傅立葉變換
對於週期訊號,如果滿足 \(Dirichlet\) 條件,就可以嘗試將其分解為傅立葉級數,並繪製成頻譜的形式,但是在實際使用的過程中我們遇到的訊號往往既不是週期的訊號又難以獲取解析式。對於複雜的現實訊號,我們可以將問題的難點拆分開,我們先解決不是週期訊號但解析式已知的情況,再去解決難以獲取解析式的情況。
從週期訊號到非週期訊號
首先我們先看一個問題:對於週期矩形脈衝訊號,週期不斷變大過程中,頻譜如何發生變化?
經過實驗我們得到下面的一組圖:
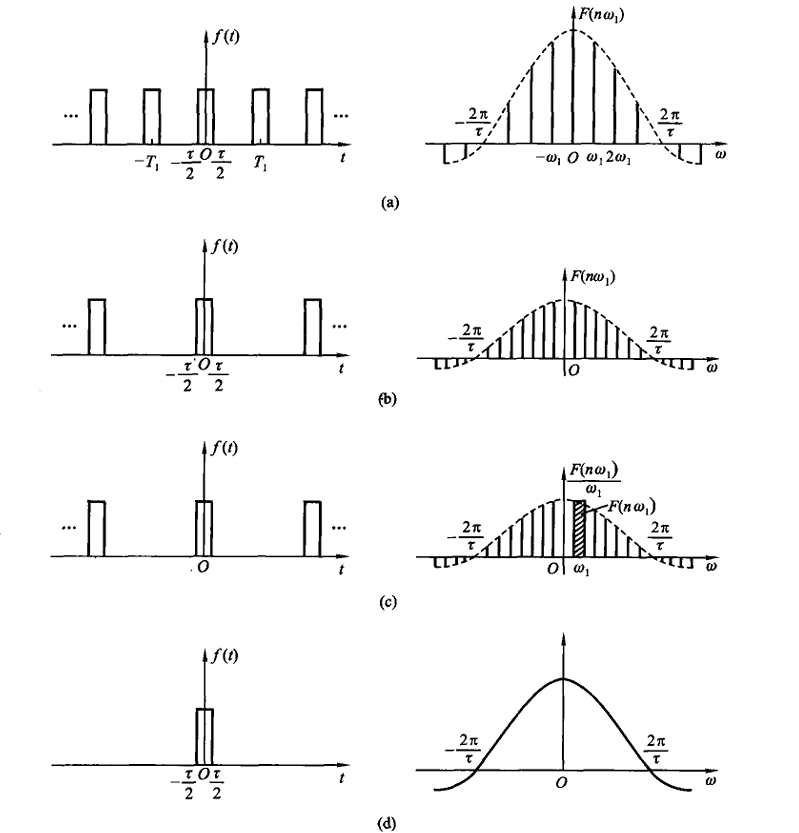
我們可以觀察到,隨著週期的不斷變大,頻譜的譜線之間距離變得越來越近。
上面的分析結果雖然是針對於週期矩形脈衝訊號而言的,但是其具有一定的普適性,對於其他週期訊號而言也能得到類似的結論。由此得到一個簡單的想法————非週期訊號可以視作訊號的週期為\(\infty\)的週期訊號。另外,我們知道訊號的能量是有限值,對於週期訊號,由帕斯瓦爾定理,我們知道幅度譜線的縱座標的數值是與能量相關的引數,在改變訊號週期的時候我們並未對其中的能量進行改變,也就是總的面積不變。基於這樣的考慮,我們引入一個函式 "頻譜密度函式",函式中的每一個值都是在一個頻率點附近能量和頻率的比值,類似於機率論中機率密度函式中離散和連續的情況。
接下來就按照上述思想進行公式推導,看能否得到什麼?
