這是一個分割股票資料的
package com.longxingyu.Stock;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import java.io.BufferedReader;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.TimerTask;
/**
*
* @author Team37
* @return stock data
*/
public class StockData implements StockInterface {
public String s;
private int isNull=0;
ObservableList<TableSet> tableData= FXCollections.observableArrayList();
public int getIsNull(){
return isNull;
}
public void setIsNull(){
this.isNull = 1;
}
public void returnNull(){
this.isNull = 0;
}
public StockData(String s){
this.s=s;
prePare(s);
}
public StockData(){}
public void prePare(String s){//輸入的字串從這裡進來,判斷有多少組資料,再用迴圈去呼叫httpData
String[] sourceArray = s.split(",");//統計要測幾組資料
for(int i=0; i < sourceArray.length; i++){
String temp=httpData(sourceArray[i]);//http請求返回的資料
String array[]=convert(temp);
if(array.length == 1){
setIsNull();
}else{
addData(array);
}
}
}
public void addData(String[] s) {//新增tableColumn元素的值
Calendar calendar=Calendar.getInstance();
Date time = calendar.getTime();
String recTime=(new SimpleDateFormat("HH:mm:ss")).format(time);
String recDate=(new SimpleDateFormat("yyyy-MM-dd")).format(time);
tableData.add(new TableSet(s[0],s[1],s[2],s[3],s[4],s[5],s[8],s[9],recDate,recTime));
}
//返回加入的observablelist
public ObservableList<TableSet> getTableData(){
return this.tableData;
}
/**
* @param urlAl
* :請求介面
* @param httpArg
* :引數
* @return 返回結果
* @author Team37
*/
public String httpData(String httpArg){
String httpUrl = "http://hq.sinajs.cn/list=";
BufferedReader reader = null;
String result = null;
StringBuffer sbf = new StringBuffer();
httpUrl = httpUrl + httpArg;
try {
URL url = new URL(httpUrl);
HttpURLConnection connection = (HttpURLConnection) url
.openConnection();
connection.setRequestMethod("GET");
connection.connect();
InputStream is = connection.getInputStream();
reader = new BufferedReader(new InputStreamReader(is, "GBK"));
String strRead = null;
while ((strRead = reader.readLine()) != null) {
sbf.append(strRead);
sbf.append("\r\n");
}
reader.close();
result = sbf.toString();
} catch (Exception e) {
e.printStackTrace();
}
return result;
}
public String[] convert(String s){//將字串解析為一個陣列對應到引數當中
String[] array;
int begin=s.indexOf("\"");
String temp=s.substring(begin+1,s.length());
array = temp.split(",");
return array;
}
}
這是一個介面
package com.longxingyu.Stock;
/**
* @author Team37
* define the Interfaces
*/
public interface StockInterface {
String httpData(String s);
void addData(String[] s);
}
將資料新增進入TableVew中
package com.longxingyu.Stock;
import javafx.beans.property.SimpleStringProperty;
import javafx.beans.property.StringProperty;
/**
* @author Team37
* define the structure of stock data
*/
public class TableSet {
private StringProperty name;//股票名稱
private StringProperty open;//今日開盤價
private StringProperty lastClose;//昨日收盤價
private StringProperty price;//當前價格
private StringProperty todayHigh;//今日最高價
private StringProperty todayLow;//今日最低價
private StringProperty count;//成交股數
private StringProperty total;//成交金額
private StringProperty date;//日期
private StringProperty time;//時間
public TableSet(String name, String open, String lastClose, String price,
String todayHigh, String todayLow, String count, String total,
String date, String time){
this.name = new SimpleStringProperty(name);
this.open = new SimpleStringProperty(open);
this.lastClose = new SimpleStringProperty(lastClose);
this.price = new SimpleStringProperty(price);
this.todayHigh = new SimpleStringProperty(todayHigh);
this.todayLow = new SimpleStringProperty(todayLow);
this.count = new SimpleStringProperty(count);
