狀態模式
狀態模式的類圖
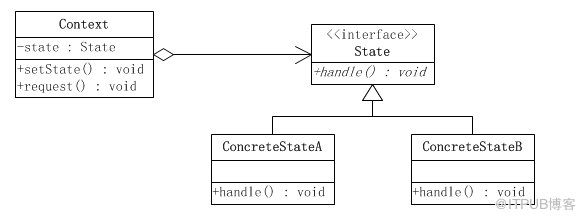
我覺得在現實生活中,比較典型的狀態模式就是紅綠燈。
與責任鏈模式不同的是,狀態模式在狀態執行時,指定下一個狀態的資訊。
而責任鏈模式則在Client裝配責任鏈。
相對來說,狀態模式的狀態轉換是固定的,而責任鏈模式的請求路徑,則可以在Client呼叫時,動態構建。
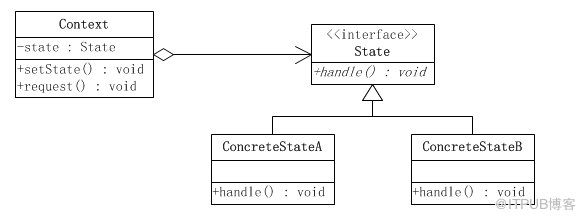
我覺得在現實生活中,比較典型的狀態模式就是紅綠燈。
-
public class Test {
-
public static void main(String[] args) throws InterruptedException {
-
紅綠燈 context = new 紅綠燈();
-
context.beginWork();
-
}
-
}
-
-
class 紅綠燈 {
-
private 指示燈 currentState = new 紅燈();
-
-
public void beginWork() throws InterruptedException {
-
currentState.亮(this);
-
}
-
-
public void setCurrentState(指示燈 currentState) {
-
this.currentState = currentState;
-
}
-
-
}
-
-
interface 指示燈 {
-
void 亮(紅綠燈 context) throws InterruptedException;
-
}
-
-
class 紅燈 implements 指示燈 {
-
-
@Override
-
public void 亮(紅綠燈 context) throws InterruptedException {
-
System.out.println("紅燈點亮");
-
Thread.sleep(3000);
-
context.setCurrentState(new 綠燈());
-
context.beginWork();
-
}
-
-
}
-
-
class 黃燈 implements 指示燈 {
-
-
@Override
-
public void 亮(紅綠燈 context) throws InterruptedException {
-
System.out.println("黃燈閃爍");
-
Thread.sleep(1000);
-
System.out.println("黃燈閃爍");
-
Thread.sleep(1000);
-
System.out.println("黃燈閃爍");
-
Thread.sleep(1000);
-
context.setCurrentState(new 紅燈());
-
context.beginWork();
-
}
-
-
}
-
-
class 綠燈 implements 指示燈 {
-
-
@Override
-
public void 亮(紅綠燈 context) throws InterruptedException {
-
System.out.println("綠燈點亮");
-
Thread.sleep(4000);
-
context.setCurrentState(new 黃燈());
-
context.beginWork();
-
}
-
- }
結果:
紅燈點亮
綠燈點亮
黃燈閃爍
黃燈閃爍
黃燈閃爍
紅燈點亮
紅燈點亮
綠燈點亮
黃燈閃爍
黃燈閃爍
黃燈閃爍
紅燈點亮
...
...
而責任鏈模式則在Client裝配責任鏈。
相對來說,狀態模式的狀態轉換是固定的,而責任鏈模式的請求路徑,則可以在Client呼叫時,動態構建。
來自 “ ITPUB部落格 ” ,連結:http://blog.itpub.net/29254281/viewspace-1127079/,如需轉載,請註明出處,否則將追究法律責任。
相關文章
- JavaStatePattern(狀態模式)JavaAST模式
- JS 狀態模式JS模式
- (三)狀態模式模式
- 狀態模式(State)模式
- 設計模式-狀態模式設計模式
- 設計模式:狀態模式設計模式
- 行為型模式:狀態模式模式
- 設計模式(十五)狀態模式設計模式
- 設計模式之——狀態模式設計模式
- javascript設計模式狀態模式JavaScript設計模式
- 設計模式(六):狀態模式設計模式
- 狀態變化模式模式
- 狀態模式(State pattern)模式
- 17_狀態模式模式
- 用設計模式去掉沒必要的狀態變數 —— 狀態模式設計模式變數
- PHP 設計模式之狀態模式PHP設計模式
- 簡說設計模式——狀態模式設計模式
- 設計模式之狀態模式(State)設計模式
- 設計模式-狀態模式(State Pattern)設計模式
- python設計模式狀態模式Python設計模式
- 設計模式20之狀態模式設計模式
- 極簡設計模式-狀態模式設計模式
- GoLang設計模式14 - 狀態模式Golang設計模式
- Python設計模式-狀態模式Python設計模式
- Java設計模式之狀態模式Java設計模式
- 設計模式——20狀態模式(State)設計模式
- 設計模式系列之「狀態模式」設計模式
- 轉載---Dephi狀態模式(State模式)模式
- 設計模式第八講-狀態模式設計模式
- 設計模式漫談之狀態模式設計模式
- python設計模式【9】-狀態模式Python設計模式
- 《Head First 設計模式》:狀態模式設計模式
- C#設計模式之狀態模式C#設計模式
- 設計模式系列9--狀態模式設計模式
- 設計模式系列之十二狀態模式設計模式
- 設計模式之狀態模式---State Pattern設計模式
- 我學設計模式 之 狀態模式設計模式
- 設計模式--直譯器模式和狀態模式設計模式