行100000條Insert操作,共耗時:107.61秒!
批量執行100*1000=100000條Insert操作,共耗時:32.64秒!
批量執行250*400=100000條Insert操作,共耗時:32.641秒!
批量執行400*250=100000條Insert操作,共耗時:33.109秒!
批量執行500*200=100000條Insert操作,共耗時:32.859秒!
批量執行1000*100=100000條Insert操作,共耗時:33.547秒!
批量執行2000*50=100000條Insert操作,共耗時:34.312秒!
批量執行2500*40=100000條Insert操作,共耗時:34.672秒!
批量執行5000*20=100000條Insert操作,共耗時:36.672秒!
D組測試過程結束,全部測試耗時:378.922秒!
單條執行100000條Insert操作,共耗時:103.656秒!
批量執行100*1000=100000條Insert操作,共耗時:31.328秒!
批量執行250*400=100000條Insert操作,共耗時:31.406秒!
批量執行400*250=100000條Insert操作,共耗時:31.75秒!
批量執行500*200=100000條Insert操作,共耗時:31.438秒!
批量執行1000*100=100000條Insert操作,共耗時:31.968秒!
批量執行2000*50=100000條Insert操作,共耗時:32.938秒!
批量執行2500*40=100000條Insert操作,共耗時:33.141秒!
批量執行5000*20=100000條Insert操作,共耗時:35.265秒!
C組測試過程結束,全部測試耗時:363.656秒!
--------D組測試----------
單條執行100000條Insert操作,共耗時:107.61秒!
批量執行100*1000=100000條Insert操作,共耗時:32.64秒!
批量執行250*400=100000條Insert操作,共耗時:32.641秒!
批量執行400*250=100000條Insert操作,共耗時:33.109秒!
批量執行500*200=100000條Insert操作,共耗時:32.859秒!
批量執行1000*100=100000條Insert操作,共耗時:33.547秒!
批量執行2000*50=100000條Insert操作,共耗時:34.312秒!
批量執行2500*40=100000條Insert操作,共耗時:34.672秒!
批量執行5000*20=100000條Insert操作,共耗時:36.672秒!
D組測試過程結束,全部測試耗時:378.922秒!
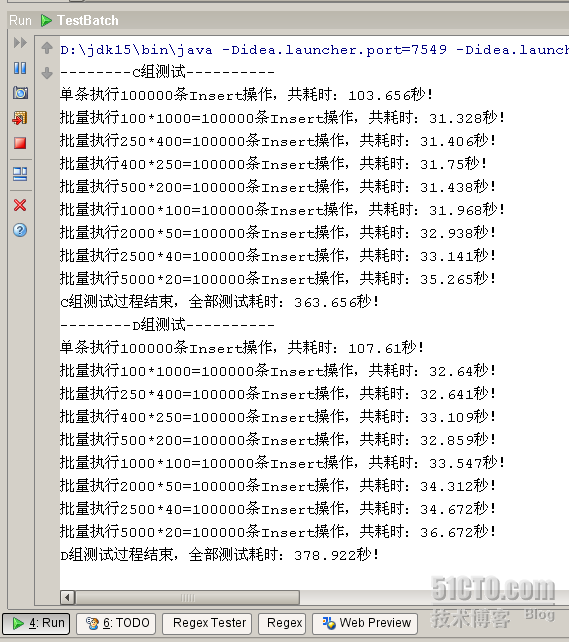
測試結果意想不到吧,最短時間竟然超過上篇。觀察整個測試結果,發現總時間很長,原因是逐條執行的效率太低了。