this.total = new SimpleStringProperty(total);
this.date = new SimpleStringProperty(date);
this.time = new SimpleStringProperty(time);
}
public String getName(){
return name.get();
}
public void setName(String name){
this.name.set(name);
}
public StringProperty nameProperty(){
return name;
}
public String getOpen(){
return open.get();
}
public void setOpen(String open){
this.open.set(open);
}
public StringProperty openProperty(){
return open;
}
public String getLastClose(){
return lastClose.get();
}
public void setLastClose(String lastClose){
this.lastClose.set(lastClose);
}
public StringProperty lastCloseProperty(){
return lastClose;
}
public String getPrice(){
return lastClose.get();
}
public void setPrice(String price){
this.price.set(price);
}
public StringProperty priceProperty(){
return price;
}
public String getTodayHigh(){
return todayHigh.get();
}
public void setTodayHigh(String todayHigh){
this.todayHigh.set(todayHigh);
}
public StringProperty todayHighProperty(){
return todayHigh;
}
public String getTodayLow(){
return todayLow.get();
}
public void setTodayLow(String todayLow){
this.todayLow.set(todayLow);
}
public StringProperty todayLowProperty(){
return todayLow;
}
public String getCount(){
return count.get();
}
public void setCount(String count){
this.count.set(count);
}
public StringProperty countProperty(){
return count;
}
public String getTotal(){
return total.get();
}
public void setTotal(String total){
this.total.set(total);
}
public StringProperty totalProperty(){
return total;
}
public String getDate(){
return date.get();
}
public void setDate(String date){
this.date.set(date);
}
public StringProperty dateProperty(){
return date;
}
public String getTime(){
return time.get();
}
public void setTime(String time){
this.date.set(time);
}
public StringProperty timeProperty(){
return time;
}
}
一個控制器
package com.longxingyu.Controller;
import com.longxingyu.Stock.TableSet;
import com.longxingyu.Stock.StockData;
import javafx.event.Event;
import javafx.fxml.FXML;
import javafx.scene.control.Label;
import javafx.scene.control.TableColumn;
import javafx.scene.control.TableView;
import javafx.scene.control.TextField;
import javafx.scene.layout.Pane;
import javax.swing.*;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.TimerTask;
/**
* Created by Team37
* control some item
*/
public class Controller {
public Controller(){
}
public Pane pane;
public TextField text;
@FXML
private TableView<TableSet> table;
@FXML
private TableColumn<TableSet,String> nameCol;
@FXML
private TableColumn<TableSet,String> openCol;
@FXML
private TableColumn<TableSet,String> lastCloseCol;
@FXML
private TableColumn<TableSet,String> priceCol;
@FXML
private TableColumn<TableSet,String> todayHighCol;
@FXML
private TableColumn<TableSet,String> todayLowCol;
@FXML
private TableColumn<TableSet,String> countCol;
@FXML
private TableColumn<TableSet,String> totalCol;
@FXML
private TableColumn<TableSet,String> dateCol;
@FXML
private TableColumn<TableSet,String> timeCol;
@FXML
private void init(){//初始化
nameCol.setCellValueFactory(cellData -> cellData.getValue().nameProperty());
openCol.setCellValueFactory(cellData -> cellData.getValue().openProperty());
lastCloseCol.setCellValueFactory(cellData -> cellData.getValue().lastCloseProperty());
priceCol.setCellValueFactory(cellData -> cellData.getValue().priceProperty());
todayHighCol.setCellValueFactory(cellData -> cellData.getValue().todayHighProperty());
todayLowCol.setCellValueFactory(cellData -> cellData.getValue().todayLowProperty());
countCol.setCellValueFactory(cellData -> cellData.getValue().countProperty());