指數形式的傅立葉級數:
\[f(t) = \sum_{n = -\infty}^{\infty} F(n \omega )e^{j n \omega t} \\
\]
頻譜為:
\[F(n \omega) = \frac{1}{T} \int_{-\frac{T}{2}}^{\frac{T}{2}} f(t) e^{{-j n \omega t}} \text{{d}t }\\
\]
兩邊同時乘上週期或者說是除以頻率:
\[F(n \omega) T= \frac{2\pi}{\omega} F(n \omega) = \int_{-\frac{T}{2}}^{\frac{T}{2}} f(t) e^{{-j n \omega t}} \text{{d}t }\\
\]
當週期 \(T\to \infty\) ,有 \(\omega \to 0\) ,同時還有 \(n \to 1\) 。定義函式:
\[F(\omega) = \lim_{ \omega \to 0 } \frac{2\pi}{\omega} F(n \omega) = \lim_{ T \to \infty } F(n \omega) T
\]
式子中 \(\displaystyle{\frac{2\pi F(n \omega)}{\omega}}\) 就是頻譜密度,稱 \(F(\omega)\) 為頻譜密度函式,簡稱頻譜函式。
由此我們便能夠得到下面這樣一組式子:
\[F(\omega) = \int_{-\infty}^{\infty} f(t) e^{-j \omega t}\text{d}x
\]
\[f(t) = \frac{1}{2\pi} \int _{-\infty}^{\infty} F(\omega) e^{j \omega t} \, \text{d}x
\]
上面的兩個式子便是傅立葉變換公式和傅立葉反變換公式,並且稱上面的\(f(t)\)和\(F(\omega)\)為一個傅立葉變換對,簡記為\(f(t)\leftrightarrow F(\omega)\).
其中的 \(F(\omega)\) 是個複數,將他的模值 \(|F(\omega) |\) 作為幅度譜,相位作為相位譜。於是就得到了非週期訊號的兩譜圖。
與週期訊號離散的譜圖類似,非週期訊號的連續譜圖中,幅度譜為偶函式,相位譜圖為奇函式。
上面的推理過程在數學上並不是嚴格的,理論上傅立葉變化也不是任何函式都能進行變換,需要滿足條件:
\[\int _{-\infty}^{\infty} | f(t)|\, \text{d}x < \infty
\]
但是對於大多數訊號都是滿足上面的關係的,並且傅立葉變換是個很好的工具,對於某些奇異函式也是適用的(如衝激訊號和階躍訊號等)。
典型非週期訊號的傅立葉變換
(一)單邊指數訊號
原訊號:
\[f(t) =
\begin{cases}
e^{-at} \qquad &(t \geqslant 0)\\ \\
0 \qquad &(t < 0)\\
\end{cases}
\]
傅立葉變換後的式子:
\[\begin{cases}
F(\omega) = \displaystyle\frac{1}{a+j \omega}\\ \\
| F(\omega) | = \displaystyle{\frac{1}{\sqrt{a^{2} + \omega^{2}}}}\\ \\
\varphi(\omega) = -\arctan\left( \displaystyle\frac{\omega}{a} \right)\\
\end{cases}
\]
(二)雙邊指數訊號
雙邊指數訊號:
\[f(t) = e^{-a | t |} \qquad (-\infty < t<\infty)
\]
雙邊指數訊號的傅立葉變換:
\[\begin{cases}
F(\omega) = \displaystyle\frac{2a}{a^{2}+\omega^{2}}\\ \\
| F(\omega) | = \displaystyle{\frac{2a}{{a^{2} + \omega^{2}}}}\\ \\
\varphi(\omega) = 0\\
\end{cases}
\]
(三)矩形脈衝訊號
矩形脈衝訊號:
\[f(t) = E\left[u\left( t - \displaystyle{\frac{\tau}{2}} \right) - u\left( t - \displaystyle{\frac{\tau}{2}} \right) \right]
\]
矩形脈衝訊號的傅立葉變換:
\[\begin{cases}
F(\omega) = E\tau \cdot\text{Sa}\left( \displaystyle{\frac{\omega\tau}{2}} \right)\\ \\
|F(\omega)| = E\tau \cdot \left|\text{Sa}\left( \displaystyle{\frac{\omega\tau}{2}} \right)\right|\\ \\
\varphi(\omega) = \begin{cases}0\\
\pi\\ \end{cases}
\end{cases}
\]
(四)鐘形脈衝訊號
鐘形訊號:
\[f(t) = Ee^{- (\frac {t}{\tau})^{2}}
