POJ 3565 Ants (最小權完美匹配 KM演算法)
Time Limit: 5000MS | Memory Limit: 65536K | |||
Total Submissions: 5583 | Accepted: 1730 | Special Judge |
Description
Young naturalist Bill studies ants in school. His ants feed on plant-louses that live on apple trees. Each ant colony needs its own apple tree to feed itself.
Bill has a map with coordinates of n ant colonies and n apple trees. He knows that ants travel from their colony to their feeding places and back using chemically tagged routes. The routes cannot intersect each other or ants will get confused and get to the wrong colony or tree, thus spurring a war between colonies.
Bill would like to connect each ant colony to a single apple tree so that all n routes are non-intersecting straight lines. In this problem such connection is always possible. Your task is to write a program that finds such connection.
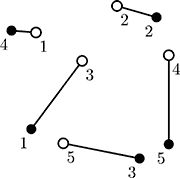
On this picture ant colonies are denoted by empty circles and apple trees are denoted by filled circles. One possible connection is denoted by lines.
Input
The first line of the input file contains a single integer number n (1 ≤ n ≤ 100) — the number of ant colonies and apple trees. It is followed by n lines describing n ant colonies, followed by n lines describing n apple trees. Each ant colony and apple tree is described by a pair of integer coordinates x and y (−10 000 ≤ x, y ≤ 10 000) on a Cartesian plane. All ant colonies and apple trees occupy distinct points on a plane. No three points are on the same line.
Output
Write to the output file n lines with one integer number on each line. The number written on i-th line denotes the number (from 1 to n) of the apple tree that is connected to the i-th ant colony.
Sample Input
5
-42 58
44 86
7 28
99 34
-13 -59
-47 -44
86 74
68 -75
-68 60
99 -60
Sample Output
4
2
1
5
3
Source
題目連結:http://poj.org/problem?id=3565
題目大意:n個白點和n個黑點,用n條不相交的線段把它們連線起來,求一個不相交的完美匹配的方案
題目分析:根據三角形兩邊之和大於第三邊可以證明最小權匹配是不相交的,然後就是模板了
#include <cstdio>
#include <cstring>
#include <cmath>
#include <algorithm>
using namespace std;
int const MAX = 105;
int const INF = 0x3fffffff;
double const EPS = 1e-6;
int n;
int visx[MAX], visy[MAX], lk[MAX], ans[MAX];
double lx[MAX], ly[MAX], w[MAX][MAX], slack[MAX];
struct POINT
{
double x, y;
}p[MAX * 2];
double dis(POINT a, POINT b)
{
return sqrt((a.x - b.x) * (a.x - b.x) + (a.y - b.y) * (a.y - b.y));
}
int DFS(int x)
{
visx[x] = 1;
for(int y = 1; y <= n; y++)
{
if(visy[y])
continue;
double t = lx[x] + ly[y] - w[x][y];
if(t < EPS)
{
visy[y] = 1;
if(lk[y] == -1 || DFS(lk[y]))
{
lk[y] = x;
return 1;
}
}
else if(slack[y] - t > EPS)
slack[y] = t;
}
return 0;
}
void KM()
{
memset(lk, -1, sizeof(lk));
memset(ly, 0, sizeof(ly));
memset(lx, 0, sizeof(lx));
for(int i = 1; i <= n; i++)
for(int j = 1; j <= n; j++)
if(w[i][j] > lx[i])
lx[i] = w[i][j];
for(int x = 1; x <= n; x ++)
{
for(int i = 1; i <= n; i ++)
slack[i] = INF;
while(true)
{
memset(visx, 0, sizeof(visx));
memset(visy, 0, sizeof(visy));
if(DFS(x))
break;
double d = INF;
for(int i = 1; i <= n; i++)
if(!visy[i] && d > slack[i])
d = slack[i];
for(int i = 1; i <= n; i++)
if(visx[i])
lx[i] -= d;
for(int i = 1; i <= n; i++)
{
if(visy[i])
ly[i] += d;
else
slack[i] -= d;
}
}
}
}
int main ()
{
while(scanf("%d", &n) != EOF)
{
memset(w, 0, sizeof(w));
for(int i = 1; i <= 2 * n; i++)
scanf("%lf %lf", &p[i].x, &p[i].y);
for(int i = 1; i <= n; i++)
for(int j = 1; j <= n; j++)
w[i][j] -= dis(p[i], p[j + n]);
KM();
for(int i = 1; i <= n; i++)
ans[lk[i]] = i;
for(int i = 1; i <= n; i++)
printf("%d\n", ans[i]);
}
}
相關文章
- poj2400 KM演算法二分圖的完美匹配演算法
- POJ 2195-Going Home(KM演算法/最小費用最大流演算法)Go演算法
- Uva11383 二分圖的完美匹配(深入理解KM演算法)演算法
- KM演算法——二分圖的最佳匹配演算法
- HDU 2255-奔小康賺大錢(Kuhn-Munkras演算法/KM演算法-完備匹配下的最大權匹配)演算法
- 二分圖最大權完美匹配
- KM演算法演算法
- hdu2255 二分圖的最佳匹配 KM演算法演算法
- 二分圖的最大匹配、完美匹配和匈牙利演算法演算法
- KM演算法小記演算法
- POJ 3469-Dual Core CPU(Dinic 最大流/最小割演算法)演算法
- POJ 1325-Machine Schedule(二分圖匹配-匈牙利演算法)Mac演算法
- POJ 2914-Minimum Cut(Stoer_Wagner最小割演算法)演算法
- POJ-3461 Oulipo-匹配的字元有幾個(KMP演算法)字元KMP演算法
- 學習筆記----KM演算法筆記演算法
- POJ 1469-COURSES(二分圖匹配入門-匈牙利演算法)演算法
- Python RE庫的貪婪匹配和最小匹配Python
- POJ 3014:Asteroids(二分匹配,匈牙利演算法)AST演算法
- POJ 2051(最小堆/優先佇列)佇列
- 對KM演算法暫時性的理解演算法
- 1.1.3.3 最小割之最小權覆蓋集、最大權獨立集
- POJ 3308 Paratroopers 最小割、最大流OOP
- poj 3164 Command Network(最小樹形圖模板題)朱_ 劉演算法演算法
- POJ 基本演算法演算法
- POJ - 3041 Asteroids 【二分圖匹配】AST
- POJ 2955-Brackets(括號匹配-區間DP)Racket
- poj--3264Balanced Lineup+ST演算法求區間最大最小值演算法
- POJ 1465-Multiple(BFS-最小整倍數)
- POJ 2195 Going Home 最小費用最大流Go
- POJ 2195 Going Home (最小費用最大流)Go
- POJ 3041-Asteroids(二分圖匹配)AST
- POJ-2192 Zipper-順序合成串匹配
- go ants原始碼分析Go原始碼
- restful鑑權白名單匹配urlREST
- POJ 1734 Sightseeing trip Floyd求無向圖最小環
- 模式匹配-KMP演算法模式KMP演算法
- 演算法與資料結構之帶權圖與圖最小生成樹演算法資料結構
- 系統最小的服務最小的許可權最大的安全。