totalCol.setCellValueFactory(cellData -> cellData.getValue().totalProperty());
dateCol.setCellValueFactory(cellData -> cellData.getValue().dateProperty());
timeCol.setCellValueFactory(cellData -> cellData.getValue().timeProperty());
}
public void addList(StockData back){//將資料加入列表
table.setItems(back.getTableData());
}
public void btnAction(Event event){
java.util.Timer timer=new java.util.Timer();
if(text.getText().length()!=0){
String value = text.getText();//表示獲取的值
timer.scheduleAtFixedRate(new TimerTask() {
@Override
public void run() {
StockData back = new StockData(text.getText());
if(value.length()!=0){
// Calendar calendar = Calendar.getInstance();
// Date times = calendar.getTime();
// System.out.println((new SimpleDateFormat("yyyy-MM-dd HH:mm:ss")).format(times));
//System.out.println("out"+back.getIsNull());
if(back.getIsNull() == 1){//判斷返回值如果為1就是股票資料沒有的時候
JOptionPane.showMessageDialog(null, "輸入的股票程式碼有錯誤","錯誤提示",1);
back.returnNull();//讓isNull的值回歸正常的值0 判斷是不是空的字串
//System.out.println("in"+back.getIsNull());
text.setText("");
timer.cancel();//結束這個執行緒
}
else{
init();
addList(back);//利用當前物件
}
//System.out.println(back.getTableData().size());
}
}
},0,20000);//調整重新整理時間
}
else{
JOptionPane.showMessageDialog(null, "輸入的股票程式碼有錯誤","錯誤提示",1);
}
}
}
用fxml寫的一個介面
<?xml version="1.0" encoding="UTF-8"?>
<!-- @author Team37
define the UI
-->
<?import javafx.scene.control.Button?>
<?import javafx.scene.control.Label?>
<?import javafx.scene.control.ScrollPane?>
<?import javafx.scene.control.TableColumn?>
<?import javafx.scene.control.TableView?>
<?import javafx.scene.control.TextField?>
<?import javafx.scene.layout.Pane?>
<Pane fx:id="pane" maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="480.0" prefWidth="774.0" xmlns="http://javafx.com/javafx/8.0.60" xmlns:fx="http://javafx.com/fxml/1" fx:controller="com.longxingyu.Controller.Controller">
<children>
<Button layoutX="480.0" layoutY="28.0" mnemonicParsing="false" onAction="#btnAction" prefHeight="26.0" prefWidth="93.0" text="確定" />
<Label layoutX="25.0" layoutY="20.0" prefHeight="34.0" prefWidth="177.0" text="請輸入你想要查詢的股票程式碼" />
<TextField fx:id="text" layoutX="212.0" layoutY="28.0" prefHeight="26.0" prefWidth="227.0" />
<ScrollPane layoutX="-402.0" layoutY="96.0" prefHeight="276.0" prefWidth="341.0" />
<ScrollPane layoutY="89.0" prefHeight="367.0" prefWidth="775.0">
<content>
<TableView fx:id="table" prefHeight="390.0" prefWidth="900.0">
<columns>
<TableColumn fx:id="nameCol" prefWidth="75.0" text="股票名稱" />
<TableColumn fx:id="openCol" prefWidth="75.0" text="今日開盤價" />
<TableColumn fx:id="lastCloseCol" prefWidth="83.0" text="昨日收盤價" />
<TableColumn fx:id="priceCol" prefWidth="67.0" text="當前價格" />
<TableColumn fx:id="todayHighCol" prefWidth="75.0" text="今日最高價" />
<TableColumn fx:id="todayLowCol" prefWidth="75.0" text="今日最低價" />
<TableColumn fx:id="countCol" prefWidth="75.0" text="成交股數" />
<TableColumn fx:id="totalCol" prefWidth="86.0" text="成交金額" />
<TableColumn fx:id="dateCol" prefWidth="75.0" text="當前日期" />
<TableColumn fx:id="timeCol" prefWidth="69.0" text="當前時間" />
</columns>
</TableView>
</content>
</ScrollPane>
</children>
</Pane>
接著主函式啟動
package com.longxingyu;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
/**
* @author Team37
* Main啟動
*/
public class Main extends Application {
@Override
public void start(Stage primaryStage) throws Exception{
Parent root = FXMLLoader.load(getClass().getResource("View/Main.fxml"));
primaryStage.setTitle("實時股票查詢系統");
primaryStage.setScene(new Scene(root));
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
執行結果如圖
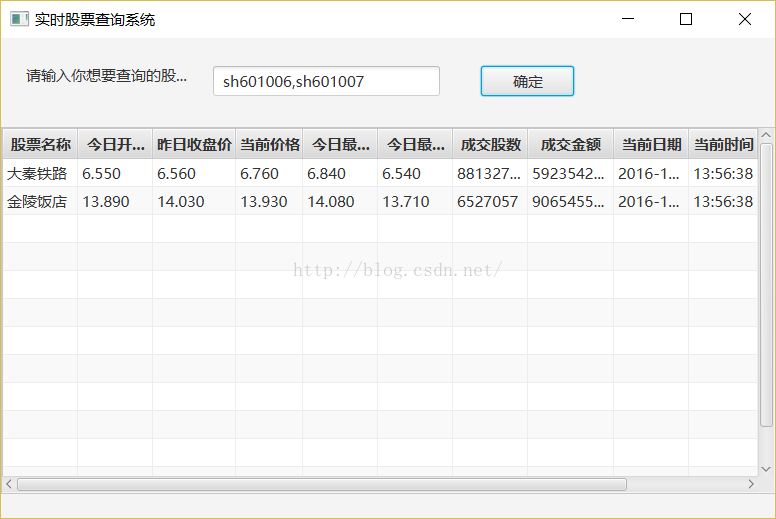