\]
鐘形訊號的傅立葉變換:
\[F(\omega) =\sqrt{ \pi } E\tau \cdot e^{-(\frac{\omega \tau} {2})^{2}}
\]
依舊是鐘形的
(五)符號函式
符號函式:
\[f(t) = \text{sgn}(t)
\]
符號函式本身不滿足絕對可積分,但是可以對他進行傅立葉變換
不妨設想雙邊奇對稱指數訊號,如果 \(a\to 0\) 的話就非常的接近符號函式,對他進行傅立葉變換,就可以得到
符號函式的傅立葉變換:
\[\begin{cases}
F(\omega) & = \displaystyle \frac{2}{j \omega}\\ \\
| F(\omega) | & = \displaystyle{ \frac{2}{| \omega |}}\\ \\
\varphi (\omega) & = \begin{cases}
-\displaystyle \frac{\pi}{2}\quad & & (\omega > 0 )\\ \\
\displaystyle \frac{\pi}{2} \quad & & (\omega <0)\\
\end{cases}
\end{cases}
\]
(六)升餘弦脈衝訊號
升餘弦脈衝訊號:
\[f(t) = \frac{E}{2} \left[ 1 + \cos \left( \frac{\pi t}{\tau} \right) \right] \quad (0 \leqslant |t| \leqslant \tau)
\]
就是把餘弦訊號向上平移半個峰峰值,第一個凸起的訊號
升餘弦訊號的傅立葉變換
\[\begin{aligned}
F(\omega) & = \frac {E\sin(\omega \tau)}{\omega \left[ 1 - \left(\frac{\omega \tau}{\pi}^{2}\right) \right]}\\ \\
& = \frac{E\tau\text{Sa}(\omega t)}{1 - \left(\frac{\omega \tau }{\pi}\right)^{2}}
\end{aligned}
\]
前面我們計算了一些常見的傅立葉變換,但是這樣的計算更多是為了便於讀者熟悉傅立葉變換這樣的工具。當讀者熟悉傅立葉變換的相關性質,上面的部分訊號有更加簡便的求解方式。下面我們繼續學習一些奇異訊號的傅立葉變換。
奇異訊號的傅立葉變換
(一)衝激函式的傅立葉變換
衝激函式的傅立葉變換為:
\[\mathscr{F} \Big[\delta(t)\Big] = 1
\]
單位衝激函式的頻譜為常數,或者說是“白色譜”。
衝激函式的傅立葉逆變換(從衝激函式的傅立葉變化可以推出):
\[\mathscr{F}^{-1} \Big[ \delta(\omega)\Big]= \frac{1}{2\pi}
\]
切記特殊訊號的傅立葉逆變換直接進行積分運算可能較為複雜,如果不熟悉複變函式的積分會得到錯誤結論。 比如下面的。
\[\mathscr{F}(1) = 2\pi \delta(\omega)
\]
直流訊號的傅立葉變換是零點處的衝激訊號。
(二)衝擊偶函式的傅立葉變換
衝擊偶函式的傅立葉變換可以透過下面方法得到:
\[\begin{aligned}
& \delta(t) = \frac{1}{2\pi} \int _{-\infty}^{\infty} e^{j \omega t} \, \text{d}\omega \\ \\
& \quad (\text{對兩邊求導})\\ \\
& \frac{\text{d}}{\text{d}t} \delta(t) = \frac{1}{2\pi} \int _{-\infty}^{\infty} j \omega e^{j \omega t} \, \text{d}\omega
\end{aligned}
\]
由此可以知道衝激函式的傅立葉變換為:
\[\mathscr{F} \Big[ \frac{\text{d}}{\text{d}t} \delta(t) \Big] = j \omega
\]
如果直接採用傅立葉變換的定義和衝擊偶函式的性質也可以得到上面的結論。
同理可以知道:
\[\mathscr{F} \Big[ \frac{\text{d}^{n}}{\text{d}t^{n}} \delta(t) \Big] = (j \omega )^{n}
\]
\[\mathscr{F} \Big[ t^{n} \Big] = 2\pi (j)^{n} \frac{\text{d}^{n}}{\text{d}\omega^{n}} \delta(\omega )
\]
(三)階躍函式的傅立葉變換
\[\mathscr{F}\Big[u(t)\Big]= \pi \delta(\omega) + \frac{1}{j\omega}
\]
傅立葉變換的性質
傅立葉變換的性質能夠極大的簡化某些複雜訊號的計算過程,因此勢必要仔細理解每一條性質並且活學活用。
(一)對稱性
若 \(F(\omega) = \mathscr{F}\Big[ f(t)\Big]\) ,則 \(\mathscr{F}\Big[ F(t)\Big] = 2\pi f(-\omega)\) .
