Codeforces Round #319 (Div. 2) (ABCE題解)
比賽連結:http://codeforces.com/contest/577
Let's consider a table consisting of n rows and n columns. The cell located at the intersection of i-th row and j-th column contains number i × j. The rows and columns are numbered starting from 1.
You are given a positive integer x. Your task is to count the number of cells in a table that contain number x.
The single line contains numbers n and x (1 ≤ n ≤ 105, 1 ≤ x ≤ 109) — the size of the table and the number that we are looking for in the table.
Print a single number: the number of times x occurs in the table.
10 5
2
6 12
4
5 13
0
A table for the second sample test is given below. The occurrences of number 12 are marked bold.
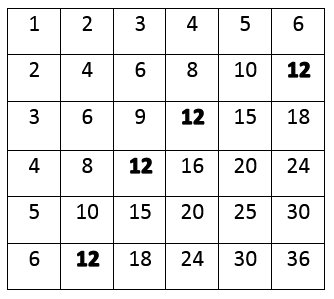
題目分析:列舉約數判斷
#include <cstdio>
int main()
{
int n, x, ans = 0;
scanf("%d %d", &n, &x);
for(int i = 1; i * i <= x; i ++)
{
if(x % i == 0 && x / i <= n && i <= n)
{
if(i * i == x)
ans += 1;
else
ans += 2;
}
}
printf("%d\n", ans);
}
time limit per test:2 seconds
memory limit per test:256 megabytes
You are given a sequence of numbers a1, a2, ..., an, and a number m.
Check if it is possible to choose a non-empty subsequence aij such that the sum of numbers in this subsequence is divisible by m.
The first line contains two numbers, n and m (1 ≤ n ≤ 106, 2 ≤ m ≤ 103) — the size of the original sequence and the number such that sum should be divisible by it.
The second line contains n integers a1, a2, ..., an (0 ≤ ai ≤ 109).
In the single line print either "YES" (without the quotes) if there exists the sought subsequence, or "NO" (without the quotes), if such subsequence doesn't exist.
3 5
1 2 3
YES
1 6
5
NO
4 6
3 1 1 3
YES
6 6
5 5 5 5 5 5
YES
In the first sample test you can choose numbers 2 and 3, the sum of which is divisible by 5.
In the second sample test the single non-empty subsequence of numbers is a single number 5. Number 5 is not divisible by 6, that is, the sought subsequence doesn't exist.
In the third sample test you need to choose two numbers 3 on the ends.
In the fourth sample test you can take the whole subsequence.
題目大意:給n個數字,問存不存在一個子序列的和是m的倍數
題目分析:和POJ 1745差不多,主要是這題的n太大不能看二維,但是當前狀態可以由之前的累計狀態推出,所以開兩個陣列一個記錄累計的餘數,一個記錄當前的餘數,a[i] = true,表示當前狀態出現餘數為i的情況,然後累計給b陣列,一旦b[0] = true,則退出
#include <cstdio>
#include <cstring>
int const MAX = 1e3 + 5;
bool a[MAX], b[MAX], flag;
int main()
{
int n, m;
scanf("%d %d", &n, &m);
flag = false;
for(int i = 0; i < n; i++)
{
int tmp;
scanf("%d", &tmp);
memset(a, false, sizeof(a));
a[tmp % m] = true;
for(int j = 0; j < m; j++)
{
if(b[j])
{
a[tmp % m] = true;
a[(tmp + j) % m] = true;
}
}
for(int j = 0; j < m; j++)
{
if(a[j])
b[j] = a[j];
if(b[0])
{
flag = true;
break;
}
}
if(flag)
break;
}
printf("%s\n", flag ? "YES" : "NO");
}
Petya can ask questions like: "Is the unknown number divisible by number y?".
The game is played by the following rules: first Petya asks all the questions that interest him (also, he can ask no questions), and then Vasya responds to each question with a 'yes' or a 'no'. After receiving all the answers Petya should determine the number that Vasya thought of.
Unfortunately, Petya is not familiar with the number theory. Help him find the minimum number of questions he should ask to make a guaranteed guess of Vasya's number, and the numbers yi, he should ask the questions about.
A single line contains number n (1 ≤ n ≤ 103).
Print the length of the sequence of questions k (0 ≤ k ≤ n), followed by k numbers — the questions yi (1 ≤ yi ≤ n).
If there are several correct sequences of questions of the minimum length, you are allowed to print any of them.
4
3
2 4 3
6
4
2 4 3 5
The sequence from the answer to the first sample test is actually correct.
If the unknown number is not divisible by one of the sequence numbers, it is equal to 1.
If the unknown number is divisible by 4, it is 4.
If the unknown number is divisible by 3, then the unknown number is 3.
Otherwise, it is equal to 2. Therefore, the sequence of questions allows you to guess the unknown number. It can be shown that there is no correct sequence of questions of length 2 or shorter.
