模板類
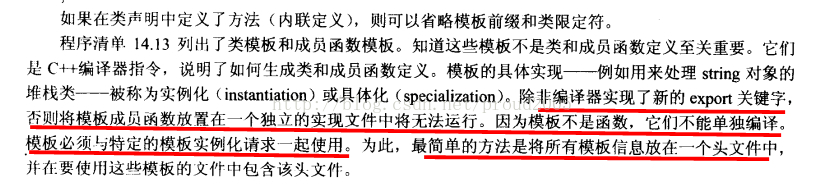
/*
* MyStack.h
*
* Created on: 2014-3-24
* Author: Administrator
*/
#ifndef MYSTACK_H_
#define MYSTACK_H_
#include "iostream"
template<class Item>
class MyStack {
public:
MyStack();
void add(const Item&);
void show();
virtual ~MyStack();
private:
Item item[100];
int top;
};
template<class Item> //模板方法
MyStack<Item>::MyStack() {
top = 0;
}
template<class Item>
MyStack<Item>::~MyStack() {
top = 0;
}
template<class Item>
void MyStack<Item>::add(const Item& ni) {
item[top++] = ni;
}
template<class Item>
void MyStack<Item>::show() {
using namespace std;
for (int var = 0; var < top; ++var) {
cout << "var:" << var<<",value:" << item[var];
}
cout << endl;
}
#endif /* MYSTACK_H_ */
陣列大小動態生成
/*
* MyStack.h
*
* Created on: 2014-3-24
* Author: Administrator
*/
#ifndef MYSTACK2_H_
#define MYSTACK2_H_
#include "iostream"
template<class Item>
class MyStack2 {
public:
MyStack2(int _size);
void add(const Item&);
void show();
virtual ~MyStack2();
private:
Item *item;
int top;
int size;
};
template<class Item>
MyStack2<Item>::MyStack2(int _size) :
size(_size) {
top = -1;
item = new Item[size];
}
template<class Item>
MyStack2<Item>::~MyStack2() {
top = -1;
delete[] item;
}
template<class Item>
void MyStack2<Item>::add(const Item& ni) {
if (++top < size) {
item[top] = ni;
}else{
top=size;
std::cout<<"full"<<std::endl;
}
}
template<class Item>
void MyStack2<Item>::show() {
using namespace std;
for (int var = 0; var <top; ++var) {
cout << "var:" << var << ",value:" << item[var]<<endl;
}
cout << endl;
}
#endif /* MYSTACK_H_ */
// MyStack<string> my;
// my.add("Aba");
// my.show();
// MyStack<int> my1;
// my1.add(1);
// my1.show();
// MyStack<const char *> my2;
// my2.add("abc");
// my2.show();
MyStack2<const char *> my2(2);
my2.add("abc");
my2.add("abc");
my2.add("abc");
my2.add("abc");
my2.add("abc");
my2.show();
表示式引數模板
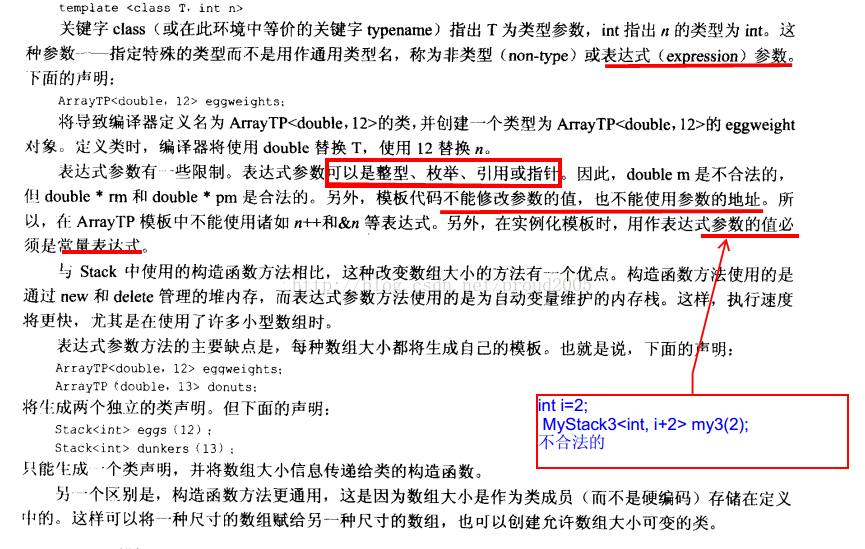
/*
* MyStack3.h
*
* Created on: 2014-3-24
* Author: Administrator
*/
#ifndef MYSTACK3_H_
#define MYSTACK3_H_
#include <iostream>
template<class TP, const int n>
class MyStack3 {
public:
explicit MyStack3(const TP initValue);
TP operator[](const int index);
~MyStack3();
private:
TP items[n];
};
template<class TP, const int n>
MyStack3<TP, n>::MyStack3(const TP initValue) {
for (int var = 0; var < n; var++) {
items[var] = initValue;
}
}
template<class TP, const int n>
TP MyStack3<TP, n>::operator[](const int index) {
return items[index];
}
template<class TP, const int n>
MyStack3<TP, n>::~MyStack3() {
}
#endif /* MYSTACK3_H_ */