如果知道 \(f(t)\) ,我們需要求他的傅立葉變換後圖形的傅立葉變換,則可以透過求解 \(2\pi f(-\omega)\) 得到。
(二)疊加性
先疊加再傅立葉變換等於先傅立葉變換再疊加。也就是傅立葉變換滿足線性變換的性質。
(三)奇偶虛實性
1、\(f(t)\) 是實函式
實函式的傅立葉變換的實部為偶函式,虛部為奇函式,幅度譜為偶函式,相位譜為奇函式。
\[\begin{cases}
R(\omega) = R(-\omega)\\ \\
X(\omega) = -X(-\omega)\\ \\
F(-\omega) = F^{*}(\omega)\\
\end{cases}
\]
推論:實偶函式的傅立葉變換是實偶函式,實奇函式的傅立葉變換是虛奇函式。
2、\(f(t)\) 為虛擬函式
虛擬函式的傅立葉變換的實部為奇函式,虛部為偶函式。
\[\begin{cases}
R(\omega) = R(-\omega)\\ \\
X(\omega) = -X(-\omega)\\ \\
F(-\omega) = F^{*}(\omega)\\
\end{cases}
\]
3、\(f(t)\) 為實函式或者複函式
\[\begin{cases}
\mathscr{F}\Big[ f(-t)\Big] = F(-\omega)\\ \\
\mathscr{F}\Big[ f^{*}(t)\Big] = F^{*}(-\omega)\\ \\
\mathscr{F}\Big[ f^{*}(-t)\Big] = F^{*}(\omega)\\
\end{cases}
\]
(四)尺度變換特性
若 \(\mathscr{F}\Big[ f(t)\Big] = F(\omega)\) , 則
\[\mathscr{F}\Big[ f(at)\Big] = \frac{1}{|a|}F\left( \frac{\omega}{a} \right)
\]
時域上訊號的壓縮,等於頻域上訊號的擴充套件;
時域上訊號的擴充套件,等於頻域上訊號的壓縮。
關於 \(\displaystyle \frac{1}{|a|}\) 的物理含義:
時域上的壓縮,訊號能量減少,因此需要乘上 \(\displaystyle \frac{1}{|a|}, \quad(a>1)\) ;
時域上的擴充套件,訊號能量變多,因此需要乘上 \(\displaystyle \frac{1}{|a|}, \quad(a<1)\) .
訊號的等效脈寬與佔有的等效頻寬成反比例,要想獲得更快的傳輸密度,需要壓縮脈寬,同時需要佔用更大的頻帶,是對頻帶資源的浪費。
(五)時移特性
若 \(\mathscr{F}\Big[ f(t)\Big] = F(\omega)\) , 則
\[\mathscr{F}\Big[ f(t - t_{0})\Big] = e^{-j \omega t_{0}}F\left( \omega \right)
\]
時間上的移動會導致頻帶上幅度譜的譜值不變,並且帶來相位上 \(-\omega t_{0}\). 從這裡可以知道時間上的滯後不會影響訊號的能量.