題目大意:兩個人做遊戲,A從1-n中選一個數字x,B通過詢問一些數字對x的整除性判斷x是什麼(即該數字能不能被x整除),現在求B至少問多少次,每次問什麼才能做到不管A從1-n中選的是哪個數字,他都能判斷出來
題目分析:分析可以發現答案就是唯一分解後只有一種素數的數,證明可以通過唯一分解定理,腦補一下就好,注意0的時候只輸出一個0
#include <cstdio>
int ans[1005];
int main()
{
int n, cnt = 0;
scanf("%d", &n);
for(int i = 2; i <= n; i++)
{
bool flag = false;
for(int j = 2; j <= i; j++)
{
int tmp = i;
while(tmp % j == 0)
{
flag = true;
tmp /= j;
}
if(tmp == 1)
ans[cnt ++] = i;
if(flag)
break;
}
}
printf("%d\n", cnt);
for(int i = 0; i < cnt - 1; i++)
printf("%d ", ans[i]);
if(cnt)
printf("%d\n", ans[cnt - 1]);
}

We call a hamiltonian path to be some permutation
pi of numbers from
1 to n. We say that the length of this path is value
.
Find some hamiltonian path with a length of no more than 25 × 108. Note that you do not have to minimize the path length.
The first line contains integer n (1 ≤ n ≤ 106).
The i + 1-th line contains the coordinates of the i-th point: xi and yi (0 ≤ xi, yi ≤ 106).
It is guaranteed that no two points coincide.
Print the permutation of numbers
pi from
1 to n — the sought Hamiltonian path. The permutation must meet the inequality
.
If there are multiple possible answers, print any of them.
It is guaranteed that the answer exists.
5
0 7
8 10
3 4
5 0
9 12
4 3 1 2 5
In the sample test the total distance is:
(|5 - 3| + |0 - 4|) + (|3 - 0| + |4 - 7|) + (|0 - 8| + |7 - 10|) + (|8 - 9| + |10 - 12|) = 2 + 4 + 3 + 3 + 8 + 3 + 1 + 2 = 26
題目大意:求一個排列,使得dist(pi, pi+1)的值小於等於25*10^8
題目分析:考慮把大座標系豎直分成1000份,則豎直方向上最多走2*10^6*10^3 = 2e9,再考慮水平方向,再當前豎直塊中最多移動2*1000次,從當前塊移動到下一塊最多走1000,一塊中的移動就算3*1000,一共10^3塊,因此水平最多走3e6,加起來肯定小於25e8,因此按縱座標排序,給每塊標號,當塊標號為奇數時,從下往上,偶數時從上往下,畫個圖就看出來了
#include <cstdio>
#include <vector>
#include <algorithm>
using namespace std;
vector < pair<int, int> > vt[1005];
int main()
{
int n, x, y;
scanf("%d", &n);
for(int i = 1; i <= n; i++)
{
scanf("%d %d", &x, &y);
vt[x / 1000].push_back(make_pair(y, i));
}
for(int i = 0; i <= 1000; i++)
{
sort(vt[i].begin(), vt[i].end());
if(i & 1)
reverse(vt[i].begin(), vt[i].end());
int sz = vt[i].size();
for(int j = 0; j < sz; j++)
printf("%d ", vt[i][j].second);
}
printf("\n");
}
相關文章
- Codeforces Round 976 (Div. 2) 題解
- Codeforces Round 983 (Div. 2) 題解
- Codeforces Round #956 (Div. 2)題解
- Codeforces Round 951 (Div. 2) 題解
- Codeforces Round 965 (Div. 2) 題解
- Codeforces Round #681 (Div. 2)E題解
- Codeforces Round 932 (Div. 2) DE 題解
- Educational Codeforces Round 93 (Rated for Div. 2)題解
- Codeforces Round #665 (Div. 2)A-C題解
- Codeforces Round #673 (Div. 2)(A-D)題解
- Codeforces Round #439 (Div. 2) A-C題解
- Codeforces Round #Pi (Div. 2) (ABCD題解)
- Codeforces Round 976 (Div. 2) 題解(A-E)
- Codeforces Round 893 (Div. 2)題解記錄
- Codeforces Round 892 (Div. 2)題解記錄
- Educational Codeforces Round 171 (Rated for Div. 2) 題解
- Codeforces Round #537 (Div. 2)解題報告
- Codeforces Round #186 (Div. 2) (ABCDE題解)
- Codeforces Round #105 (Div. 2) (ABCDE題解)
- Codeforces Round #200 (Div. 2) (ABCDE題解)
- Codeforces Round #313 (Div. 2) (ABCDE題解)
- Codeforces Round #266 (Div. 2)解題報告
- Codeforces Round #323 (Div. 2) (ABCD題解)
- Codeforces Round #331 (Div. 2) (ABC題解)
- Codeforces Round 899 (Div. 2)題解記錄
- Codeforces Round 979 (Div. 2) (ABCD個人題解)
- Educational Codeforces Round 168 (Rated for Div. 2) 題解
- Codeforces Round 986 (Div. 2)題解記錄(A~D)
- Codeforces Round 987 (Div. 2)題解記錄(A~D)
- Codeforces Round 984 (Div. 3) 題解
- Codeforces Round 962 (Div. 3) 題解
- Codeforces Round 946 (Div. 3) 題解
- Codeforces Round #646 (Div. 2)【C. Game On Leaves 題解】GAM
- Codeforces Round #315 (Div. 2) (ABCD題解)
- Codeforces Round 975 (Div. 2)題解記錄(A,B,E)
- Codeforces Round #639 (Div. 2)
- Codeforces Round #541 (Div. 2)
- Codeforces Round #682 (Div. 2)