(六)頻移特性
若 \(\mathscr{F}\Big[ f(t)\Big] = F(\omega)\) , 則
\[\mathscr{F}\left[ f(t) e^{j\omega_{0} t}\right] = F\left( \omega - \omega_{0}\right)
\]
頻移技術:將原訊號乘上一個正弦、餘弦或者正餘弦混合訊號,等效於乘上 \(e^{j \omega_{0} t}\) 隨後就將訊號的頻譜進行了移動,使其移動到合適的未被佔用的頻帶。這項技術廣泛應用於通訊中。
(七)微分特性
若 \(\mathscr{F}\Big[ f(t)\Big] = F(\omega)\) , 則
\[\mathscr{F}\left[ \frac{\text{d}^{n}f(t)}{\text{d}t^{n}} \right] = (j\omega)^{n} F\left( \omega \right)
\]
若 \(\mathscr{F}\Big[ F(t)\Big] = F(\omega)\) , 則
\[\mathscr{F}^{-1} \left[ \frac{\text{d}^{n}F(\omega)}{\text{d}\omega^{n}} \right] = (-jt)^{n} f(t)
\]
(八)積分特性
若 \(\mathscr{F}\Big[ f(t)\Big] = F(\omega)\) , 則
\[\mathscr{F}\left[ \int_{-\infty}^{t} f(\tau) \text{d} \tau \right ] = \frac{F\left( \omega \right) }{j \omega} + \pi F(0) \delta(\omega)
\]
若 \(\mathscr{F}\Big[ F(t)\Big] = F(\omega)\) , 則
\[\mathscr{F}^{-1} \left[ \int_{-\infty}^{\omega} F(\Omega) \text{d} \Omega \right] = - \frac{f(t)}{jt} + \pi f(0) \delta(t)
\]
(九)卷積性質
1、時域卷積定理
給定兩個時間函式 \(f_1(t)\) 和 \(f_2(t)\)
已知:
\[\begin{aligned}
\mathscr{F}[f_{1}(t)] & = F_{1}(\omega)\\
\mathscr{F}[f_{2}(t)] & = F_{2}(\omega)
\end{aligned}
\]
則:
\[\mathscr{F}[f_{1}(t) * f_{2}(t)] = F_{1}(\omega) F_{2}(\omega)
\]
兩函式的卷積的傅立葉變換等於兩函式傅立葉變換後的乘積。
2、頻域卷積定理
給定兩個時間函式 \(f_1(t)\) 和 \(f_2(t)\)
已知:
\[\begin{aligned}
\mathscr{F}[f_{1}(t)] & = F_{1}(\omega)\\
\mathscr{F}[f_{2}(t)] & = F_{2}(\omega)
\end{aligned}
\]
則:
\[\mathscr{F}[f_{1}(t) \cdot f_{2}(t)] = \frac{1}{2\pi}F_{1}(\omega) * F_{2}(\omega)
\]
兩函式的傅立葉變換在頻域上的卷積再乘上 \(\displaystyle{\frac{1}{2\pi}}\) 後的結果與兩函式相乘後在進行傅立葉變換相同。
頻域卷積定理主要用在通訊系統的調製與解調。
結語
傅立葉變換是訊號分析和通訊的基礎,後續的其他型別的傅立葉變換也是基於最原始的傅立葉變化得到的,無論是離散時間傅立葉變換還是離散傅立葉變換,都需要對原始的傅立葉變換非常熟悉。淺顯描述傅立葉變換和傅立葉級數,傅立葉級數相當於把訊號一巴掌拍散,出現無限個有一定間隔的幻影,而傅立葉變換將訊號拍成緻密幻影。
文章的最後附上一張傅立葉變換表,方便讀者查閱。
性質 |
時域\(f(t)\) |
頻域\(F(\omega)\) |
時域頻域對應關係 |
1. 線性 |
\(\sum_{i=1}^{n}{a_{i}f_{i}(t)}\) |
\(\sum_{i=1}^{n}{a_{i}F_{i}(\omega)}\) |
線性疊加 |
2. 對稱性 |
\(F(t)\) |
\(2\pi f(-\omega)\) |
對稱 |
3. 尺度變換 |
\(f(at)\) |
\(\frac{1}{| a |}F\left( \frac{\omega}{a} \right)\) |
壓縮與擴充套件 |
4. 時移 |
\(f(t-t_{0})\) |
\(F(\omega)e^{-j\omega t_{0}}\) |
時移與相移 |
5. 頻移 |
\(f(t)e^{j\omega_{0}t}\) |
\(F(\omega-\omega_{0})\) |
調製與頻移 |
6. 時域微分 |
\(\frac{\text{d}^{n}f(t)}{\text{d}t^{n}}\) |
\((j\omega)^{n} F( \omega)\) |
|
7. 頻域微分 |
\((-jt)^{n} f(t)\) |
\(\frac{\text{d}^{n}F(\omega)}{\text{d}\omega^{n}}\) |
|
8. 時域積分 |
\(\int_{-\infty}^{t} f(\tau) \text{d} \tau\) |
\(\frac{F\left( \omega \right) }{j \omega} + \pi F(0) \delta(\omega)\) |
|
9. 時域卷積 |
\(f_{1}(t) * f_{2}(t)\) |
\(F_{1}(\omega) F_{2}(\omega)\) |
|
10. 頻域卷積 |
\(f_{1}(t) \cdot f_{2}(t)\) |
\(\frac{1}{2\pi}F_{1}(\omega) * F_{2}(\omega)\) |
|